String Join Method in Java
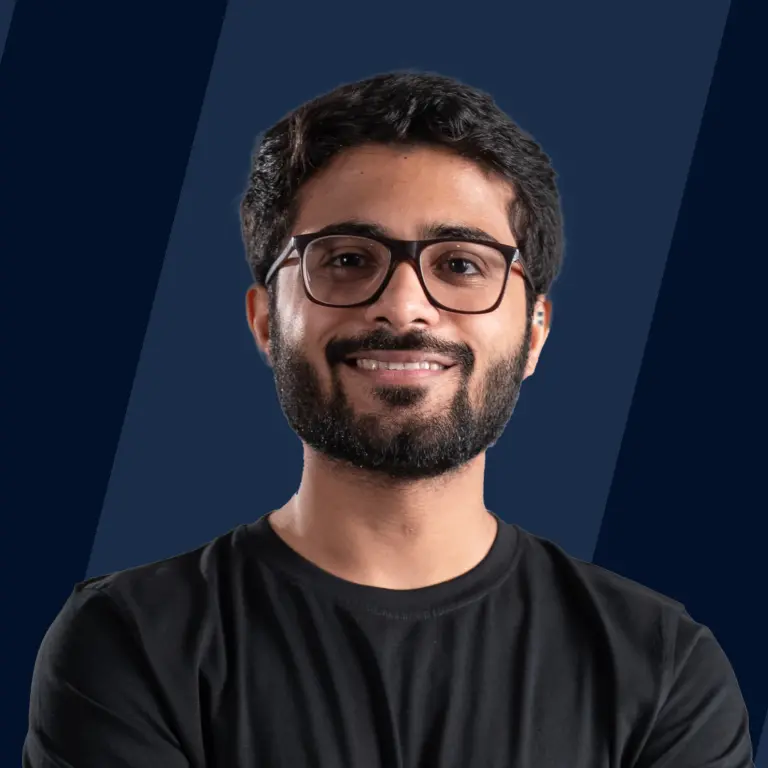
Overview
The String join or the String.join() method in Java is used to concatenate two or more strings with a delimiter. It joins the strings passed as parameters with a delimiter and returns a new string. This was introduced in JDK 1.8. In this article, we will comprehend the String.join() method in Java.
The java.lang.String.join() Method in Java
As programmers, there are often scenarios where you need to concatenate two or more strings. In such a case, we can use the java.lang.String.join method present in the java.lang package.
The String.join in Java is a method used to concatenate two or more strings using a delimiter. Delimiters are characters that mark the beginning and end of two strings. The String.join was introduced in JDK 1.8 and is one of the important string methods. It joins the string and returns a single string.
The String.join in Java is a static method. That means we do not need to create a String object to call this method.
Syntax
There are two ways by which the String.join can be used.
For strings, the following method is used.
For iterable objects (HashSet, ArrayList, LinkedList), the following method is used.
In the above two syntaxes, the ... represents one or more CharSequence. A CharSequence is a readable sequence of char values. It is an interface and some classes including- CharBuffer, StringBuffer, and StringBuilder implement it. It plays an important role when you need to access characters in a sequence without modifying the original data. CharSequence is important in operations like searching, matching, or extracting substrings from a larger text.
Parameters
The String.join in Java takes in two parameters-
- delimiter- it is the first parameter. It is a parameter that separates each element.
- elements- elements to be joined together
Return Types
The String.join in Java returns a new String joined using a delimiter.
Examples
Example 1
We will use - as a delimiter and join the given strings.
Output:
The delimiter here is - which is used to join the rest of the given strings.
Example 2
When we use null as a delimiter, we get the NullPointerException.
Output:
Example 3
In the strings that are supposed to be joined, if some of the elements are null, then null is also concatenated. The NullPointerException is not thrown.
Output:
As there are many strings, null is also treated as a string and concatenated.
Example 4
We will use an iterable to understand the working on the String.join method.
Output:
We have created an integrable-ArrayList. We have added elements to it and then joined it with / delimiter. It works in the same way.
FAQs
Q. What is the purpose of the String join method in Java?
A. The String join method is used to concatenate two or more strings with a specified delimiter and return a single, new string.
Q. What happens if a null delimiter to the String join method?
A. If a null delimiter is passed to the String join method, it will throw NullPointerException.
Q. Do we need to create an object of the String class to use the String.join method?
A. No. The String.join method is a static method. That means we do not need to create a String object to call this method.
Q. How many parameters does the String.join method take?
A. The String.join method takes 2 parameters-
- delimiter- it is the first parameter. It is a parameter that separates each element.
- elements- elements to be joined together
Conclusion
- The String.join() method in Java is used to concatenate two or more strings with a delimiter. It joins the strings passed as parameters with a delimiter and returns a new string.
- The String.join was introduced in JDK 1.8. The String.join method is a static method. That means we do not need to create a String object to call this method.
- It is present in the java.lang package.
- The method takes 2 parameters- delimiter and elements. The delimiter is the first parameter that separates each element and the second onward parameters are the elements to be joined together.
- When we use null as a delimiter, we get the NullPointerException.
- In the strings that are supposed to be joined, if some of the elements are null, then null is also concatenated. The NullPointerException is not thrown.