Socket Programming in Python
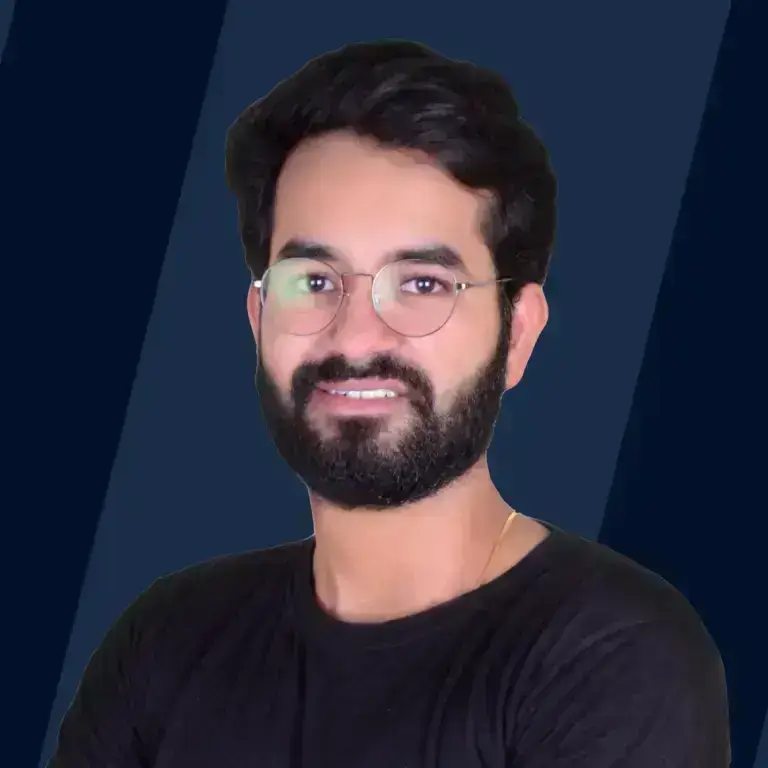
Socket programming in python provides inter-process communication between the nodes (client and socket) in the network (the network could be a logical, local network to the computer or one that’s physically connected to an external network). Well, a server is software that forms the listener socket, which means it waits for the client's requests and processes them accordingly.
Where a client is a requester of this service, it reaches out to the server to form a connection. So, the client program requests some resources from the server, which in turn, sends a response to that inquiry.
Connecting to Python Socket Server
Now, we will create a socket server program and save it using any name(in our case, it’s ). So, to make a Python socket server, we have to import the socket module, then we will perform the useful tasks to build the connection between client and server. Let’s see step-by-step what we are going to do.
The server utilizes the bind() function to establish a connection using a specific IP and Port, enabling it to receive incoming requests through those parameters. When the bind function is executed with an empty string in place of the IP, it allows the server to accept requests from various computers within the network. Furthermore, the server employs the listen() function, transitioning it into a mode that enables it to await incoming connections. Conclusively, the server features an accept() function, which facilitates the initiation of a client connection, and a close() function, responsible for terminating the client connection.
Making a Server
- Import the module.
- (Optional) Utilizing the socket.gethostname() method is a discretionary step. By either employing this or opting for an empty string, your server is capable of accepting connections from external computers.
- Allocate a port number on your machine, ensuring it exceeds 1024 since ports below this threshold are designated for well-known internet services. In this scenario, the selected port is 12121.
- Create a socket object instance through the socket.socket() method, which could alternatively be performed at an earlier stage.
- Proceed to associate the IP/host and port number using the bind() method.
- Invoke the listen() method to enable the server to accept connections, with the option to set a limit on the number of concurrent client connections.
- Initiate a client connection with the accept() method, communicate via the send() method, and conclude the session using the close() method to sever the connection.
Now, let's jump into the code:
Explaination:
As illustrated in the above example, the initial step involves importing the socket library, which is essential for socket programming in python. Following that, a socket object is created and linked with the hostname and port number, effectively associating our machine with its local hostname.
This setup ensures that the system responds exclusively to requests originating from this specific hostname, or when the IP address 127.0.0.1 is utilized, it restricts communication to only local machines.
Subsequently, we activate the server's listening state. You may wonder about the significance of the number 2 passed to the listen() method. This figure represents the maximum number of pending connections that the operating system can queue for this socket. In this scenario, the socket can queue up to two connections, beyond which the system's kernel will start to decline any additional incoming requests for the socket.
Connecting to Python Socket Client
Now, we will create something with which the server can interact, i.e., a client. We will make a program and save it using any name(in our case, it’s ). This program is very similar to the one mentioned above (i.e., ). The only difference is that in the server program we use the bind() method to bind host and port, but in the client program we use the connect() method to connect host and port so that it can connect to the server easily and communicate with it.
Making a Client
- Import a socket module.
- (Optional) Get the hostname using the socket.gethostname() function as both server and client programs are running on your local PC.
- Using the socket.socket() function, you can create an instance socket object.
- Then, connect to the local host by passing the port number and hostname of the server. In our case, the port number is 12121.
- Finally, send and receive messages from the server using the send() and recv() functions, and close the connection with the server.
Now, let's jump into the code
As you can see in the code above, Initially, we generate a socket object, establish a connection to localhost (port 12121), proceed to gather data from the server, dispatch data back to the server, and finally terminate the connection.
Note: Run the above client script after starting the server script.
Python Socket Programming Output
We have made a server and a client. Now, let's see the output of the above codes. So first of all, we will start the server program and then the client program.
As you can see in the above output, after starting both client and server, they both get connected and communicate with each other, and finally they get disconnected.
Example of Socket Programming in Python
There are many examples that exist that use socket programming in python. Since everything involves communication, that means they are using sockets. Like your web browser, the Internet, chat bots, etc.
A chat bot is the most basic example that uses networking. It is something like WhatsApp, but this is only for two users.
Discussing online banking services, whenever a customer engages with the bank's service using a web browser, this action triggers a request to the bank's web server.
The banking system might save the customer's sign-in details in its database, with the web server accessing this database in a client capacity. An application server then processes the retrieved data by enforcing the bank's operational rules and relays the processed information back to the web server. In the final step, the web server delivers the outcome to the client's web browser for visualization.
Conclusion
- Socket programming in python provides inter-process communication between the nodes (client and socket) in the network (the network could be a logical, local network to the computer or one that’s physically connected to an external network).
- The client program requests some resources from the server, which in turn, responds to that request.
- A socket is a network endpoint for communication between servers and clients.
- The server has bind(), listen(), accept(), close(), and other methods that help with client connections.
- The best example of the socket programming in python is the web browsers such as Google chrome, Mircosoft edge, etc.