JavaScript String toUpperCase() Method
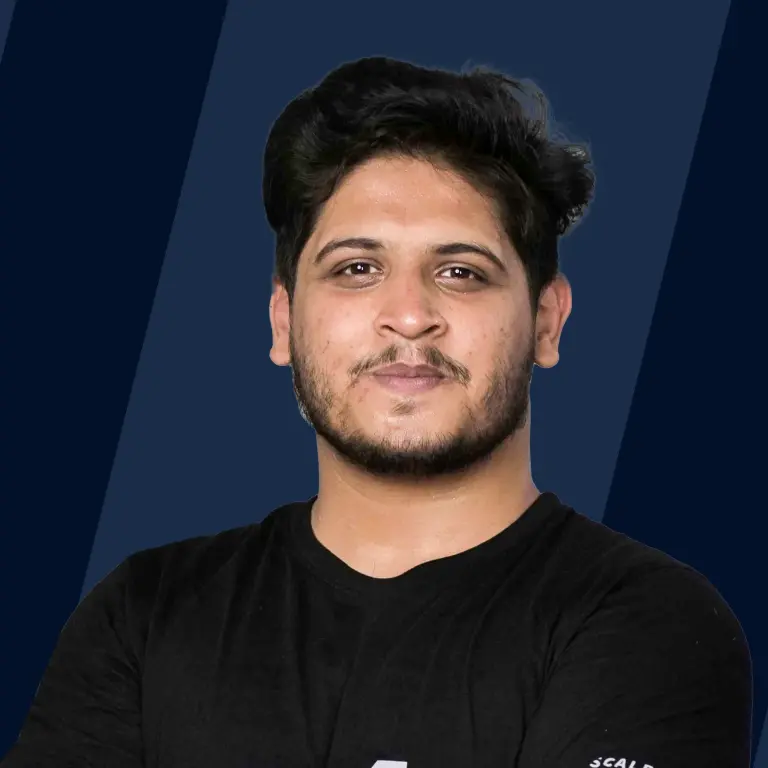
Overview
The toUpperCase() method in JavaScript can be used to convert a string into uppercase characters. This method converts every lowercase character to uppercase and does not alter any other character. The toUpperCase method returns a new string and doesn't modify the original one.
Syntax of JavaScript String toUpperCase() Method
Let's look into the syntax of toUpperCase() JavaScript method():
- str should be a string object.
Parameters of JavaScript String toUpperCase() Method
There are no parameters for the toUpperCase() method. User just call touppercase javascript using required string.
Return Value of JavaScript String toUpperCase() Method
Return value of JavaScript string toUpperCase() method is a string. The toUpperCase() method returns a new string with all upper-case characters transformed to lower-case characters.
Exceptions of JavaScript String toUpperCase() Method
- When used on null or undefined, the toUpperCase() function throws a TypeError.
Example
Let's see a basic example to implement touppercase javascript function:
Output:
Explanation: In the above example, the method toUpperCase() converts all lower case characters to upper case characters equivalents. Use this JavaScript Compiler to compile your code.
What is String toUpperCase() Method in JavaScript?
In JavaScript, the toUpperCase() is a built-in string function. we use the toUpperCase() method to convert the string's lowercase characters to uppercase. Since JavaScript strings are immutable, the toUpperCase() method has no effect on the value of the string. String toUpperCase() method only works with the string data type and it will throw a TypeError if called by any null or undefined object..
More Examples
Example1: Conversion of Non-String Values to Strings
If we call toUppercase() with an object other than string that it will give an error. To ensure that these scenarios work as expected and result in useful outcomes, we should convert the non-string value to a string value to avoid getting errors from touppercase javascript method.
Output:
we convert the non-string variable z to string variable then convert it into uppercase by using touppercase javascript function.
Example2: Conversion of only the First Letter of a String
Three steps are necessary to change a string's first letter to an equivalent uppercase value:
Step 1. Find the first letter and specifically call toUpperCase() on it.
Step 2. Remove the initial character from the string's remaining characters to create a new string without it.
Step 3. The two strings should be combined.
Output:
- firstly, Utilizing the index of the string's initial character (using the substring notation [0]) and capitalizing it by using toUpperCase() makes the first half of this task simple.
- Secondly, To create a string that excludes the first character, we use the substring() method.
- Finally, use the + operator to simply concatenate the two strings together and got the first letter capital by using touppercase javascript function.
Example3: Conversion of First Letter of Every Word in a String
Let's use the following string as our example:
Follow these procedures to capitalise each word in this string:
Step 1. Start by separating each word.
The divide technique allows us to accomplish this task. split() method divides a string object based on a supplied character. In this instance, we specify the value to conduct the split on as the character representing a blank space (' ').
In addition to their special characters and numerals, this will return an array containing every word in our original string. In essence, any portion of the string that is separated by a space character will have its own entry in the array.
Output:
Step 2. Go over the array iteratively.
The following step requires iterating through the array and handling each string separately. There are many ways to accomplish it; in the example below, the map() method is used which do the provided operation to each element of array and return a new array accordingly.
Step 3. Capitalize the first character of each word.
To capitalize the string's first character, grab the first character of each string and use touppercase javascript method. Then, combine the characters using the remainder of the string pulled from the substring() method.
Output:
Step 4. Recombine the array into a single string to complete the process.
The array of substrings must be put back together in the final step, and the space characters that removed by the split() method must be reinserted. The join() technique is used to do this. The join() method combines the array's contents back into a single string. We use the empty space character (' ') as a parameter to retrieve our sentence structure:
Output:
Supported Browser
Browser | Version |
---|---|
Chrome | 1 and higher |
Edge | 12 and higher |
Firefox | 1 and higher |
Internet Explorer | 3 and higher |
Opera | 3 and higher |
Safari | 1 and higher |
Looking to build a solid foundation in front-end development? Our JavaScript certification course is your gateway to success. Sign up today!
Conclusion
- The toUpperCase() method in JavaScript is used to convert string lowercase characters to uppercase format.
- toUpperCase() method returns a new string and does not alter the original string in any way.
- To convert an entire string or just the first character of each word in a string to uppercase, use the JavaScript toUpperCase() method.
- We should call toUppercase() only with string type objects or objects converted to string type otherwise it will throw an error.