JavaScript setAttribute()
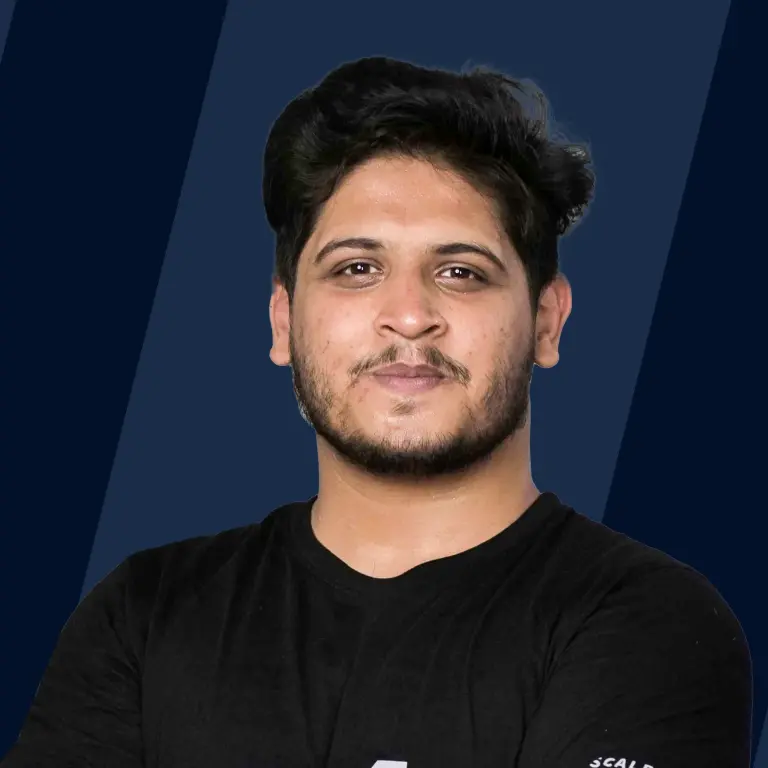
The JavaScript function setAttribute() assigns values to HTML element attributes, including adding new ones. If the attribute doesn't exist, setAttribute() creates it. The function doesn't return any data. Attribute names become lowercase when applied to elements. Using setAttribute() sets attribute values on chosen HTML elements; existing ones are modified, and new attributes are added. However, it overwrites other CSS properties, so avoid using it for inline styles. To use setAttribute():
- Select the HTML element.
- Use methods like getElementById(<id>), getElementsByTagName(<tag_name>), etc.
- Apply setAttribute(attributeName, attributeValue) to add attributes.
In this article, we will learn about setAttribute() function in JavaScript.
Syntax of setAttribute() in JavaScript
setAttribute in JavaScript requires all of its parameters when called. None of the parameters are optional. The parameters significance for this function is as follows:
Parameters of setAttribute in JavaScript
Let's look at the parameters of the javascript set attribute.
-
attributeName: The attribute's name is what attribute we wish to add to the HTML element. It is not an optional argument and cannot be left unfilled. For example, href, type, style, etc.
-
attributeValue: The attribute's value is what value we wish to add to the attribute name.
Additionally, it is also a mandatory argument. For example, https://www.google.com for href attribute, text for type, etc.
Return Value of JavaScript setAttribute()
The JavaScript setAttribute() function returns undefined.
Exceptions of JavaScript setAttribute()
The following code values may cause this function to raise a DOMException:
- INVALID_CHARACTER_ERR: This means that a character not permitted in attribute names for HTML or XML files is present in the name argument.
- NO_MODIFICATION_ALLOWED_ERR: This means that there is no way to change the attributes of an HTML element, they are read-only.
Also, the setAttribute() function can be used to add the style attribute, but it is not advised to do so for styling, since it may cause existing style attributes to be overwritten.
Incorrect Method:
It is advised to avoid using the following syntax to alter the style of an HTML element.
Correct Method:
Following is the syntax of how to modify the style property of an HTML element using JavaScript.
Example of JavaScript setAttribute() Function
The below JavaScript program demonstrates how to set an attribute for an HTML element using setAttribute in JavaScript:
JavaScript Code:
Output:
Explanation:
- In the above example, we have added an attribute href = "https://scaler.com/topics" to <a> element having id = "set".
- We have added an onclick = "demo()" attribute to the Set Attribute button, so when we click on the Set Attribute button, the demo() function invokes.
- In the demo() function, document.getElementById("set") is used to select the <a> HTML element, and setAttribute("href", "https://scaler.com/topics"); is used to set the href attribute value to https://scaler.com/topics link. Similarly, we have set the style attribute to color: red;.
More Examples
1. Changing the color of a button on click using setAttribute in JavaScript
Output:
Explanation:
- In the above example, we have added the style attribute with background-color: "red"; value to the button HTML element having id = "set" using the setAttribute() function.
- We have an onclick = "changeColor()" attribute with the Click to change the color button, so when we click on the Click to change the color button, the changeColor() function invokes.
2. Setting the type of input field to the button type using setAttribute in JavaScript.
Output:
Explanation:
- In the above example, we have added the type and value attributes with button and Now it's a button value respectively to the input HTML element having id = "set" using the setAttribute in JavaScript.
- We have an onclick = "convert()" attribute with the Click to convert... button, so when we click on the Click to convert... button, the convert() function invokes.
3. Disabling a button using setAttribute in JavaScript
Output:
Explanation:
- In the above example, we have added the disable attribute to the button HTML element having id = "set" using the setAttribute() function.
- We have an onclick = "disable()" attribute with the Click to disable... button, so when we click on the Click to disable... button, the disable() function invokes.
Supported Browser
Desktop/Laptop (Large Screen)
Browsers | Versions Supported |
---|---|
Google Chrome | v4.0-v102.0, v103.0, v104.0-v106.0 |
Microsoft Edge | v12.0-v102.0, v103.0 |
Mozilla Firefox | v2.0-v103.0 |
Opera | v10.6-v87.0 |
Safari | v3.1-v15.5, v16.0-STP |
Internet Explorer | v6.0-v11.0 |
Mobile/Tablet (Small Screen)
Browsers | Versions Supported |
---|---|
Chrome Android | v102.0 |
Firefox for Android | v101.0 |
Opera Android | v12.0, v12.1, v64.0 |
Safari on iOS | v3.2-v15.5, v16.0 |
Samsung Internet | v4.0-v17.0 |
WebView Android | v4.4-v4.4.4 |
Conclusion
- setAttribute in JavaScript is mostly used to assign a value to the HTML attributes.
- We can also add a new attribute with a value to a certain HTML element using the setAttribute() function.
- The setAttribute() function returns undefined / NONE.
- We can get, set, remove, or check if an attribute is present or not in the HTML element using the DOM methods.