String Comparison in Python
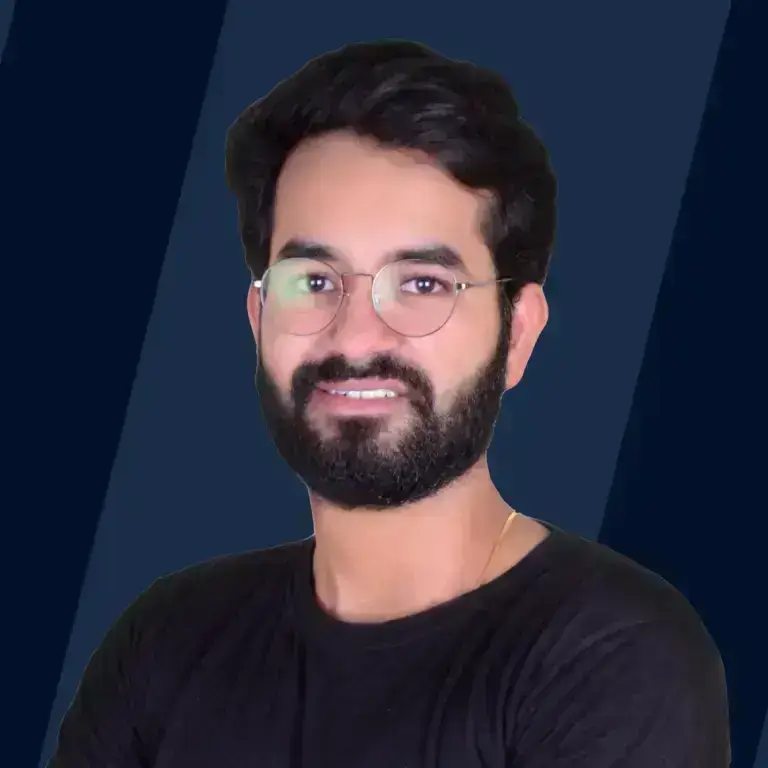
String comparison in Python is essential for sorting, validating, and analyzing text, involving methods to check equality, lexicographical order, and pattern matching, adaptable for both case-sensitive and -insensitive scenarios.
How to do String Comparison in Python?
Python string comparison involves determining the equality or relative order of strings using various methods. From basic operators to user-defined functions and advanced libraries, this guide covers essential techniques to compare strings effectively in Python.
Python offers multiple methods for comparing strings, each suited to different scenarios.
- Method 1: Using Relational Operators
- Method 2: Creating a User-defined Function
- Method 3: String Comparison Using Python str Method
- Method 4: Using Regular Expression
- Method 5: Using Is Operator
Let's discuss each method one by one:
Method 1: Using Relational Operators
Relational Operators are used for comparing values in Python. Relational operators are generally used for numeric values but they can also be applied to the string. Relational operators return true or false depending upon the condition. In the case of strings, relational operators sequentially compare each character of both strings according to the characters' Unicode value. As comparison is done for each character, relational operators maintain lexicographical order.
Operators
-
less than(<)- This operator returns True if the first string is lexicographically smaller than the second string. Otherwise, it returns False. It has the syntax: str1 < str2 where str1 and str2 are the strings to be compared.
-
greater than(>)-This operator returns True if the first string is lexicographically larger than the second string. Otherwise, it returns False. It has the syntax: str1 > str2 where str1 and str2 are the strings to be compared.
-
less than equal to(<=)-This operator returns True if the first string is lexicographically smaller than or equal to the second string, otherwise it returns False. It has the syntax: str1 <= str2 where str1 and str2 are the strings to be compared.
-
greater than equal to(>=)-This operator returns True if the first string is lexicographically larger than or equal to the second string. Otherwise, it returns False. It has the syntax: str1 >= str2 where str1 and str2 are the strings to be compared.
Example
In this example, we have created two strings str1 and str2. Then we compared these two strings using the above-mentioned relational operators.
Output
Read more about Relational Operators in Python
Method2: Creating a user-defined function
1. Checking if Strings are equal
We can also have a user-defined function to compare two strings. This function would return whether the strings are equal or not.
Here we have implemented the comp_str function to compare two strings. First, the function checks whether the strings are of the same length. If they have different lengths, it returns that the strings are not equal. Otherwise, it compares characters at the same indices of both strings. If a mismatch is found, it returns that the strings are not equal. If no mismatch is found after comparing all the indices, it returns that the strings are equal.
Output
2. Finding Lexicographical order of Strings
We can also have a user-defined function to compare two strings. This function would return the string that is lexicographically(alphabetically as in a dictionary) larger. Here we have implemented the comp_str function to compare two strings. It compares characters at the same indices of both strings. If a character of a string is found to have a larger ASCII value than the character of the other string, that string is the lexicographically larger string. Otherwise, the comparison continues to the next character. The strings are equal if all the characters of both strings have the same ASCII values.
Output
Method 3: String Comparison Using Python str Method
In Python, the str class provides various methods that facilitate string comparison beyond the basic equality or relational operators. Two such powerful methods are startswith() and endswith(). These methods are particularly useful for scenarios where you need to check if a string begins with or ends with a certain substring, which is a common requirement in string manipulation tasks such as parsing file paths, URLs, or any data that follows a specific pattern.
Using startswith()
The startswith() method allows you to check if a string starts with a specified substring. It's a straightforward way to filter or categorize strings based on their prefixes. This method is case-sensitive, meaning that "Hello" and "hello" would be considered different substrings. However, you can combine this method with lower() or upper() to perform a case-insensitive comparison.
Example:
In this example, filename.startswith('report') returns True because the filename indeed starts with the substring 'report'. However, filename.startswith('Report') returns False due to the case difference.
Using endswith()
Conversely, the endswith() method checks if a string ends with a specified substring. This is particularly useful when working with file extensions, URL paths, or any string data where the suffix carries significant meaning.
Example:
Here, url.endswith('/page') evaluates to True because the URL string ends with the substring '/page'. The case-sensitive nature of this method results in url.endswith('/Page') returning False.
Combining Methods for Enhanced Comparisons
For more complex comparison needs, these methods can be combined with other string methods or used within list comprehensions and loops to filter or process collections of strings efficiently. For example, to find all files in a list that have a certain extension, you could use a list comprehension with the endswith() method.
Example:
This example filters out the list of filenames, selecting only those that end with the '.txt' extension.
Method 4: Using Regular Expression
Regular Expressions (Regex) offer a highly flexible and powerful method for string comparison in Python, enabling pattern matching and complex string analysis beyond simple equality checks. Using the re module, Python can perform case-sensitive and case-insensitive string comparisons, search for patterns, and much more.
Case-Sensitive Comparison
For a direct and case-sensitive comparison, re.match() is typically used. It checks if the specified pattern is found at the beginning of the string. The syntax re.match(pattern, string) returns a match object if the pattern is found, and None otherwise.
Case-Insensitive Comparison
To perform a case-insensitive comparison, you can use the re.IGNORECASE flag. This allows for flexibility in matching strings regardless of their case.
Advanced Pattern Matching
Regular expressions shine when you need to find specific patterns within strings, not just at the beginning. For instance, re.search() can locate a pattern anywhere in the string, and re.findall() can find all occurrences of the pattern. This is particularly useful for searching or extracting information from text data.
Method 5: Using Is Operator
In Python, string comparison can also be performed using the is and is not operators, which are fundamentally different from the commonly used == and !=. These operators do not compare the content of the strings but rather their identities, i.e., whether they point to the same object in memory.
This distinction comes from Python's optimization technique known as "string interning," where immutable objects like strings may be stored only once in memory for efficiency. This means if two string variables point to the same literal value, they might actually reference the same object in memory, having the same unique ID.
Using is Operator
The is operator checks if both operands refer to the same object, returning True if they do, and False otherwise.
Example:
Consider the following example where we compare two strings using the is operator:
In this example, str1 and str2 point to the same interned string, thus having the same ID, resulting in True for str1 is str2. However, str1 and str3 do not share the same ID, leading to False. Even after modifying str1 to match str3's value, they remain distinct objects, hence str1 is str3 still results in False.
Using is not Operator
Conversely, the is not operator checks if two operands do not refer to the same object, returning True if they don't, and False otherwise.
Example:
Using the same variables from the previous example, we get:
Here, str1 is not str2 yields False because they refer to the same object initially. In contrast, str1 is not str3 gives True both before and after modifying str1, as they never point to the same object.
Conclusion
- In this article, we have discussed the process of string comparison.
- This is followed by different methods to compare strings.
- Then we have also seen the process of comparing strings both in a case-sensitive and case-insensitive manner.
- That is followed by methods to compare different permutations of a string.