How to Sort ArrayList in Java?
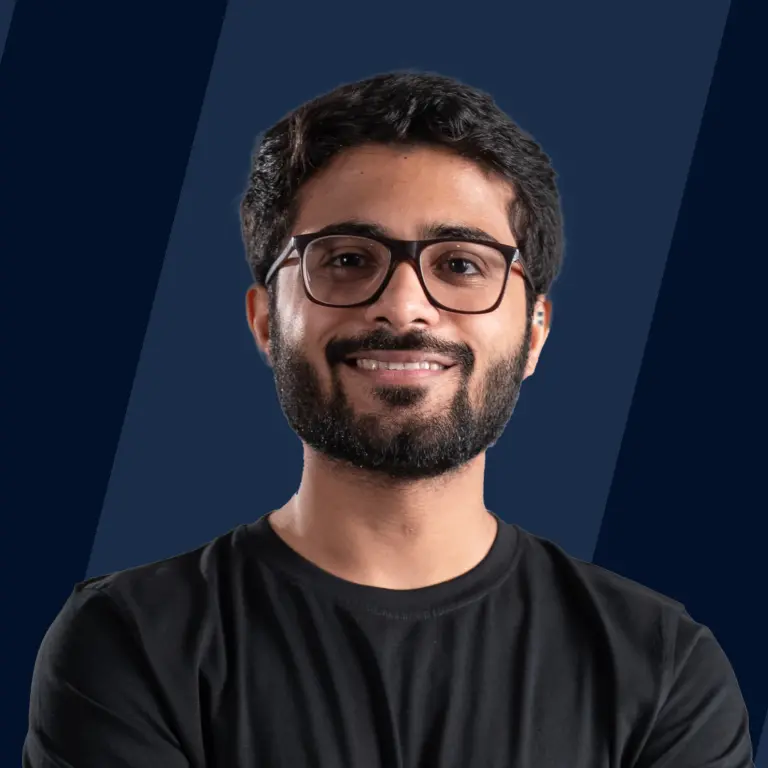
Overview
In this article, we are going to learn about how to sort ArrayList in java. Before getting started with the topic of sorting ArrayList in java, let us get a short overview of what is an ArrayList in java.
Arraylist in java :An ArrayList** in java is a Collections framework class which exists inside the java.util package. It usually inherits the properties of the AbstractList class. An ArrayList is a dynamic data structure that stores elements in it dynamically, that is it does not have any size limits. So we can add as many elements as we want inside an ArrayList, but it should not exceed the memory limit the JVM has available.
Note: An ArrayList in java can store the elements in an unsorted manner, not in ascending or descending order but just index-wise. So here, in this article, we are going to learn how we can sort an ArrayList in java, to arrange the elements in an ArrayList in ascending or descending order.
How to Sort ArrayList in Java?
In Java, there is a collection framework that provides interfaces like List, Set, Stack, Queue, etc., and also classes like LinkedList, ArrayList, etc. in which multiple elements or objects can be stored. Usually, the elements are stored in an unsorted manner. To arrange the data in a sorted manner we use different methods. An ArrayList can be sorted in two ways ascending or descending order. Mainly the collections class provides two methods to sort an ArrayList in Java in ascending or descending order.
- sort()
- reverseOrder()
Let's discuss these sorting methods in detail.
Collections.sort() Method
Using the sort() method of the collections class we can sort ArrayList in ascending order in java. Basically, it takes the elements of ArrayList as parameters and sorts them in ascending order, and returns an ArrayList that is sorted in ascending order, with respect to the original ordering of its elements.
Now let us see the syntax of the Collections.sort() Method in java.
Syntax of ArrayList Sort()
Below given is the syntax for the Collections.sort() Method in java to sort an ArrayList in ascending order.
Explanation : Here in the above syntax, we are using the sort() method from the collection class to sort the ArrayList in ascending order. Basically the sort() method takes two parameters inside it, one is the object of ArrayList and another is the comparator (Collections.reverseOrder()) using which we can sort the ArrayList in descending order, but for sorting the ArrayList in ascending order you don't need the second argument (Collections.reverseOrder()), and just by using the object name of ArrayList we can sort it in ascending order.
Now let us see the syntax for the Collections.sort() Method in java to sort an ArrayList in descending order.
Explanation : Here in the above syntax, we are using the sort() method from the collection class to sort the ArrayList in descending order. Basically the sort() method takes two parameters inside it, one is the object of ArrayList and another is the comparator (Collections.reverseOrder()) using which we can sort the ArrayList in descending order. We will discuss comparator in detail below.
There is an alternative way of using the sort() method to sort an array in ascending or descending order. Let us check the syntax of it and discuss it.
Explanation : Here in the above syntax we are using the sort method. Here the ArrayList is the object of the ArrayList class. It has a single parameter Comparator which will specify the sorting order of the ArrayList which is whether we want to sort the ArrayList in ascending order or descending order.
So in the above syntax, we have seen that how we can use the sort() method in different ways to sort an ArrayList in Java.
Sort() Parameters
Basically, the sort() method of the Collection class takes two parameters that are the object of ArrayList to be sorted, and a Comparator. However, the Comparator is optional if we do not pass it then it will sort the ArrayList in ascending order.
The ArrayList sort() method will take a single parameter comparator.
Comparator : It specifies the sorting order of the ArrayList, that is with this we can sort the ArrayList in ascending order and descending order.
Sort() Return Values
The sort() method does not return any value. It will just order the elements in ascending or descending order according to the natural ordering of its elements.
Sort ArrayList in Ascending Order
Let us now briefly understand how we can sort an ArrayList in ascending order with the help of an example.
Code :
Output :
Explanation :
In the above code, we have created an ArrayList of type Integer and added some elements to it. After that, we used the sort() method of the Collections class and passed the object of the ArrayList class to sort the ArrayList in ascending order, and we printed the elements of the ArrayList before and after sorting the ArrayList in ascending order.
Let us take another example of sorting an ArrayList in ascending order.
Code :
Output :
Explanation :
In the above code, we have created an ArrayList of the type String and added some elements to it. This time we have used the sort() method of the Collections class in a different way, with the object of ArrayList calling the sort() method with the dot (.) notation. Inside the method, we have passed the Comparator.naturalOrder() which will sort the ArrayList in lexicographical ascending order, basically, if we are sorting the ArrayList in dictionary manner we termed it as a lexicographically sorting. We have also printed the elements of the ArrayList before and after sorting the ArrayList in ascending order.
Sort ArrayList in Descending Order
Let us now briefly understand how we can use the collections.reverseorder and Comparator.reverseorder to sort an ArrayList in descending order with the help of an example.
Code :
Output :
Explanation :
In the above code, we have created an ArrayList of type Integer and added some elements to it. After that, we used the reverseOrder() method along with the sort() method of the Collections class and passed the object of the ArrayList class to sort the ArrayList in descending order, and we printed the elements of the ArrayList before and after sorting the ArrayList in descending order.
Let us take another example of sorting an ArrayList in descending order.
Code :
Output :
Explanation :
In the above code, we have created an ArrayList of the type String and added some elements to it. This time we have used the sort() method of the Collections class in a different way, with the object of ArrayList calling the sort() method with the dot (.) notation. Inside the method, we have passed the Comparator.reverseOrder() which will sort the ArrayList in descending order, and we have printed the elements of the ArrayList before and after sorting the ArrayList in descending order.
Sort the ArrayList in Reverse Order
Basically sorting an ArrayList in reverse order is similar to sorting an ArrayList in descending order. Let us now briefly understand how we can sort an ArrayList in reverse order with the help of an example.
Code :
Output :
Explanation :
In the above code, we have created an ArrayList of type Integer and added some elements to it. This time we have used the sort() method of the Collections class in a different way, with the object of ArrayList calling the sort() method with the dot (.) notation. Inside the method, we have passed the Comparator.reverseOrder(). Here the reverseOrder() method of the Comparator interface sort the elements of the ArrayList in reverse order that is, in descending order.
Sort the Arraylist using Comparator
Throughout the article, the comparator term is mentioned many times, let us now understand what is comparator.
Comparator : Comparator is an interface that is used for rearranging the elements of an ArrayList in sorted order. The comparator can be also used for sorting a user-defined object in an ArrayList. In java, the comparator is defined inside the java.util package. With the help of a comparator, we can sort an ArrayList on the basis of multiple variables. Without affecting the original User-defined class in any way, we can implement the comparator. We need to override the compare() method provided by the comparator interface to sort an ArrayList using Comparator. Below is the syntax of how we can use the comparator method inside the collections.sort() method after rewriting the compare() method.
Syntax :
Explanation :
In the above syntax, the Collections.sort() method consists of two parameters one is the list which should be sorted in ascending or descending order depending on the comparator, and another is the Comparator class instance which will specify the sorting order of the ArrayList. The above Collections.sort() method does not return any value, it just sort the ArrayList in ascending or descending order.
Let us now look at the example of sorting an ArrayList using Comparator, to make it more clear.
Example Of Sorting An ArrayList Using Comparator
Code :
Output :
Explanation :
In the above code, we have a Students class which consists of multiple students roll, name, and marks. Inside the public class MyClass, we are creating an ArrayList object of the Students class, and we are storing different student features(roll, name, marks) inside the ArrayList. We are sorting the ArrayList with the Collections.sort() method, in which we are taking the ArrayList object as the parameter and also the Comparator interface (MarksComparator). The Comparator interface is defined inside the MarksComparator class. Inside the MarksComparator class we have override the compare() method of the Comparator interface. We have sorted the Students class on the basis of their marks. We can also sort it on the basis of name and roll. Let’s sort the above ArrayList based on their name.
Example of Sorting an ArrayList Based on their Name Using Comparable Interface
Comparable : A comparable is an interface, that is used to compare an object of the same class with an instance of that class. it provides the compareTo method that takes a parameter of the object of that class.
Code :
Output :
Explanation :
In the above code, we have a Students class which consists of multiple students roll, name, and marks. Inside the public class MyClass, we are creating an ArrayList object of the Students class, and we are storing different student's features(roll, name, marks) inside the ArrayList. We are sorting the ArrayList with the Collections.sort() method, in which we are taking only the ArrayList object as the parameter. We have implemented the comparable interface inside the Students class itself. Inside the Students class we have override the compareTo() method of the Comparable interface. We have sorted the Students class on the basis of their names this time. We have compare the String values with the compareTo() function.
Let us now look at the example of sorting an ArrayList using lambda function.
Example of Sorting an ArrayList using lambda function
Code :
Output :
Explanation :
In the above code, we have a Students class which consists of multiple students roll, name, and marks. Inside the public class MyClass, we are creating an ArrayList object of the Students class, and we are storing different student's features(roll, name, marks) inside the ArrayList. We are sorting the ArrayList with the Collections.sort() method, in which we are taking the ArrayList object as the parameter. We have also a lambda function inside the Collections.sort() method where we can directly sort the Students class on the basis of their marks.
A lambda expression is a short block of code that can take one or more than one parameter and returns a value. Lambda expressions are similar to methods, but they do not need a name and they can be implemented right in the body of a method.
In the above code, the lambda function consists of two parameters and compares them and helps in sorting the ArrayList in ascending order.
Conclusion
In this article, we learned about sorting an ArrayList in java. Let us recap the points we discussed throughout the article:
- An ArrayList in java is a Collections framework class which is interpreted inside the java.util package.
- Usually the ArrayList in java stores the elements in an unordered manner. So to arrange the data in a sorted manner we use different methods.
- The two main methods provided by the collections class are sort() and reverseOrder() methods.
- Using the sort() method of the collections class we can sort ArrayList in ascending order in java.
- The sort() method contains a single parameter which is the List that is to be sorted.
- The sort() method does not return any value, it just rearranges the elements of ArrayList in ascending order.
- We have seen some examples of how we can sort an ArrayList in ascending and descending order.
- Using the reverseOrder() method of the collections class we can sort ArrayList in descending order in java.
- The reverseOrder() method is basically taken as a parameter inside the Collections.sort() method. Which will sort the ArrayList in descending order.
- We have seen an example of how we can sort an ArrayList in descending order using the reverseOrder() method along with the sort() method in Java.
- Comparator is an interface that is used for rearranging the elements of an ArrayList in sorted order.
- With the help of Comparator we can sort an ArrayList on the basis of multiple variables.
- We have seen some examples of how we can sort an ArrayList using Comparator, and we have also sorted the ArrayList on the basis of multiple fields. ::