String Class in Java
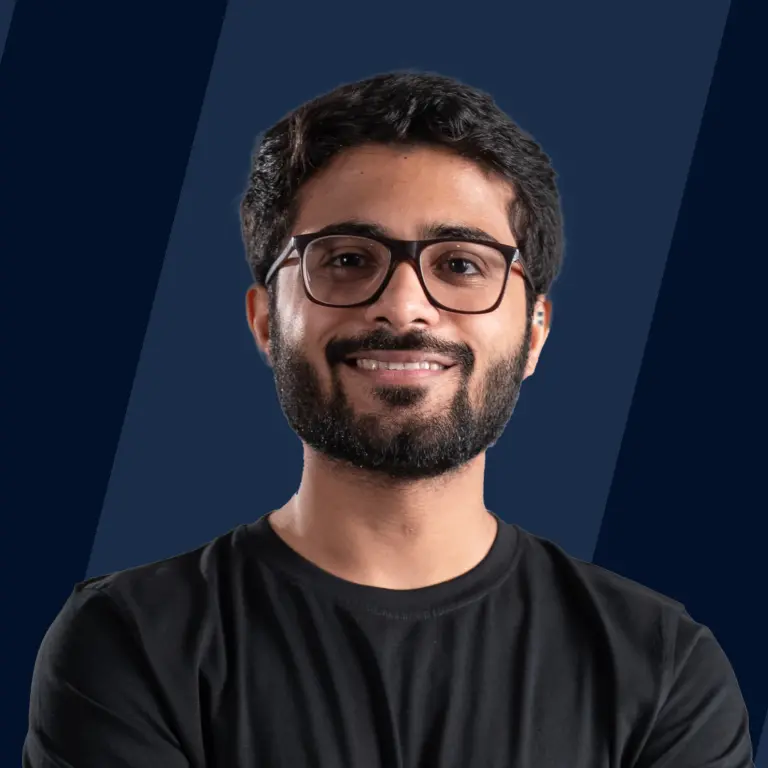
Overview
The use of strings is essential to programming. Java has a String class that allows for the creation and manipulation of strings. The character sequence can also be represented using an interface called CharSequence. One of the classes that implements this interface is the String class. Thus, in Java, a string is an object that is essentially a sequence of characters.
For instance, the word "hello" is made up of a string of the characters "h," "e," "l," "l," and "o." Additionally, we have a variety of String methods that make it simple to interact with String in Java.
What is String Class in Java?
String refers to a group of characters. String objects in Java are immutable, which simply means they can never be modified after they have been created. Java only supports operator overloading for the String class. The + operator allows us to combine two strings. For instance, "a"+"b"="ab." StringBuilder and StringBuffer are two helpful classes in Java that can be used to manipulate strings.
How to Create a String Object in Java?
A few of the more well-known techniques to generate a string object in Java are listed below.
By String Literal
The most typical method of producing string is this one. In this instance, double quotes are used to surround a string literal.
When double quotes are used to generate a String, JVM searches the String pool(A location inside the heap memory is called the String Constant Pool. It includes distinctive strings. The JVM determines whether a string is already present in the string pool before generating a new object in the pool. ) to see whether any other Strings with the same value are already stored there. If an existing String object is found, it simply returns the reference to it; otherwise, a new String object with the specified value is created and stored in the String pool.
Using New Keyword
Like any other Java class, we can construct String objects with the new operator. The String class has a number of constructors that can be used to create a String from a char array, byte array, StringBuffer, or StringBuilder.
Constructors of String Class in Java
1. String()
produces a blank string. Due to the immutability of String, it is largely worthless.
2. String(String original)
from another string, generates a string object. String is useless since it is immutable.
3. String(byte[] bytes)
creates a new string using the system's default encoding from the byte array.
4. String(byte bytes[], String charsetName)
utilises the character encoding supplied. UnsupportedEncodingException is thrown if the encoding is not supported.
5. String(byte[] byte_arr, Charset char_set)
In order to create a new String, decode the byte array. It decodes using the char set.
6. String(byte bytes[], int offset, int length)
The first byte to be decoded is indicated by the offset. The length defines how many bytes need to be decoded. If offset, length, or offset is more than bytes, this constructor raises an IndexOutOfBoundsException.
7. String(byte bytes[], int offset, int length, Charset charset)
Similar to the constructor above, the only difference is that the encoding to be used must be specified.
8. String(byte bytes[], int offset, int length, String charsetName)
The character set encoding name is supplied as a string, unlike the example before. If the encoding is not supported, this will throw an UnsupportedEncodingException.
9. String(char value[])
uses the character array to generate the string object.
10. String(char value[], int offset, int count)
uses the character array to generate the string object.
11. String(char value[], int offset, int count)
The initial character's index is specified by the offset. The amount of characters to use is determined on the length. If offset, length, or offset and value are negative, this constructor raises an IndexOutOfBoundsException.
12. String(int[] codePoints, int offset, int count)
Creates a string from an array of Unicode code points as input. If any of the code points are incorrect, it raises the IllegalArgumentException exception. If the offset length, or offset is more than codePoints, this constructor raises an IndexOutOfBoundsException.
13. String(StringBuffer buffer)
uses the data contained in the string buffer to produce a new string. Internally, this constructor uses the StringBuffer function toString()
14. String(StringBuilder buffer)
Takes the contents of the string builder and produces a new string.
Methods of String Class in Java
We will now talk about Java's methods for Strings. String Methods are highly helpful since they make working with Strings in Java very simple. The String methods in Java allow us to do many different things, including determining a string's length, concatenating several strings, determining whether two strings are equal, changing a string's characters' case, and much more. Let's talk about a few of Java's most well-liked and frequently utilised String methods. It should be noted that the Java class java.lang.String offers all of these string methods.
Method | Description |
---|---|
char charAt(int index) | It provides a char value for the specified index. |
int length() | return the length of string |
static String format(String format, Object... args) | returns a formatted string |
static String format(Locale l, String format, Object... args) | It gives back a formatted string in the specified locale. |
String substring(int beginIndex) | It provides a substring based on the begin index. |
String substring(int beginIndex, int endIndex) For the supplied begin index and finish index, a substring is returned. | |
boolean contains(CharSequence s) | After comparing the sequence of char values, it returns true or false. |
static String join(CharSequence delimiter, CharSequence... elements) | It returns a joined string. |
static String join(CharSequence delimiter, Iterable<? extends CharSequence> elements) | It returns a joined string. |
boolean equals(Object another) | It determines whether the string and the supplied object are equal. |
boolean isEmpty() | check if string is empty or not. |
String concat(String str) | It concatenates the string with the supplied string. |
String replace(char old, char new) | It replaces the old char with the new char. |
String replace(CharSequence old, CharSequence new) | It changes the supplied CharSequence anywhere it appears. |
static String equalsIgnoreCase(String another) | It compares a different string. Case is not checked. |
String[] split(String regex) | A split string that matches the regex is returned. |
String[] split(String regex, int limit) | It gives back a split string that matches limit and regex. |
String intern() | An interned string is returned. |
int indexOf(int ch) | The requested char value index is returned. |
int indexOf(int ch, int fromIndex) | Starting with the supplied index, it returns the specified char value index. |
int indexOf(String substring) | The requested substring index is returned. |
int indexOf(String substring, int fromIndex) | The required substring index beginning with the supplied index is returned. |
String toLowerCase() | returns string in lowercase. |
String toLowerCase(Locale l) | |
String toUpperCase() | returns string in uppercase. |
String toUpperCase(Locale l) | IIt gives back an uppercase string using the chosen locale. |
String trim() | removes leading and trailing whitespace. |
static String valueOf(int value) | returns a string representation of the supplied int value. |
Examples of String Class in Java
Example 1
In this example we are going to discuss some methods of string class in java
Output:
Code Explanation :
In Above example first we have used length() method to find the length of string(Hello World) that print 10. Then we have used charAt() method that print the character at given position. substring() method print the substring of string s with starting index as 3. Then concat() method adds two string so Hello World is printed.After that we have called indexOf() that will returns the index at which World is found.We have used other methods like equal() equalsIgnoreCase() to check equality of two strings with and without ignoring case,used compares() to find difference between ASCII value.
Conclusion
- Strings are objects in Java that internally consist of a string of characters. Simply put, a string is a grouping or arrangement of characters.
- Newly created strings are kept in a specific location in the heap known as the String Constant Pool or String Pool.
- Since strings are considered objects in Java, they can be generated using both the new keyword and string literals.
- Java has a variety of methods for Strings that can be used to make working with strings in Java simple.
- The + operator and Java's concat() function are the two methods for concatenating strings.
- Since strings in Java are immutable, their value cannot be modified once it has been initialised (or created).