What are Static Binding and Dynamic Binding in C++?
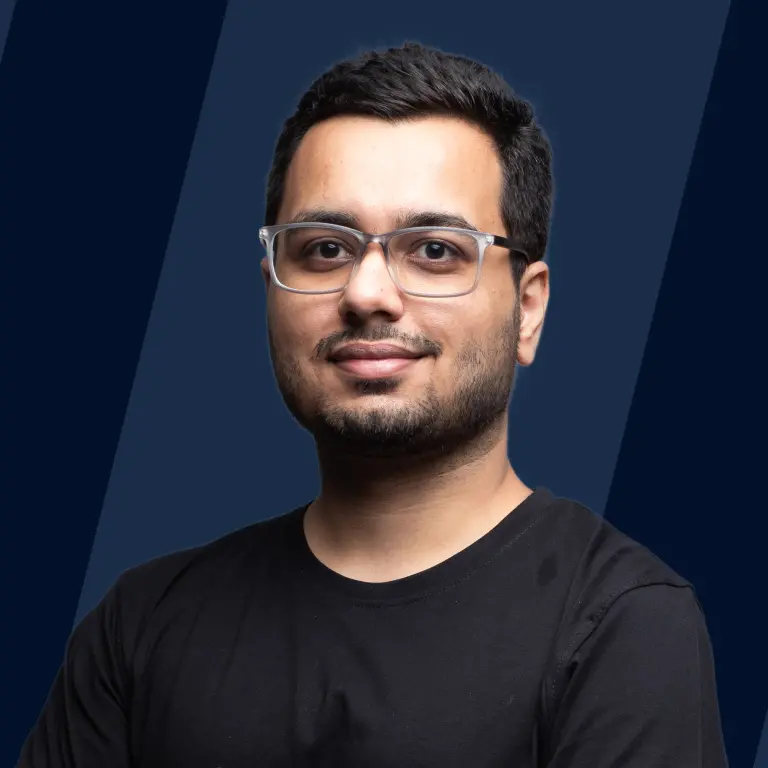
Binding helps in linking a bridge between a function call and its corresponding function definition.
Before getting to static binding & dynamic binding, we need to understand the concept of binding. When we create a function, we have two crucial things:-
- A function definition - defines a procedure to execute.
- A function call - invokes the respective function for implementation.
Now both the function definition and function calls are stored in the memory at separate addresses. And our program can have more than one function for its smooth operation. Hence, we need a technique to match the appropriate function call with its definition.
The process of matching a specific function call to its respective function definition is known as binding. But why is this of such interest? A lot can be utilized using the two kinds of binding: static binding & dynamic binding.
Before looking at static binding & dynamic binding, let us understand the need for binding with a practical example. Suppose we have a class bind with two member functions. The class definition, along with the driver code, is as follows :
Now let’s see the Output:
If there was no way of binding in C++, the two calls & definitions could’ve mixed up. Hence, binding is needed to link a function call with its function definition accurately.
When this binding occurs at Compile time, we call it a Static Binding, and when this binding occurs at Runtime, we call it a Dynamic Binding.
Now that we know about binding, we shall move to static and dynamic binding in C++.
What is Static Binding?
Binding at compile time is known as static binding.
There is always a default setting for any tool or method. For binding, static binding in C++ is the default route. Static binding ensures linking the function call and its function definition at compile-time only. It is also why it is synonymous with compile-time binding or early binding.
But how does the linkage take place? Before getting into a practical example, there are two ways by which static binding can be achieved: function overloading & operator overloading. Overloading is a technique through which the compiler uniquely identifies each function definition when it is called or linked. It locates by matching the parameters passed to those functions.
There are some known advantages associated with static binding in C++ :
- It performs better than a dynamic binding in C++. The compiler knows before runtime about all the methods of the class & is aware of which ones can or can’t be overridden. Hence, it is easier for the compiler to associate the objects with their respective class(es). It concludes with not needing an extra overhead.
- Better performance also results in being more efficient and faster.
The downside of static binding is that it reduces flexibility. All information about the values of parameters and function calls is predefined and cannot be changed at runtime.
Now that we are grasping some concepts of static binding let us look at an example to get through it.
Examples of Static Binding
We have seen that static binding in C++ is implemented using function overloading & operator overloading. Hence, let’s start with static binding using function overloading.
Function Overloading
When two or more functions have the same name but differ in the types or number of parameters they accept, it is known as function overloading. It is a feature of object-oriented programming.
Now let us create a class with member functions only to depict static binding in C++. We will return a different number of parameters to overload our member functions.
Note: First, we called the function with three parameters on purpose to check if the function calls & definitions are linked correctly or not.
Let’s check our output to see if we got the desired result:
As the above image depicts, the output matches our function calls. The function with three parameters is executed first, following the one with two parameters.
Operator Overloading
Usually, we use operators to do mathematical calculations, but if we want to do operations on the objects of a class, how can that be performed? It takes place using operator overloading. In operator overloading, we give a special meaning to our operator to perform operations on objects of a class. Hence, we overload our desired operator.
We can perform unary operator overloading that works on one operand only or binary operator overloading that works on two operands. For this example, we will consider binary operator overloading.
Let us try to add two numbers using a class. The class definition with the driver code is as follows:
In the given class, we have provided an uncommon meaning to our addition symbol (+). By overloading it, we will operate on two objects of a class, something unusual in basic mathematics.
The output of the code snippet is :
What is Dynamic Binding?
Binding at runtime is known as dynamic binding.
There are instances in our program when the compiler cannot get all the information at compile time to resolve a function call. These function calls are linked at runtime. Such a process of binding is called dynamic binding. Since everything is postponed till runtime, it is also known as run-time binding or late binding.
In C++, it is executed using virtual functions. A virtual function is a member function in the base class that is overridden (re-defined) by its derived class(es). When declaring in the base class, we use the keyword virtual to distinguish it.
Some of the many advantages of dynamic binding in C++ are :
- It is very flexible as one function is used to handle different objects.
- It also helps in reducing the overall size & making our program readable.
However, since the linkage takes place during runtime, it can make the program slow. This is one of the main disadvantages of dynamic binding. Now, let’s look at how to use dynamic binding using virtual functions.
Example of Dynamic Binding
As noted earlier, dynamic binding is achieved using virtual functions. This section will focus on a simple explanation along with a practical example.
Virtual Function
It is a function in the base class inherited by the derived class. The functions have the same name, but their functionality can differ as required. We know how to access base class member functions. But how do we access it in the derived class? We use a pointer or a reference to the base class and access the derived class version of the function.
Let us see the syntax of a virtual function:
Note: The keyword virtual only needs to be mentioned in the base class.
Now that we have learned about virtual functions let us move on to practical implementation. To simplify things for everyone to understand, we will take our base class as ‘base’ and derived class as ‘derived’.
What will the output of the above code snippet be? Before moving on, figure out & implement yourself. The desired output is as follows :
Dynamic binding in C++ runs successfully using a pointer. Here the pointer stores the location of the derived class. The pointer ptr is assigned the address of object d of the derived class. Hence, it executes the member function of that class.
If we want to execute the member function of both the base class and the derived class, our driver code will look like this :
Hence, the narrative changes here & we get to perform two different procedures using less space & more flexibility. The output is as follows:
We twisted a bit here by first accessing the derived class's member function.
What is the Difference Between Static Binding & Dynamic Binding?
Basis of Comparison | Static Binding | Dynamic Binding |
---|---|---|
Event Occurrence | During compile time only. It is by default in C++. | During the run time. |
Alternate Name(s) | Early Binding, Compile-time Binding | Late Binding, Runtime Binding |
Information Available | All the information to resolve function calls is available simultaneously. | The compiler cannot determine all the information at compile time. |
Advantage | It is efficient while executing as all the information is available before runtime. | It is flexible as different types of objects are handled at runtime by a single member function. |
Speed | It is faster. | It is slower. |
Example | Function overloading or operator overloading in C++. | Virtual functions in C++. |
Learn More about the Concepts of C++
We are thorough with the primary concepts of static binding and dynamic binding in C++. There are many more concepts crucial in the fundamentals of C++. Some areas we have already visited include function overloading, operator overloading & virtual functions. To understand better about these topics & pace on in your journey to C++, visit here.
Conclusion
- Binding provides a linkage between a function call and its definition.
- There are two kinds of binding: static binding & dynamic binding in C++.
- Static binding works during compile time and performs better.
- Static binding can be applied using function overloading or operator overloading.
- Dynamic binding works during run time & is more flexible.
- Dynamic binding can be accomplished using virtual functions.