Static Block in Java
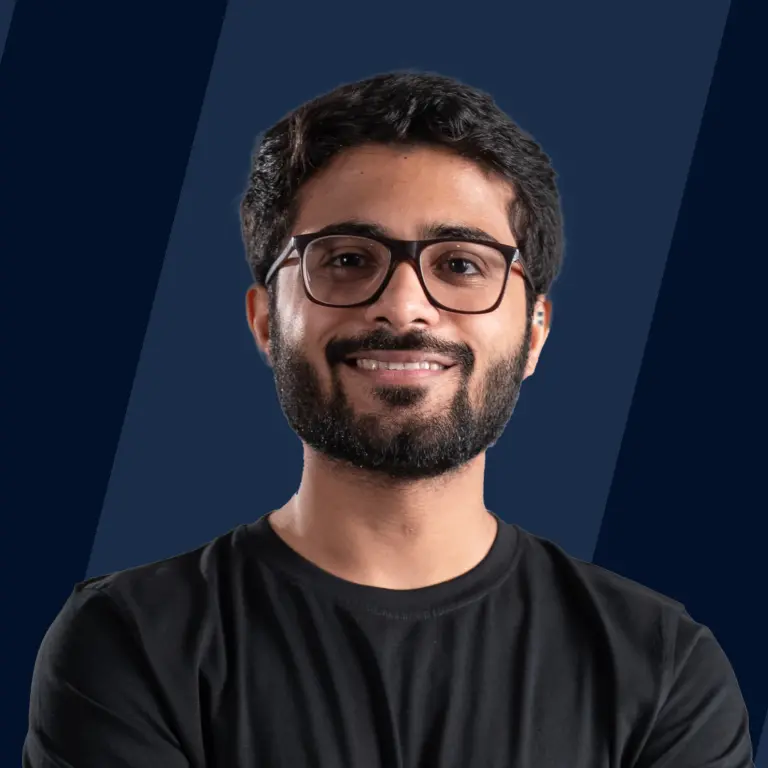
This article will teach us about the static block in Java. A static block is a block associated with the static keyword. The set codes inside the static block can run only once when a class is loaded into memory. A static block is also known as a static initialization block. It is not supported in C++. Having got an overview, let us understand static blocks in Java in depth in this article.
Introduction to Static Block in Java
A static block in Java is a code associated with the static keyword, executed only once when the class is loaded into memory by the Java ClassLoader. It is used for static initialization of a class, ensuring that certain fields or operations are performed just once, typically at the beginning of the program's execution.
The static keyword is a non-access modifier in Java that makes a member (variable or method) independent of class instances. It signifies properties common to all objects in the class, with only one copy of the static member existing regardless of the number of class instances. This keyword can be used with variables, methods, blocks, and nested classes.
By leveraging a static block, we can initialize static fields or execute necessary operations at class loading time, ensuring efficiency and avoiding redundant initialization during the program's runtime. This makes static blocks crucial for setting up a class during runtime while ensuring the code inside executes only once. Therefore, static blocks are also referred to as static initialization blocks, contributing significantly to Java classes' structure and initialization process.
A static block is executed before the main method during the classloading.
Let us see an example of declaring a static block in Java.
Output
Explanation
So in the above code, we have created a public class Main, and inside it we have created a static block. The static block is automatically executed during the loading of the class; at first, the class is loaded into the memory; that is, JVM loads the dot class file (in byte code) into the memory. During the dot class file loading into memory, the static block is executed, and it will print its content.
Note : In the above code, there is no main() method, so this code will only work for a JDK version 1.5 or previous. In the latest version, it will throw an error.
Calling of Static Block in Java
Now, let us discuss what a static block in Java is called. There is no specific way to call a static block in Java, as it is executed automatically when the class is loaded in memory.
To clarify the above point, let us take an example of how the static block in Java is called automatically.
Output
Explanation
The above code has a public class Myclass, which includes a constructor, method, and a static block. In the main method, we can see that, except for the static block, we are calling the constructor method of that class, but after the execution of the class, we can see the elements: "one", "two, "three", "four", in the list is added inside the static block, which is before the elements "five", "six" in the list which is been added inside the constructor. This happens, even though we have not called the static block inside the main() method. You can also see that the content of the static block is executed before the constructor.
Hence, we may clearly conclude that, we don't need to call the static block manually, rather it is executed automatically at the very beginning whenever the class is loaded in the memory.
Execution of Static Block in Java
The static block is executed only once by the Java virtual machine (JVM) when the class is loaded into the memory by Java ClassLoader. Usually, in JDK version 1.5 or previous, the static block can be executed successfully without the main() method, this is because the static block is executed before a valid main() method is searched for, so if you exit the program at the end of the static block, you will receive no errors. However, in case of JDK version after 1.6 it will throw an error if there is no main() method inside our code. As in JDK version after 1.6, after loading the class the JVM will check for the main method before loading static content.
During class loading, even before the main method is loaded, the JVM executes the static block.
Hence, let us take an example of the execution of a static block in java.
Code
Output
Explanation
So in the above code, we have a public class Main which includes a static block and a main method. Usually in JDK version 1.5 or previous we can execute the code successfully and print the content inside a static keyword without creating any main() method, but JDK version after 1.5 will throw an error if there is no main() method. So in the latest versions, JVM first looks for the main method and then, starts executing the program including the static block.
Static blocks can also be executed before constructors. Let us see another example of how the static block will execute if the code contains constructors.
Code :
Output
Explanation
In the above code, we have a public class MyClass and another class Helper. When the code is been executed we can see the static block content is printed before the constructor of the Helper class content. By this, we got a clear idea that static blocks can also be executed before constructors. We have also noticed although we have two objects, the static block is executed only once. This is because the static block is executed by default during the loading of the class.
Why is the Static Block Executed Before the Main Method?
The static block is executed before the main method because the Java Language Specification directed that static blocks must be executed first.
When a particular class is executed, The java virtual machine (JVM) performs two actions during the runtime. They are as:
- At first, the class is loaded into the memory, that is, JVM loads the dot class file (in byte code) into the memory.
- During the dot class file loading into memory, the static block is been executed. After the loading of the dot class file, the main method is been called for execution. Therefore, the static block is executed before the main method.
In other words, we can also say that the static block is stored in the memory during the time of class loading and before the main method is executed, so the static block is executed before the main method. Let us see an example to demonstrate that the static block is executed before the main method.
Code :
Output :
Explation :
So in the above code, we have a public class Main which contains a static block and a main method. We can clearly see the content inside the static block is printed before the main() method content. Hence, we can say that the static block is executed before the main() method.
How Many Times does a Dot Class File Loaded into Memory?
A dot class file is loaded into the memory only one time during runtime. So, the static block will be executed only one time during the loading of the dot class file into the memory. Although the instance block’s execution depends upon the object creation, that is the number of times the object is created the instance block will be executed that number of times. You might be thinking about what is an instance block, so let's discuss it in a short overview.
instance block : Instance Initializer block is used to initialize the instance variable or data member. Every time an object of the class is created, it runs.
If we create 5 objects, the instance blocks will be executed 5 times but the execution of the static block depends upon the class loading. Since the class is loaded only one time, the static block will be executed only one time.
Let us take an example program to understand this concept better.
Code :
Output :
Explanation :
So in the above code, we have a public class MyClass which contains an instance block, static block, and a main method.
When the program is been executed the static block's content is printed at first and only one time during the loading of the class into the memory. AFter that, the instance block's content is printed 5 times as the object is called 5 times. Hence, we can say the static block is executed only once during the dot class file (.class) loading into the memory, and the instance block will be executed as many times as the object is created.
Order of Execution of Multiple Static Blocks in Java
In a java program, a class can have multiple static initialization blocks that will execute in the same order they appear in the program, that is, the order for the execution of static block in a class will be from top to bottom.
That is, during the loading of the dot class files, the order of execution of multiple static initialization blocks is executed automatically in sequence from top to bottom.
Let us take an example program to understand the order of execution of multiple static blocks.
Code :
Output :
Explanation
In the above code, we have a public class MyClass which contains an instance block, multiple static blocks, and a main method.
When the code is executed and during the loading of the class file, we can clearly see the contents inside the multiple static blocks are printed in order like the at first the static block-1 is executed then the static block-2 and it goes on in order from top to bottom and then after that, the main block is executed.
We can also see the content inside the instance block is printed only once as the object is created only once. in the end, the main method code is also executed. Hence, we can say the order of execution of multiple static initialization blocks is executed automatically from top to bottom during the dot class file loading.
Can we Execute the Static Block without the Main Method inside a Class?
In Java Development Kit (JDK) version 1.5 or previous the static block can be executed successfully without the main() method inside the class, but JDK version after 1.5 will throw an error message if there is a static block but no main() method inside the class.
The static blocks are essentially executed during the loading of the dot class (.class) file, even before the JVM calls the main method.
Let us take an example program running on JDK version 1.5 or Previous where we will not declare the main method in the class and see what happens.
Example-1 : Running on JDK version 1.5 or Previous
Code :
Output :
Explanation :
In the above code, we have a public class MyClass which contains only a static block we can clearly see the code is executed successfully and it is able to print the static block's content that is "Static block can be printed without main method". Hence, in JDK version 1.5 or previous, it is possible to execute static block without a main method inside the class.
Let us take an example program running on JDK version 1.5 and later where we will not declare the main method in the class and see what happens?
Example-1 : Running on JDK version 1.5 and later
Code :
Output :
Explanation :
So in the above code, we have a public class MyClass which contains only a static block. We can clearly see the code is unable to execute successfully and it throws an error: Main method not found in class MyClass demanding for defining the main method.
Hence, in JDK version 1.5 or later it is not possible to execute a static block without a main method inside the class.
Use of Static Block in Java
There are many uses of static blocks in java, however, let us discuss the primary uses of static blocks in java:
- Use static initialization blocks to execute logic or code before the main method or even before the class's initialization.
- Static initialization blocks are commonly used to change the default values of static variables in Java. These variables belong to the class and are initialized during class loading.
- They are handy for initializing static fields that require complex setups or multi-step processes. For example, initializing a map of country codes to names.
- Static blocks can also be used to set up static resources or ensure other classes are loaded before execution. This ensures the code runs only once.
- They are useful when initialization code may throw exceptions. Handling exceptions allows for graceful error management, such as catching IOExceptions and handling them appropriately.
- In cases where you need to execute code before a constructor, especially if you need to perform actions before the superclass's constructor is called, static blocks provide a solution. They are guaranteed to execute before any constructor in the class.
Difference between Static Block and Instance Block in Java
We have got a clear idea about what is a static initialization block. We have also got a small overview of the instance block in Java. Let us now discuss the difference between a static block and instance block in java.
The differences between instance block and static block in java are as follows:
Static Block | Instance Block |
---|---|
A static block is also known as a static initialization block. | A instance block is also known as instance initialization block or non-static block. |
The Static blocks execute before instance blocks in java. | The instance block executes after the static blocks. |
Only static variables can be accessed inside the static block, if we try to access any non-static variable (instance variables) inside the static block it will throw an error stating non-static variable cannot be referenced from a static context. | The static and non-static variables (instance variables) can be accessed inside the instance block. |
The static blocks can be used for initializing static variables or calling any static method in java. | The instance blocks can be used for initializing instance variables or calling any instance method in java. |
Static blocks execute in memory during the loading of its dot class (.class) file. | The instance block executes only when the instance of the class is created, not called during the loading of its dot class (.class) file in memory in java. |
Inside a static block we cannot use the this keyword | Inside an instance block we can use the this keyword. |
Let us understand what is this keyword in java.
this keyword : The this keyword refers to the current object in a method or constructor. The this keyword is mostly used to distinguish between class attributes and parameters with the same name and remove confusion (because a class attribute is shadowed by a method or constructor parameter). Let us take an example program of this to understand this more clearly.
Code :
Output :
Explanation : In the above code, we have a public class Main, which consists of a constructor with parameter and the main() method. Here, when the class constructor is called inside the main() method with a value, we store that argument value inside a class or instance variable with the this keyword. We did this to eliminate the confusion between class attributes and parameters with the same name (because a class attribute is shadowed by a method or constructor parameter). If you omit the keyword in the example above, the output would be 0 instead of 15.
Conclusion
- A static block is a block that is associated with the static keyword.
- The static keyword is a non-access modifier in Java. That is, the static keyword makes a member (variables or methods) of a class independent of its objects. It is used when we are defining properties that are common to all objects in the class.
- The static block is stored in the memory during class loading and before the main method is executed, so the static block is executed before the main method. It runs once when the class is loaded into the memory.
- A dot class file is loaded into memory only once. So, the static block will be executed only once while loading the dot class file into memory.
- The Instance Initializer block is used to initialize the instance variable or data member. It runs every time a class object is created.