Sort a String in Java
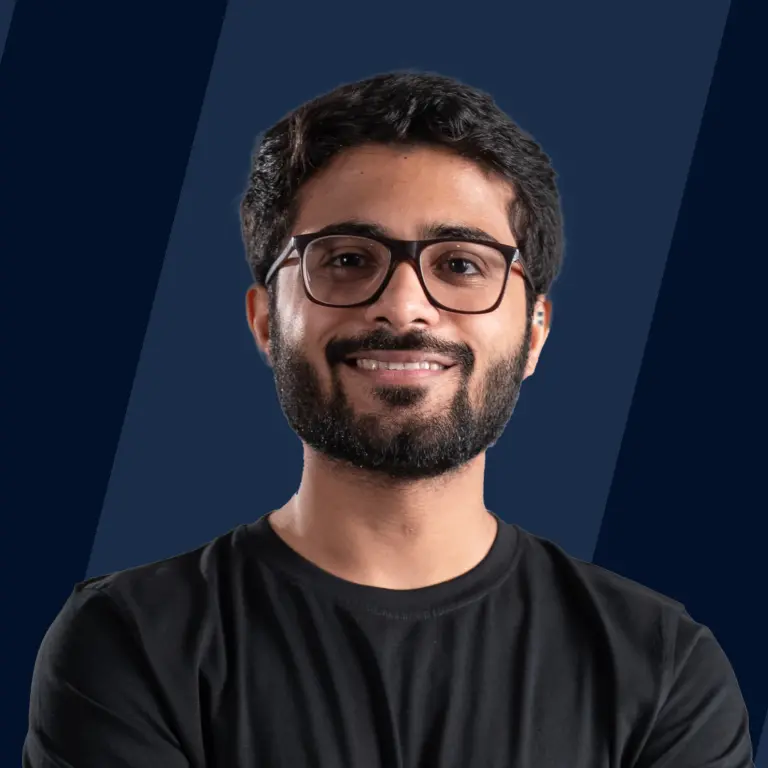
Overview
Strings are nothing but a sequence of characters. In certain situations, we need to reorder this sequence in such a way that its characters are arranged in lexicographically increasing or decreasing order. This is where the concept of sorting a string comes in.
Java has several ways to rearrange the characters inside the string in a particular order, many of them require it to be converted into an array of characters, like the Arrays.sort() and sorted() methods. Java has inbuilt methods to sort a string in any order be it increasing or decreasing.
Introduction
A string is a collection of characters that can include letters, numbers, symbols, and even spaces. To be recognized as a string in Java, it must be surrounded by double-quotes. For example, "Scaler", "how to sort a string in java?" and "123Random" are strings.
Strings in Java can be created using two ways.
- String literal
- Using new keyword
To get the desired output from our program, we frequently need to arrange the individual characters in strings in different orders. Sorting is the process of arranging a sequence (string in this case) based on desired criteria.
In other words, sorting a string is the procedure to arrange its characters in increasing or decreasing order. Java provides methods like Arrays.sort(), reverseOrder() and sorted() to sort a string, which are covered in the subsequent sections.
Method 1: Arrays.sort() Method
In Java, the Arrays.sort() method is used to sort any type of array, whether it is an integer or a character array. To sort a String using this method:
- Convert the input string into a char array using the toCharArray() method.
- Sort the char array using Arrays.sort() method.
- Finally convert the sorted array back to a String.
This Arrays.sort() method has the time complexity of where N is the size of the array.
Example:
Output:
Custom sorting using Arrays.sort() method
Custom sorting allows us to modify the Arrays.sort() method's sorting criteria since we know that sorting is done based on ASCII values of characters therefore we can define a custom logic to compare two characters and decide which one should come first.
The demonstration for the same is shown below, where we will be sorting the string "scaler" in reverse order.
- Convert the input string into a Character array by creating a Character array of length equal to the size of the string. Populate this array with the characters of the string using a for loop.
- Pass one more parameter to the Arrays.sort() method that is the Comparator and override the default comparison criteria based on ASCII values.
- Syntax of the compare() function to be used as Comparator:
- The compare() function returns a negative value if o1 has to come first, positive if o2 has to come first, and 0 if they are the same.
Output:
Method 2: Using Java 8 Streams and sorted() Method
Java 8 provides us with the Stream, which can sort any kind of list using its built-in sorted() method. So we will need to convert the string into a list of characters using the chars() method first then sort it using the sorted() method.
Example:
Output
Explanation:
- The example above sorts the String "Scaler" in alphabetically increasing order.
- The inputstr is converted into the list of chars and then, this list is arranged in increasing alphabetical order using the sorted() method.
- The collect() method takes the sorted object and passes it to appendCodepoint() method of [StringBuilder](https://www.scaler.com/topics/java/stringbuilder-in-java/), which appends the string representation of each codepoint object to the sequence.
- Finally toString() method is used to convert it into a string.
Method 3: reverseOrder() Method
The reverseOrder() method of the Java Collections class is used to sort an array in lexicographically decreasing order. Because it is a static method, we can use the class name to call it directly.
- Convert the input string into a Character array similar to what we have done above.
- Pass a second argument to Arrays.sort() method as Collections.reverseOrder() which reverses the ordering of characters after sorting.
- The array is first sorted in ascending order using the Arrays.sort() method, and then the natural ordering is reversed using the reverseOrder() method, resulting in a sorted array in descending order.
The syntax for reverseOrder():
Example:
Output
Explanation:
- We have used the input string "scaler" string to demonstrate reverseOrder() method.
- First, we have converted the string into a Character array and then use the Arrays.sort() method passing reverseOrder() as a comparator to rearrange it.
- Convert it back to a String by applying toString() method on StringBuilder object sb.
Time Complexity: where is the number of characters in the input string.
Conclusion
- Strings are a sequence of characters which can be rearranged in a particular order such as lexicographically increasing or decreasing order.
- Strings in Java can be sorted using various methods such as:
- Arrays.sort()
- sorted() method (Java 8 Streams)
- We can define custom comparators to arrange the characters of a string. These Comparators can be defined using the compare() function.
- Collections.reverseOrder() method can be used to reverse sort a string. It is passed as second argument inside Arrays.sort() method.