Sum of Digits of a Number in Python
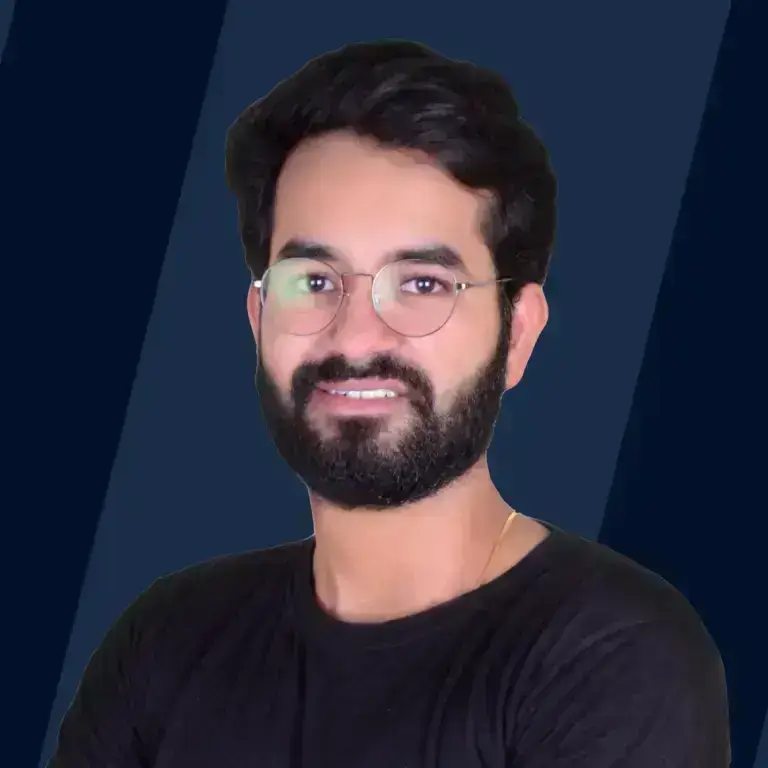
Overview
The article is about different ways to find the sum of digits of a number in Python. We can calculate the sum of digits of a number by adding a number's digits while ignoring the place values. So, if we have the number 567, we can calculate the digit sum as 5 + 6 + 7, which equals 18.
Introduction to Sum of Digits of a Number
The goal is to determine the sum of digits of a number given as an input in Python. Example:
Input:
Output:
Input:
Output:
We begin by dividing the number into digits and then adding all of the digits to the sum variable. To break down the string, we use the following operators:
The modulo operator % is used to extract the digits from a number. After removing the digit, we apply the divide operator to shorten the number.
Different Methods to Find Sum of Digits of a Number in Python
Using str() and int() methods
To convert a number to a string, use the str() function. To convert a string digit to an integer, use the int() function.
Convert the number to a string, iterate over each digit in the string, and add to the sum of the digits in each iteration.
Algorithm Flow:
-
Step 1: Gather user input.
-
Step 2: Create a variable to hold the result.
-
Step 3: Convert the number to a string.
-
Step 4: Write a loop for each digit in a number.
-
Step 5: Convert the digit to an integer and add it to the sum.
-
Step 6: Invoke the function and print the result.
Code:
Output:
Using iteration
We shall use loops to calculate the sum of digits of a number. Loops are used to execute a specific piece of code continually. Some looping statements are for loop, while, and do-while.
To find the rightmost digit of an integer, divide the integer by 10 until it equals 0. Finally, the remaining will be the rightmost digit. Use the remaining operator " percent " to receive the reminder. Divide the obtained quotient by 10 to get all the digits of a number. To find the number's quotient, we use “//”.
Algorithm Flow:
-
Step 1: Create a function for finding the sum using the parameter n.
-
Step 2: Declare a variable sum to store the digit sum.
-
Step 3: Create a loop that will run until n is greater than zero.
-
Step 4: To the remainder returned by, add the sum variable (n percent 10)
-
Step 5: Change n to n/10.
-
Step 6: Collect feedback from the user.
-
Step 7: Invoke the function defined earlier and pass the input as an argument.
-
Step 8: Print the sum of the values returned by the function.
Code:
Output:
Conclusion
- A sum of digits of a number in python in a given number base is the sum of all its digits. For example, the digit sum of the decimal number 9045 would be 9+0+4+5=18.
- sum of digits of a number in Python can be calculated in many ways, such as using inbuilt functions, iteration, and recursion, as discussed earlier.