sscanf in C
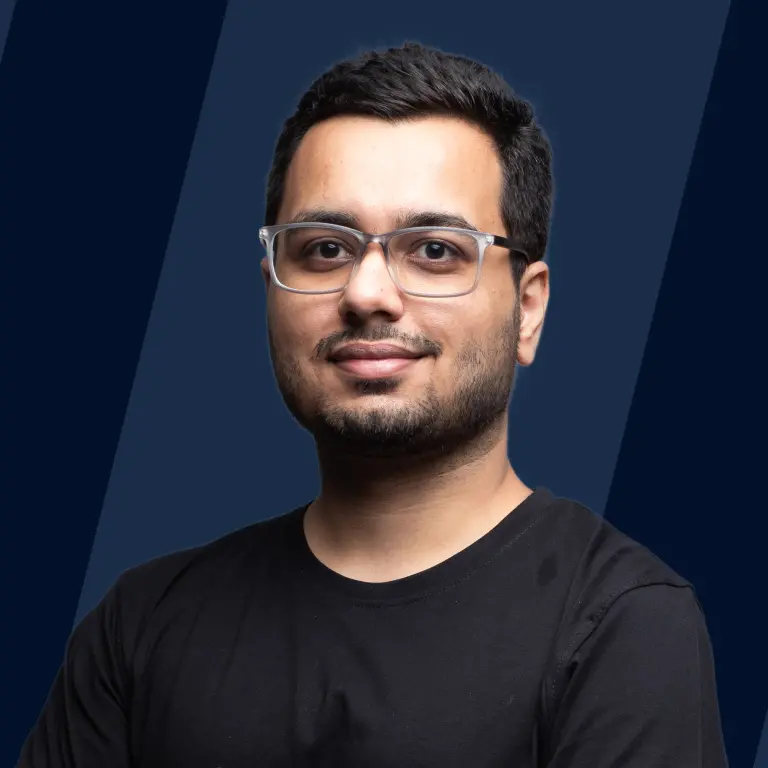
Overview
With the sscanf() function in C, we can read (or extract) data from a string input. The sscanf() function is similar to the scanf() function, the only difference being that scanf() takes input from the console, while sscanf() takes input from a string (or a character array).
Syntax of sscanf() Function in C
Parameters of sscanf() Function in C
The sscanf() function requires two mandatory arguments and optional additional arguments.
- char* string_name :
Pointer to the string that needs to be read. - char* format_string :
It is a string containing a unique sequence of characters that specify how characters will be extracted from the input string. It can contain whitespace characters, non-whitespace characters (except %), and format specifiers. - &var_name1, ... :
These are the additional-arguments that sscanf takes to store the values (or items) extracted from the string we read. Each argument should be a pointer to a variable whose data type is the same as the corresponding format specifier. The number of these additional arguments should be at least equal to the number of format specifiers in the format_string string. If the number of arguments is more than the number of format specifiers, the sscanf() function ignores the redundant arguments.
Note :
In a format_string, the whitespace characters are used to separate two items/values, the format specifiers are used to read items, and the non-whitespace characters are not read at all.
Return Value of sscanf() Function in C
- Return type on a successful execution : int (any integer greater than or equal to 0)
- The return value in case of an input failure (string could not be read) : EOF (EOF denotes the end of an input string)
On successful execution, the sscanf() function returns the count of successfully assigned additional arguments. This count value ranges from the expected number of items to zero (in case of a format mismatch failure).
Example
Let us look at a simple example to get a basic understanding of the sscanf() function in C.
In this example, we will use sscanf() to read data from a string and store the read data in another variable.
Output :
In this example, we had a string str containing a single word, "Programming". To extract this word from str, we used the sscanf() function. Because we wanted to extract text from the string, we used "%s" as the format_string. The value extracted by sscanf() was stored in the extracted_value variable.
Now, because we extracted one value using sscanf(), sscanf() returned the value 1. This value was stored in the items_read variable. Finally, we printed the extracted_value and items_read variables.
Exceptions of sscanf() Function in C
The sscanf() function usually returns a positive int value that depicts the number of items it reads. However, in some cases, it might return unexpected values. These exceptions are:
- Format mismatch :
If there is a format mismatch between the format specifier and the corresponding item in the given string, sscanf might return a number that is smaller than the value we expected. - Input failure :
If sscanf is unable to read a string, it returns EOF instead of a number. - Other errors :
In case of any error other than an input failure, sscanf returns -1.
What is sscanf() Function in C ?
In the C programming language, sscanf stands for string scanf. The function sscanf in C is a file handling function. It is used to read input from a string, a buffer (temporary storage that stores input/output commands), or a character array. The input read by sscanf is stored in the locations given in additional-arguments.
A string containing multiple words, separated with whitespace characters (like space characters or new line characters) can be used as input in the sscanf() function. In this case, The sscanf() function will read all words, not just the first one.
More Examples
Example : 1 - Reading Multiple Items of Different Data Types
Output :
In the above example, we used sscanf() to extract the brand name, model name, and model number from the string phone_str. Because the string phone_str contained 2 texts (separated by whitespace) and 1 number, we used 3 format specifiers "%s %s %f" to read values from the string.
Note :
that to read every item, the additional-arguments : brand, model_name, and model_number should have data types corresponding to the format specifiers.
In "%s %s %f", the first %s sign extracted Samsung from the given string and stored it in the brand variable, the second %s extracted M from the given string and stored it in the model_name variable, and %f extracted the number, i.e. 20.1 from the given string and stored it in the model_number variable.
As we were able to successfully assign values to three variables, sscanf() returned the value 3.
Example : 2 - Reading a Few Items from a String Literal
If a string literal contains multiple items/values, it is not mandatory to read all the items. We can also read (and extract) only a few items. We use non-whitespace characters to ignore the characters that we do not want to extract.
Output :
In the above example, we had a string str containing the text "Varun is 23years old and owns iPhone12". We only wanted to extract useful information from the string, i.e. name, age, and phone. To do so, we used this format_string : "%s is %dyears old and owns %s".
We know that the non-whitespace characters in the format_string are not extracted by sscanf (except the format specifiers). So, the three format specifiers we wrote will extract items that correspond to the text in the string str, i.e., the first %s extracts Varun, %d extracts 23, and the second %s extracts iPhone12. These extracted values were stored in the variables name, age, and phone, respectively. Finally, sscanf returned 3 because we extracted three values from the string str.
Conclusion
- The function sscanf in C is used to read input from strings.
- The sscanf function returns an int value that depicts the number of items it read.
- If sscanf in C is unable to read a string input, it returns EOF. It returns -1 in case of any other error.
- The sscanf function allows us to either read a few items or read all items from a string input.