Subprocess Module in Python
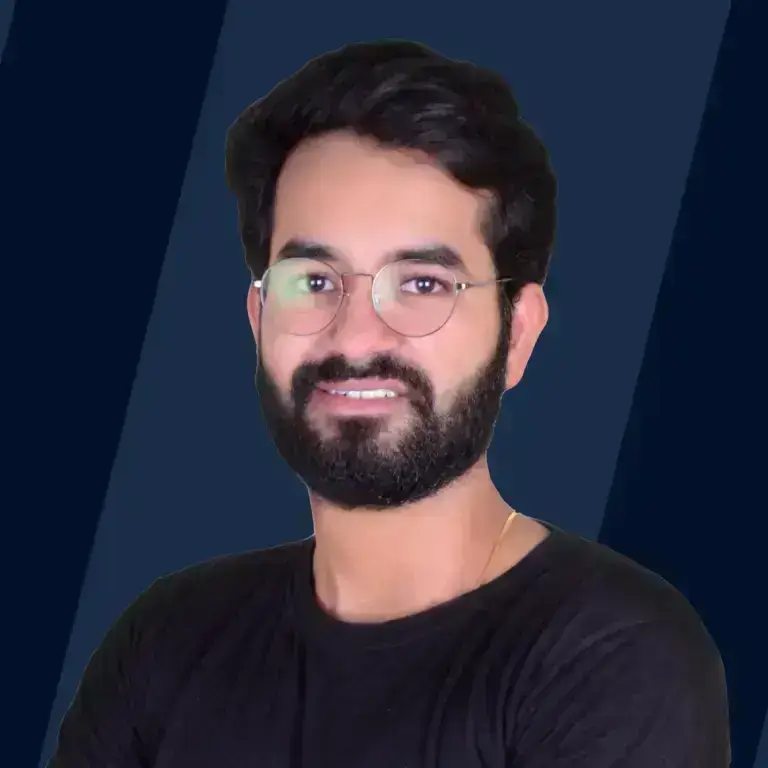
Overview
A running program is called a process. Each process has its system state. A program can create new processes using library functions. However, the subprocesses are independent and run with their private system state and the main thread of execution.
The subprocess module in Python is for producing child processes. When we use a subprocess, Python is the parent that creates a new child process. Hence the name is sub-process. The subprocess module in Python allows us for running external programs and reading their outputs or results in our Python code.
What is Subprocess Module in Python?
Let us look into what is the subprocess module in Python.
Introduction
The subprocess module in Python is meant for producing the child processes. These processes might be anything from shell scripts to graphical user interfaces. The sub in the subprocess name refers to the parent-child connection of processes. Python is the parent process that generates a new child process when we are using the subprocess. And we are responsible for deciding what that new child procedure is.
The Subprocess module is used to run new programmes and scripts by launching new processes. It enables you to launch new applications directly from a Python program that you are building. So, you may use a subprocess in Python to run external applications from a git repository or code from C or C++ programmes. Using subprocess in Python, you can also obtain exit codes and input, output, or error streams.
Use of Subprocess to Run Any Application
Let us finally learn a few processes through which we can utilize the subprocess module to run any application in Python.
Running an External Program We can utilize the subprocess.run function to run any external program from our python code. To do that, we need to perform the below steps:
- Import the subprocess module
- import the sys modules into your code
Code :
Output:
Let us break down our code to understand each parameter:
-
sys.executable: It is the absolute path of the Python executable, from where our code was invoked from. For example, it could be a path like /usr/local/bin/python.
-
subprocess.run: It contains the parts of the command we are trying to run. In this example, since we pass the first string as sys.executable, we are asking our subprocess.run to execute a new Python program.
-
-c: It allows us to pass a string with an entire Python program to execute. Here we pass our print statement.
We can say that, [sys.executable, "-c", "print('Hey Pythonista :P')"] translates roughly to /usr/local/bin/python -c "print('Hey Pythonista :P')"
Exceptions to Subprocess Module in Python
Let us discuss some exceptions that can be raised in the subprocess module in Python.
1. exception subprocess.SubprocessError It is the base class for all the exceptions from the Subprocess Module in Python.
2. exception subprocess.TimeoutExpired It is the subclass of SubprocessError, and is raised whenever a timeout expires while waiting for a child process.
3. exception subprocess.CalledProcessError It is the subclass of SubprocessError, and it is raised when a process run by check_call(), check_output(), or run()(with check=True) returns a non-zero exit status.
Catching Exception Output from the subprocess.check_call() :
We have already learned about the subprocess.check_call() module previously in this article. Now exceptions can raise out of this command, and to catch them, we can follow the below steps:
-
Firstly, run the subprocess.check_call() commands with the given arguments.
-
If the return code from the command is zero, then simply return.
-
Otherwise, raise the CalledProcessError. We can get the return code from the CalledProcessError object from its returncode attribute.
-
In case, check_call() is not able to start the process, it will propagate the exception that was raised.
Basic Usage of the Subprocess Module in Python
There are multiple use cases of the subprocess module in Python. Let us look into a few of them below --
-
The subprocess module is included in the Python 3 and above versions. It is used for running external programs and then reading out their outputs into our Python code.
-
We can also use the subprocess module in scenarios when we want to use another program in our system from within our Python code.
There are other uses as well but we will cover all these in detail once we have learned about the syntax and basic format of the Subprocess Module in Python ahead in the article.
Syntax of the Subprocess Module in Python
Before using the subprocess module in Python, we need to import it. Since it comes inbuilt with Python, we do not need to install this module.
Syntax:
The run() function will be the main connection through which we will communicate with the Python subprocess module. As run() can handle most of the scenarios, the documentation suggests using it. The Popen class is useful for edge scenarios where you need greater control.
A very detailed explanation of each of the parameters the subprocess module takes and their usage can be found here in the
Official Python documentation.
Replaceable Functions Through Subprocess Module in Python
With the help of the subprocess module, we can:
- start new processes
- connect to their input, output, or error pipes
- and get their return codes.
In comparison to some of the other modules, it provides a higher-level interface and is designed to take the role of the below specific functions:
- os.system()
- os.spawn*()
- os.popeopen
- popen2.*()
- commands.*()
Let us now discuss in detail how can the subprocess module replace the above-mentioned functions.
One thing you would know is that UNIX commands cannot be used in a Python script in the same way that Python code can. For example, if we use the echo command in our Python code, we will surely get a syntax error, obviously because echo is not a statement or function that comes with Python. And we use the print command instead. Hence to run the UNIX commands in Python, we need to create a subprocess that runs the command.
os.system()
The os.system() mainly passes the command and arguments to our system's shell. We can run multiple commands at once by using it and set up pipes and input or output redirections.
Replacing os.system() In the following examples, we assume that the subprocess module is imported with “ from subprocess import *”. So let us look at how it has replaced the above function:
os.popen()
The os.popen() command has been deprecated since version 2.6 and the documentation says -- This function is obsolete. Use the subprocess module. docs.python.org. So let us learn about the subprocess.call() which has replaced this command.
subprocess.call()
The subprocess. the call is just like the Popen class. The call() method is used to initiate a program. Let us look at the syntax below:
Syntax :
The following parameters are accepted by Python's call() method from the subprocess:
-
args: This refers to the command you want to run. By using a semicolon to separate them, you can pass numerous commands (;)
-
stdin: This denotes the value of the standard input stream sent by (os.pipe()).
-
stdout: It represents the result in the standard output stream.
-
Stderr: This manages any errors from the standard error stream that happened.
-
shell: If true, this boolean parameter causes the programme to run in a new shell.
The Python subprocess call() function generally returns the executed code of the program. But in case there is no program output, then the function returns the code itself that is executed successfully.
os.spawn
The os.spawn is also replaced by the subprocess module in Python. Let us look into all the os.spawn functions on how they have been replaced with the subprocess module in Python.
Replacing the os.spawn family
The below examples are directly taken from the Python official Documentation. Let us look into each of them:
1. P_NOWAIT example:
2. P_WAIT example:
3. Vector example:
4. Environment example:
Replacing os.popen(), os.popen2(), os.popen3()
os.popen()
os.popen2()
os.popen3()
os.popen4()
Replacing Functions from the popen2 Module
Let us look into the examples from documentation where we replace the popen2 module from Python's subprocess module.
Code 1:
Code 2:
Please note that:
popen2.Popen3 and popen2.Popen4 work as same as subprocess.Popen, except for a few points:
- Popen raises an exception if the execution fails.
- The capturestderr argument is replaced with the stderr argument.
- Here the stdin=PIPE and stdout=PIPE must be mentioned.
- All the file descriptors are closed by default in popen2, but in the case of Popen, we have to specify the close_fds=True explicitly.
Commands.*()
There are certain commands that are replaced by Python's subprocess module. Let us learn about them below:
Replacing /bin/sh shell backquote
Replacing shell pipeline
Types of Subprocess Modules in Python
Let us look into the types of subprocess module in Python.
subprocess.check_call()
The Subprocess module in Python has a call() method that is used to initiate a program.
Below given is the syntax of the subprocess check_call():
Parameters:
The following parameters are accepted by Python's call() method from the subprocess:
-
args: This refers to the command you want to run. By using a semicolon to separate them, you can pass numerous commands (;)
-
stdin: This denotes the value of the standard input stream sent by (os.pipe()).
-
stdout: It represents the result in the standard output stream.
-
stderr: This manages any errors from the standard error stream that happened.
-
shell: If true, this boolean parameter causes the programme to run in a new shell.
Return Value:
The Python subprocess call() function generally returns the executed code of the program. But in case there is no program output, then the function returns the code itself that is executed successfully.
Also, it can raise a CalledProcessError exception occasionally.
Save Process Output (stdout) in Subprocess in Python - subprocess.check_output()
With the help of the check output function, the save process output or stdout enables us to immediately store the output of a code in a string.
Below given is the syntax for this method :
Parameters:
The following parameters are accepted by Python's subprocess.check_output() from the subprocess module:
-
args: This refers to the command you want to run. By using a semicolon to separate them, you can pass numerous commands (;)
-
stdin: This denotes the value of the standard input stream sent by (os.pipe()).
-
stdout: It represents the result in the standard output stream.
-
stderr: This manages any errors from the standard error stream that happened.
-
shell: If set true, this boolean parameter causes the programme to run in a new shell.
-
universal_newlines: When set to true, the boolean argument universal_newlines opens the files with a universal newline for stderr and stdout.
Return Value: The Python subprocess.check_output() function generally returns the same output as the subprocess call(). It outputs the executed code of the program. But in case there is no program output, then the function returns the code itself that is executed successfully.
It can also raise a CalledProcessError exception occasionally.
Examples of Subprocess Module
In this example, we will see the Python subprocess module to execute programs written in different languages such as C, C++ and Java. Let us look into our example below --
C Program:
C++ Program
Java Program:
Let us write the Python code to execute the above codes.
Python Code:
output:
Conclusion
In this article, we discussed the Subprocess Module in Python. Let us summarise what we learned till now.
-
Subprocess module in Python is used to run new programmes and scripts by launching new processes. It enables you to launch new applications directly from a Python program that you are building.
-
It is used for running external programs and then reading out their outputs into our python code.
-
Subprocess module in Python has replaced functions like os.system(), os.spawn*(), os.popen*(), popen2.*()
-
The Subprocess module in Python has a call() method that is used to initiate a program.
-
The subprocess.check_output() enables us to immediately store the output of a code in a string.
-
We can utilise the subprocess.run function to run any external program from our Python code.