Shift() in JavaScript
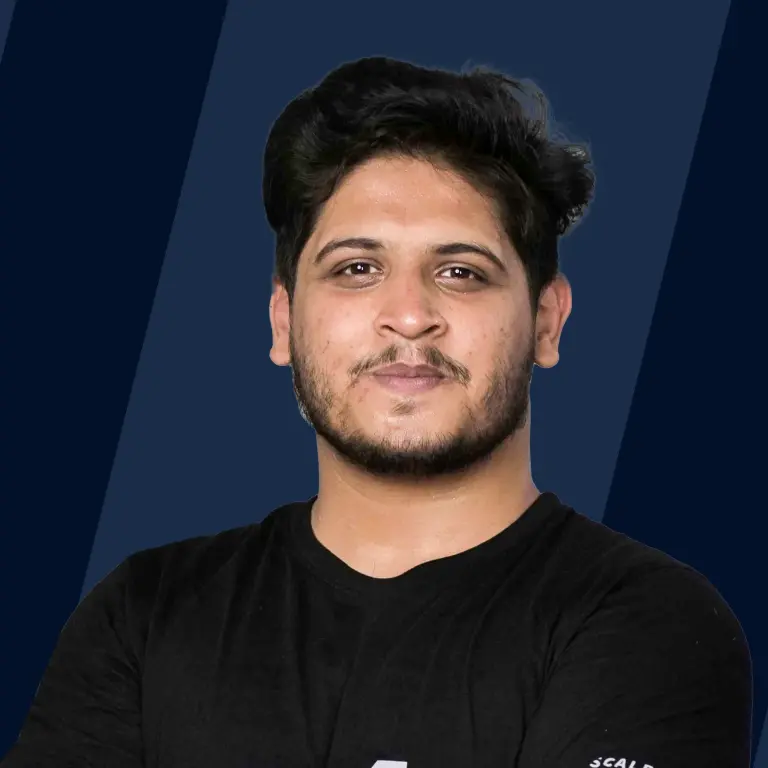
Overview
shift is an inbuilt Array method in Javascript that removes the first element from an array and returns the removed element. This method modifies the length of the original array.
Syntax Of Array shift() In Javascript
The syntax of array shift in javascript is very simple. You have to use it on the array from which you want to remove an element.
The following shows the syntax of the shift() method :
Where array refers to the name of a variable that contains the array of elements, you need to add shift() with a dot to the end of the variable from which you want to remove the element.
Parameters Of Array shift() In Javascript
To work with shift(), you don't have to add parameters because this method does not accept any parameters.
Return Value Of Array shift() In Javascript
There are two cases sometimes it returns elements or is sometimes undefined.
- If the array is not empty, it removes the first element and returns the removed element.
- If the array is empty, it returns the undefined.
Example
Let's look at an example to understand shift() in JavaScript.
Output
What Is Array shift() In Javascript?
shift() method removes the first element from an Array and returns the removed element.
Using shift, the element on the 0th index is removed, and the other items are moved forward.
Just imagine if you have an array of numbers from 1 to 4 (Eg. arr = ) and you want to remove the first element. You have to use the shift method on the array to do so. Shift removes the first element from the array and returns the removed element.
Furthermore, the shift method reduces the size of the array by 1.
Important points to remember :
- After deleting the 0th index or the first element, it shifts the other values to the consecutive index as it updates the entire array because of deleting a single value. It affects the performance and slows down the process.
- This method changes the original array and the length of the array.
- If the array is empty, then the shift function returns undefined.
The issue with shift() operation :
Hope you understand how shift() works but now come to the point when it starts giving some issues.
The main problem is performance because we know that shift removes the first element and moves forward all the elements in the array.
So when we remove one element, it changes the index of all other elements in the array, and that's where unnecessary operations start performing by the code, and it slows down the process.
Let's understand this with an illustration:
The shift() method do 3 things :
- First, it removes the element from the 0th index.
- it moves forward all the elements and renumbered their index.
- And last, it updates the length property of the array.
Because of all these operations, it depends on the size of the array. The more elements in the array, The more time it takes to perform all operations.
More Examples
Example 1 : Removing an element from an array
To remove an element from an array, you have to call the shift method on the array.
Check the below code:
Output:
How do all of these work?
First, we are defining an array with the value from 1 to 6 with the name of num.
Second, we are removing the first element from the num array and assigning the removed element to the returnValue variable that contains the return value.
Third, we are outputting the return value on the console with the help of the console function.
In the last, we are printing the num array to cross-check the removed element, and you can see the output that the first element is removed and the length of the array gets updated.
Always remember when you use the shift method it updates the original array and its length.
Example 2 : Using shift() method in while loop
We can use the shift method by combining it with the while loop to remove more than one element.
The while loop executes the block of code as long as a specified condition is true.
Output :
Let's understand it line by line.
First, we have an array with the variable name of num that contains the value from 1 to 6.
We are assigning another variable with the name of the number without giving the value to it because we will use it in the while loop to store the return value of the shift method.
And these two lines are the most crucial logic of your code to use the while loop with the shift method to remove all the elements from the array.
First, we are using while loop syntax.
The condition of our code is that we are calling the shift method on the number array and using the number variable to assign the return value of the shift.
And we are checking while condition if the return value is undefined then stop the execution of code block, or return value is something else then continue the execution.
This loop continues till the condition becomes false.
The second last part of the code is to print a message that says Done so we know that execution is stopped and all the elements are removed from the array.
At last, we are printing the array on the console to prove that all the elements are removed from it.
Browser compatibility
The shift() method in javascript is supported by all the browsers such as Chrome, Edge, Safari, Opera, etc.
Conclusion
- The shift() method removes the first element from an array and returns the removed element.
- The shift() method changes the original array and its length (as the first element is removed from the array).
- The shift() returns undefined if the array is empty.