String Concatenation in C++
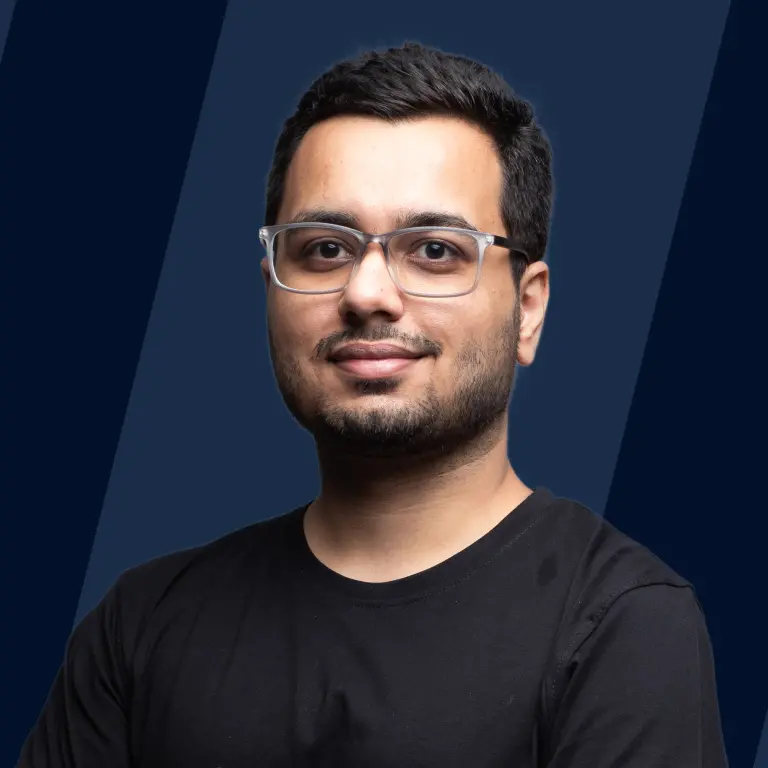
Overview
String concatenation in C++ is the joining of string characters end-to-end. There are many ways to concatenate a string, like using the loops, strcat() function, append() function, and "+" operator. The different types of concatenation are applied to different types of strings; for C strings, we use strcat() whereas for normal string objects, we use the "+" operator and append() function.
Introduction to String Concatenation in C++
As you may know, there are two types of strings in C++. first are one-dimensional arrays of characters that terminate with a null character called C strings, and another is just strings that are the object of string class. String concatenation in C++ occurs when at least two strings are combined, in which case the second string is added to the end of the first string.
Concatenate Two Strings Using a Loop
In the C++ language, we have two types of loops; let us take both of them into consideration to concatenate two strings.
- For Loop
- While Loop
1. C++ Program to Concatenate Two Strings Using Loop
Approach:
We use a string traversal approach to solve this program using for loops. Since there are two strings, we're using two for loops. During the string traversal, each character of that string is stored inside a first string variable, and the same string variable is used again to store the second string. Hence, we get concatenated string as output.
Code:
Output:
Explanation :
As you can see in the above C++ program, we have two strings, s1, and s2. Since we are using for loops to concatenate strings. For the traversal over the first string, we use a for loop and store it inside a string variable named outcome.
Again we use a for loop for the traversal over the second string s2 and store the string s2 inside the string variable outcome just after we already stored s1. As a result, we get the concatenation of two strings, s1 and s2.
2. C++ Program to Concatenate Two Strings Using a while Loop
Approach:
The approach for concatenating two strings using the while loop is; first, we use for loop for the traversal over the first string to get the first empty index to add string2 characters, and use the while loop we insert the string2 characters in string1. First, we check if string2 is null or not; if it comes out to be not null, then we add the characters of the second string behind the first string. Hence, on printing string1 we get concatenated string as output.
Code:
Output:
Explanation:
In the above C++ program, we are concatenating two C strings using a while loop. Now using for loop string s1 is traversed until null is encountered. Now using the while loop, the characters of s2 are checked if the string is not equal to null; if it is true, then the code inside the while loop adds the characters of s2 after s1. Hence, the strings s1 and s2 get concatenated.
Concatenate Two Strings Using the "+" Operator
A "+" operator is an arithmetic operator in C++, but when applied to a string, it joins the second string to the end of the first string. To illustrate this better, let's look at one simple program.
Approach :
Simply add two strings using the + operator.
Code:
Output:
Explanation :
As you can see in the above C++ program, we have two strings, s1, and s2. Another string variable, outcome, is used to store the output of s1+s2, where the + operator adds the characters inside s2 behind s1 so that the string is concatenated.
Concatenate Two Strings Using the strcat() Function
In C++, we can concatenate two C strings using the strcat() function. We cannot concatenate two string objects using the stract() function. The declaration should be a character array, not a string; otherwise, it will give an error. An array of type C-string terminated by a null character is a C-string.
To illustrate this better, let's look at one simple program.
Approach :
Using the inbuilt string function starcat() to concatenate two C strings.
Code:
Output :
Explanation :
As you can see in the above C++ program, we have two char, s1, and s2. Now coming to the strcat function, we first have to include a header <string> to use strcat(). strcat() takes two char parameters and joins the second parameter to the end of the first. To print the concatenated string, we need to print the first parameter.
Concatenate Two Strings Using the append() Function
The append is a string function used to append one string behind the other. Let's see one example to illustrate it better.
Approach:
Using the inbuilt string function append() to add a second string behind the first string.
Code:
Output :
Explanation :
In the above C++ program, we have two strings s1 and s2. We use the C++ <string> append function. The append function appends the character of the second string, s2, into the first one, s1. To get the concatenated, we need to print the first string, s1.
Conclusion
- The concatenation of two strings happens when the second string is added to the end of the first string.
- In C++, we have two types of strings, C Strings and String objects, where the C strings are declared as a character array that terminates with a null character, and String objects are declared as a string.
- There are many ways to concatenate strings, like using the loops, + operator, strcat() function, and append() function.