swap() in C++
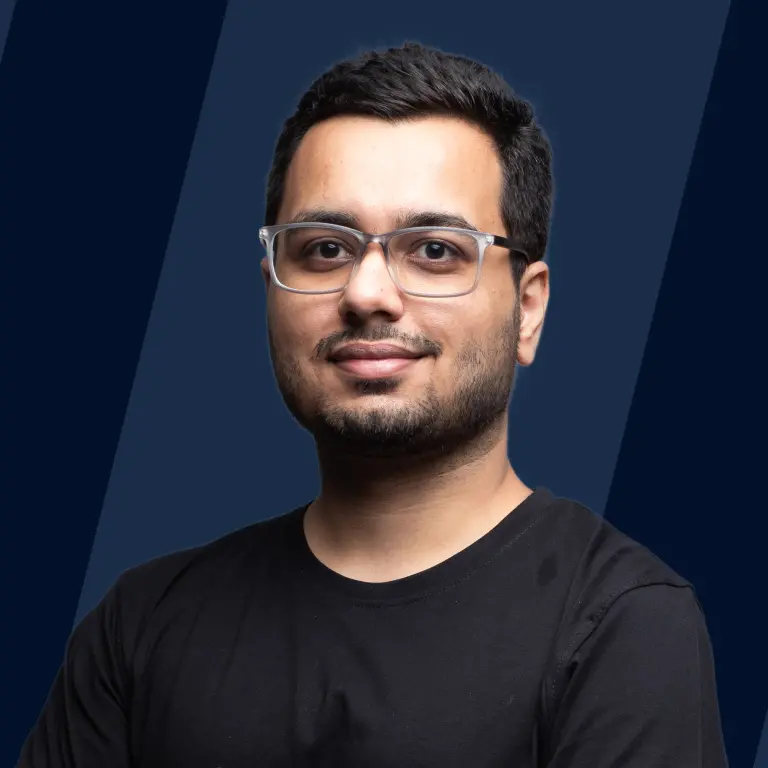
Overview
The swap C++ function is a simple and useful function used to swap the values stored in variables. Using the swap C++ function, we can swap the value stored in two variables without using a third or temporary variable. Let us learn more about the swap C++ function and its implementation.
Syntax of swap() in C++
The syntax of the swap() in C++ is as follows:
Parameters of swap() in C++
Now that we know the syntax of the swap() function in C++ Let's look at the parameters passed to the swap() function in C++.
- a - The variable whose value needs to be swapped with the value of variable b.
- b - The variable whose value needs to be swapped with the value of variable a.
Return Value of swap() in C++
The Return Value of the swap function is nothing. Therefore the swap C++ function has a void return type.
Exceptions of swap() in C++
The swap function in C++ works in most cases and has no exceptions.
Complexity
The swap function in C++ has constant complexity. If the swap function is used on an array of n elements, then the swap function has the complexity of n.
Examples
Now we know the syntax of swap() in C++. Let us look at some examples and understand how to use the swap() function in our programs.
Using std::swap() with variables
code
output
In the above code, The swap function swaps the value stored in variables a and b.
Using std::swap() with Arrays
output
In the above code, The swap function swaps the value stored in the array a and b.
Using std::swap() with Characters
output
In the above code, The swap function swaps the char value stored in the array a and b.
Using std::swap() with String
code
output
In the above code, The swap function swaps the value stored in the string a and b.
How to Swap Two Variables in C++ without Using the std
Swapping two numbers using a temporary variable
output
In the above code, We created a temporary variable temp to swap the values stored in the variables a and b.
You can learn more about swapping two numbers in the Swapping of Two Numbers in Java article.
Swapping two numbers using an arithmetic operation
output
In the above code, We used a basic arithmetic operation to swap the value stored in two variables, a and b.
How Does std::swap() in C++ Work?
The swap C++ function is used to swap the value of two variables. Using the swap C++ function, we need not use a third temporary variable or the Arithmetic Operators method to swap the value stored in two variables. The swap C++ function takes two variables as it's a parameter. The swap C++ function swaps the values stored in the variables, passed as the parameter. The swap C++ function swaps the variable's value and doesn't return anything hence swap C++ function has a void return type.
Conclusion
- The swap C++ function swaps the values stored in two variables.
- The syntax of the swap function is swap(a,b).
- The swap C++ function takes two variables as parameters.
- The value of the variables passed as the parameter will be swapped.
- The swap C++ swaps the value of the variables passed as the parameter.