Staticmethod() in Python
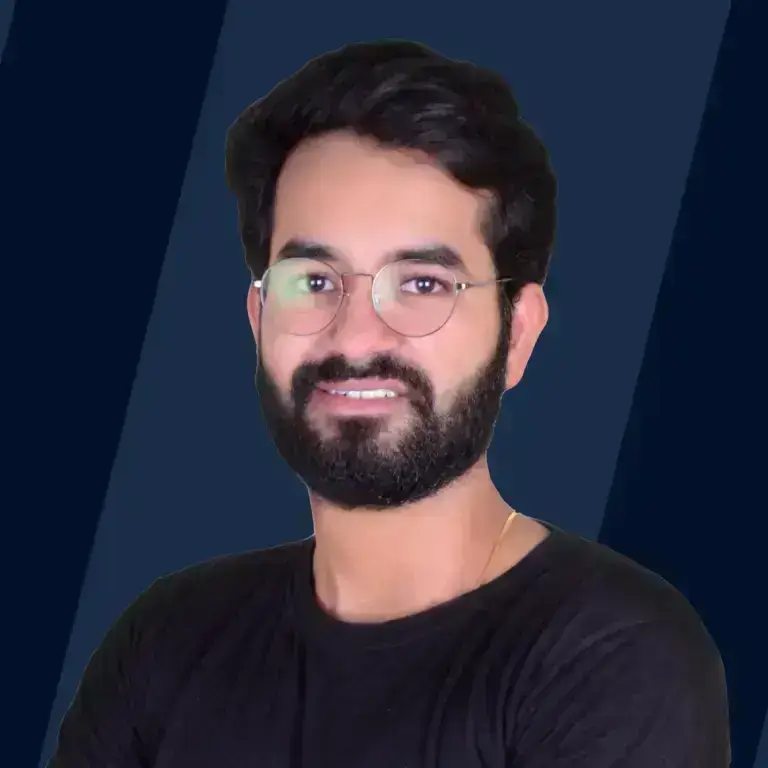
The @static method in Python is similar to class-level methods in Python, the difference being that a static method in Python is bound to a class rather than the class objects. This means that a static method can be called without an object for that class and that a static method cannot modify the state of an object as it is not bound to it.
staticmethod() Syntax
The Syntax of @staticmethod in Python is:
Note: static method in python is considered an un-pythonic form of creating a static function. Hence, in newer versions of Python, you can use the staticmethod in python.
staticmethod() Parameters
The staticmethod() method takes a single parameter:
- function: - function that needs to be converted into a static method
staticmethod() Returns
The staticmethod() in python returns a static method for a function passed as the parameter.
what is static method in python?
Static method in python is associated with the class itself, not with any specific instance of the class. They do not require the creation of a class instance. So they are not dependent on the state of the object.
Static methods in Python are similar to Python class-level methods, the difference being that a static method is bound to a class rather than the objects for that class. This means that a static method can be called without an object for that class and also that static methods cannot modify the state of an object as they are not bound to it.
The main difference between a static method and a class method is:
- Static method knows nothing about the class and deals with the parameters.
- The class method works with the class since its parameter is always the class itself.
They can be called both by the class and its object.
Examples
-
Create a static method using staticmethod()
The code snippet below demonstrates how to use staticmethod in Python:
Output
In the above program, we converted the add function into a static method and called the Test.add function without creating an object of the class. This approach is controlled as creating a static method out of a class method at each place is possible.
-
Create a static method Using @staticmethod
The code snippet below shows a more subtle way of creating a static method:
Output
The above program uses @staticmethod, a built-in decorator that defines a static method in the class in Python. A static method doesn't receive any reference argument whether it is called by an instance of a class or by the class itself. The add function cannot have self or cls parameter.
When Do You Use the Static Method in Python?
Grouping Utility Functions to a Class
Sometimes, it makes sense to group functions that perform utility tasks related to a class but do not need to interact with class instances. These functions do not require access to the self parameter characteristic of class methods. Here's an example:
Example: Create a Utility Function as a Static Method
Output:
Explanation: In this example, is_weekend is a utility function that doesn't need to interact with any instance of the DateHelper class. It simply takes an input (a day of the week) and returns whether or not it's a weekend. This is a perfect use case for staticmethod since the method's logic is relevant to the class but does not require any class or instance data.
Having a Single Implementation
static method in python is also useful when you need a function that behaves the same way across all instances of a class and subclasses. This ensures that the method's implementation is not overridden in subclasses.
Example: How Inheritance Works with Static Method
Output:
Explanation: Here, generic_sound is a static method of the class Animal. When we inherit Animal into Dog, we do not override generic_sound in the Dog class. As a result, calling generic_sound on either Animal or Dog produces the same output. This illustrates how a static method maintains a single implementation across both the parent class and its subclasses, making it ideal for situations where the method's behaviour should not vary with inheritance.
Conclusion
- The staticmethod() built-in function returns a static method for a given function.
- A static method in python can be called without an object for that class, and that static method cannot modify the state of an object as it is not bound to it.
- Static methods have limited use because they don't have access to the attributes of an instance of a class (like a regular method does), and they don't have access to the attributes of the class itself (like a class method does).