Convert String to Number in JavaScript
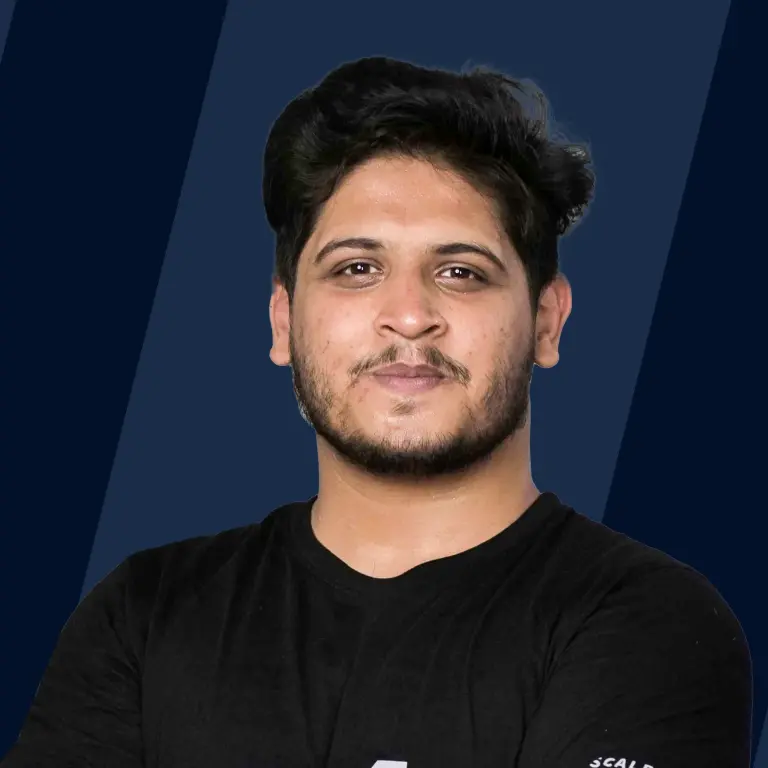
Overview
Javascript provides multiple built-in methods to convert a string value to a number. The concept behind the conversion of string to number is type conversion in Javascript. Various methods provided by Javascript can be used to convert the string into a number only if the string characters are convertible into numbers, otherwise, the NaN value is displayed.
Introduction
In programming, we often encounter situations where we have to perform a set of operations on different kinds of data. Often in such cases, the data type of these sets of data needs to be the same.
For example :
Suppose we have made a percentage calculator website that takes the marks scored and total marks as input and divides them, and multiplies the result by 100.
Now suppose the input value is a string, thus it is not possible to perform division among marks scored and total marks. So, how do we calculate the percentage ?
If we would need to extract the inputs in form of numbers. The Javascript provides the feature to convert string input to a number value. Thus the marks scored and total marks can be converted to numeric values before performing arithmetic operations on them.
How to Convert String to Number in Javascript ?
Javascript provides many built-in methods as well as provides operator support to convert a string to a number in Javascript. The main idea behind this is type conversion in Javascript.
In Javascript, type conversion is the process of converting data of one type to another.
For example :
Output :
Some common methods provided by JavaScript to convert a string to a primitive number areL parseInt(), parseFloat(), Math.floor(), Math.ceil(), Unary Operator / Multiply by 1.
Approaches for the String to Number Javascript
In this section, we will discuss different methods to convert a string into a number in Javascript. Following are some approaches to converting a string to a number in Javascript :
Using the parseInt() Function
A string in a Javascript program can be converted into a number using the parseInt() function. The parseInt function in Javascript takes a string and converts it into an integer value.
Syntax :
Parameters :
The parseInt in Javascript takes two parameters :
- stringval :
The stringVal refers to the input string value that will be converted to an integer. - radix :
The radix is used to represent the base of the number into which the output will be displayed. It contains numbers between 2 and 36. This is an optional parameter and by default, its value is 10 which refers to the decimal system.
Note :
Passing a non-string value as stringVal will lead to SyntaxError: Unexpected identifier.
Example :
In this example, we will convert the string "13.55" to a number using the parseInt function.
Output :
Explanation of the example :
In the above example, we are passing the string str to the parseInt function. Since the value of the radix is not mentioned thus the parseInt, by default will take the value of the radix equal to 10 and display the result in a decimal system. Thus it outputs 13.
Note :
As discussed earlier, the parseInt function returns an integer value, thus the value '13.55' which is a decimal value gets converted into an integer.
Using the parseFloat() Function
In the above case, we can observe that the input string was a decimal value but the output number was an integer. Thus in the cases where the input is decimal, parseInt is not effective. Thus for such cases, we can use parseFloat() in Javascript. The parseFloat returns the decimal output without rounding off values.
A string in a Javascript program can be converted into a number using the parseFloat() function. The parseFloat function in Javascript takes a string and converts it into a decimal value.
Syntax :
Parameters :
The parseFloat in Javascript takes two parameters :
- stringval :
The stringVal refers to the input string value that will be converted to an integer. - radix :
The radix is used to represent the base of the number into which the output will be displayed. It contains numbers between 2 and 36. This is an optional parameter and by default, its value is 10 which refers to the decimal system.
Note :
Passing a non-string value as stringVal will lead to SyntaxError: Unexpected identifier.
Examples :
In this example, we will convert the string "13.55" to a number using the parseFloat function.
Output :
Explanation of the example :
In the above example, we are passing the string str to the parseFloat function. Since the value of the radix is not mentioned thus the parseFloat, by default will take the value of the radix equal to 10 and display the result in a decimal system. Thus it outputs '13.55'.
If the first character of the input string cannot be converted into a number then the parseFloat function returns NaN.
In the above example, suppose the input string is 'M13.55'.
Output :
Then the parseInt will output NaN as the first character of the given string is 'M' which cannot be converted into a number.
Using the Unary Plus Operator (+)
A string in a Javascript program can be converted into a number using the unary operator (+). The unary operator in Javascript is placed followed by the input string and it converts the string input into a number and outputs the result in the decimal system. If the input string is an integer then it returns an integer and if the input string is a float value then it returns a float value.
Syntax :
Parameters :
- stringval :
The stringVal refers to the input string value that will be converted to an integer.
The + value should be prefixed to the stringVal and there should not be any space.
Example :
In this example, we will convert the string "13.55" to a number using the unary operator.
Output :
Explanation of the example :
In the above example, we are using the unary operator along with the string str. Since the type of the number is float thus the unary operator will return a float value. Thus it outputs '13.55'.
If the first character of the input string cannot be converted into a number then the unary operator also returns NaN.
In the above example, suppose the input string is 'Bic13.55'.
Output :
Then the unary operator will output NaN as the first character of the given string is 'B' which cannot be converted into a number.
Multiplying the String by the Number 1
A string in a Javascript program can be converted into a number by multiplying the string with the numeric value 1. The number 1 upon multiplication does the type conversion of the string value into a number and returns the string into a number in the decimal system. If the input string is an integer then it returns an integer and if the input string is a float value then it returns a float value.
Syntax :
Parameters :
- stringval :
The stringVal refers to the input string value that will be converted to an integer.
Example :
In this example, we will convert the string "13.55" to a number by multiplying it by the number 1.
Output :
Explanation of the example :
In the above example, we are multiplying the string str with the number 1. This will do the type conversion of the string. Since the type of the number is float thus the product will return a float value. Thus it outputs '13.55'.
Dividing the String by the Number 1
A string in a Javascript program can be converted into a number by dividing the string with the value 1. The number 1 upon division does the type conversion of the string value into a number and returns the string into a number in the decimal system. If the input string is an integer then it returns an integer and if the input string is a float value then it returns a float value. Using the division we can find the numbers upto three decimal places precisely.
Syntax :
Parameters :
- stringval :
The stringVal refers to the input string value that will be converted to an integer.
Example :
In this example, we will convert the string "13.55" to a number by dividing it by the number 1.
Output :
Explanation of the example :
In the above example, we are dividing the string str by the number 1. This will do the type conversion of the string. Since the type of the number is float thus the division will return a float value. Thus it outputs '13.55'.
Subtracting the Number 0 From the String
Strings can also be converted to a number in Javascript by subtracting the string with the numeric value 0. The number 0 upon subtraction does the type conversion of the string value into a number and returns the string into a number in the decimal system. If the input string is an integer then it returns an integer and if the input string is a float value then it returns a float value.
Syntax :
Parameters :
- stringval :
The stringVal refers to the input string value that will be converted to an integer.
Example :
In this example, we will convert the string "13.55" to a number by subtracting it from the number 0.
Output :
Explanation of the example :
In the above example, we are subtracting the string str by the number 0. This will do the type conversion of the string. Since the type of the number is float thus the division will return a float value. Thus it outputs '13.55'.
Using the Bitwise NOT Operator (~)
A string in a Javascript program can be converted into a number with the help of the bitwise NOT (~) operator. The bits of an operand get inverted and the operand value is converted into a 32-bit signed integer when the bitwise NOT operator is used upon a string.
A 32-bit signed integer is a value that represents an integer in 32 bits (or 4 bytes).
The bitwise NOT operator (~) will invert the bits of an operand and convert that value to a 32-bit signed integer. A 32-bit signed integer is a value that represents an integer in 32 bits (or 4 bytes).
The use of a single bitwise NOT (~) operator upon an operand is equivalent to the operation (-x - 1).
Syntax :
Parameters :
- stringval :
The stringVal refers to the input string value that will be converted to an integer.
Example :
In this example, we will convert the string "13" to a number by using the bitwise NOT operator.
Output :
Explanation of the example :
In the above example, the bitwise NOT operator converts the str into a 32 bits signed integer and returns the value. It performs the operation (-x-1) thus the result is (-13 - 1) = -14.
Using the Math.floor() Function
Math.floor() :
The floor() function of the Math object accepts a floating point number and returns the largest integer less than or equal to the given number. Since floor() is a static method of Math thus in Javascript we always use it as Math.floor(), rather than as a method of a Math object you created.
A string in a Javascript program can also be converted into a number with the Math.floor() function. The Math.floor() function takes the string and rounds off the value present in the string to the immediate integer value lesser than or equal to the given value.
The string upon conversion returns an integer value.
Syntax :
Parameters :
- stringval : The stringVal refers to the input string value that will be converted to an integer.
Example :
In this example, we will convert the string "13.55" to a number by using the Math.floor function.
Output :
Explanation of the example :
In the above example, the Math.floor is passed '13.55' as the parameter. Since the closest integer to 13.55 is 14 thus it converts the value to 13.
Using the Math.round() Function
A string in a Javascript program can also be converted into a number with the Math.round() function. The Math.round() function takes the string and rounds off the value present in the string to the nearest integer. The Math.round() function is used to round a Number To 2 Decimal Places in JavaScript.
The string upon conversion returns an integer value.
Syntax :
Parameters :
- stringval : The stringVal refers to the input string value that will be converted to an integer.
Example :
In this example, we will convert the string "13.55" to a number by using the Math.round function.
Output :
Explanation of the example :
In the above example, the Math.round is passed '13.55' as the parameter. Since the closest integer to 13.55 is 14 thus it converts the value to 14.
JavaScript is the backbone of modern web applications. Enroll in our JavaScript course and master the art of creating dynamic web experiences.
Conclusion
- A string can be converted to a number in Javascript with the use of multiple built-in methods.
- Javascript uses type conversion to convert a string to a number.
- The Javascript provides the following built-in methods : parseInt(), parseFloat(), Math.floor(), Math.ceil(), Unary Operator / Multiply by 1.
- If the given string input has characters that cannot be converted to a number then it returns NaN.