Python String strip() Method
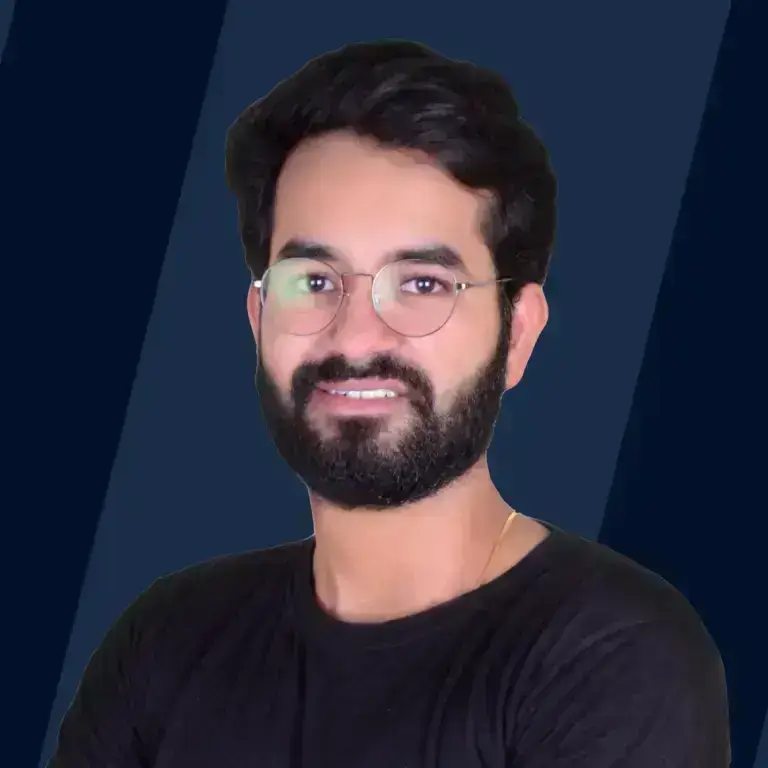
The strip function in Python is an inbuilt function in python that is used for removing a set of characters from a string. But it can only be removed if those characters appear at the start or end of the string.
By default, the strip() function only removes whitespaces from the start and end of the string. The strip() function returns a replication of the input string, and thus, the original string remains unmodified.
Syntax of strip() in Python
The syntax of the strip() in python is as follows:
where myStr is the string on which the strip function is applied and del_string is a parameter.
Parameters the strip() Function in Python
It accepts one parameter,
- del_string, all characters present in the parameter are removed from the start and end of the string. The order of the characters in the parameter does not matter; every character present in del_string is removed irrespective of the order.
This is an optional parameter and can also be left unspecified or set as None.
Return Value of strip() in Python
Return Type: str
The strip() function returns a new string by replacing a few or no characters from the old string according to the specified functionality.
Example of strip() in Python
In this example, we are going to use the strip() function.
Without Parameters
When we don't pass the parameter to the strip function, it removes whitespaces from the start and end. Let's see the working example of this discussion.
Output:
Explanation: As there was no specified parameter that's why only the whitespaces are removed from the start and end of our input string. Also, one point to notice is, the whitespace between Hi and There is not removed because it is inside the string, not at the beginning or end.
With Parameters
Here we will use the function with parameters. We can see that the order of the characters to be removed does not matter. It can also be seen from this example that strip() stops on the first occurrence of a character that is not to be removed or can say not exists in the parameter string.
Output:
Explanation:
The occurrences of '.' are removed only until the first 's' was encountered and the remaining'.' after that 's' is not removed. Similarly from the end, the '.' and 'a' are removed untill the 'd' was encountered.
What is strip() in Python?
Ever wondered how unwanted characters are removed from a string. For example, the unwanted whitespaces are removed if you add whitespaces before your name in a form. Well, this is done using the python strip() function.
Working of the strip() in Python
The python strip() function removes the characters specified in the del_string parameter from the input string.
The strip function works by traversing the input string from the start, removing the character specified in the del_string parameter until a character not specified in del_string is encountered. On encountering such a character, it stops. Then the same procedure is followed from the end of the string, traversing the string in reverse order, and stops on encountering a character not specified in the del_string parameter.
If the parameter is not specified or is set to None, only the whitespaces are removed from the starting and end of the input string.
The strip() function doesn't alter the original string, as strings are immutable in python.
More Examples
Example 1: Strip Function With None
Output:
Explanation: We have passed None as the del_string parameter, due to which only the whitespaces are removed from the start and end of our input string.
Example 2: Original String is not altered
Output:
Explanation:
We can see that the string myStr remains the same after applying the strip() function. This shows that the strip function does not change the original string.
Example 3: Passing Substring as Parameter
We can also directly pass a substring to this parameter, as we know the order of the characters in the del_string parameter does not matter, So the strip() function will remove that substring from the end and beginning.
Output
Explanation: We have directly used the string paramStr as a parameter to the strip() function, and we can see that "one" has been removed from the beginning of the original string.
Check out this article to know more about Substring in Python.
Example 4: Python strip() on Invalid Data Type
The strip() function can only be applied to a string and can accept only a string as a parameter.
- If we apply the strip() function on an invalid data type, we will get an error stating that strip() is not defined for that data type.
Output:
- If we use an invalid data type for the del_string parameter, we get an error stating that strip arg must be None or str.
Output
Example 5: Python Stripping Specific Character with Strip() Function
Output:
Explanation
- The original_string variable contains the string "xxxHello, World!xxx", where "x" is the character we want to remove from both ends.
- The strip() function is used on the original_string with the argument 'x', indicating that all instances of the character "x" should be removed from both the beginning and end of the string. It's important to note that strip() only removes the characters from the ends, not from the middle of the string.
- The result is stored in the stripped_string variable, which now contains "Hello, World!"—the original string without the "x" characters at the beginning and end.
Why Is the Python Strip() Function Used?
The Python strip() function is widely used in text processing and data manipulation for several important reasons.
-
By default, strip() removes leading and trailing whitespace, including spaces, tabs, and newline characters, essential for cleaning up user input or data imported from files.
-
It is instrumental in data preprocessing to clean up data by removing unwanted characters from strings, ensuring consistency and accuracy in data analysis.
-
Ensures uniformity in strings, especially when the data comes from various sources with inconsistent formatting, making it easier to process and analyze.
-
Helps in parsing user input or data from external sources by stripping extraneous characters, preventing errors and simplifying further processing.
-
Removing leading and trailing characters makes string comparison more reliable and straightforward, crucial in many programming contexts like sorting, searching, and matching operations.
Conclusion
- The python strip() function can be used to remove whitespaces from the start and end of a string.
- The caller object of the function and the passed parameter must be a string.
- The python strip() is used to remove any unwanted characters from the start and end of a string.
- The strip() function in python should not be used to remove characters in our required string as it may cause those characters to be deleted.