What are the Types of Array in C?
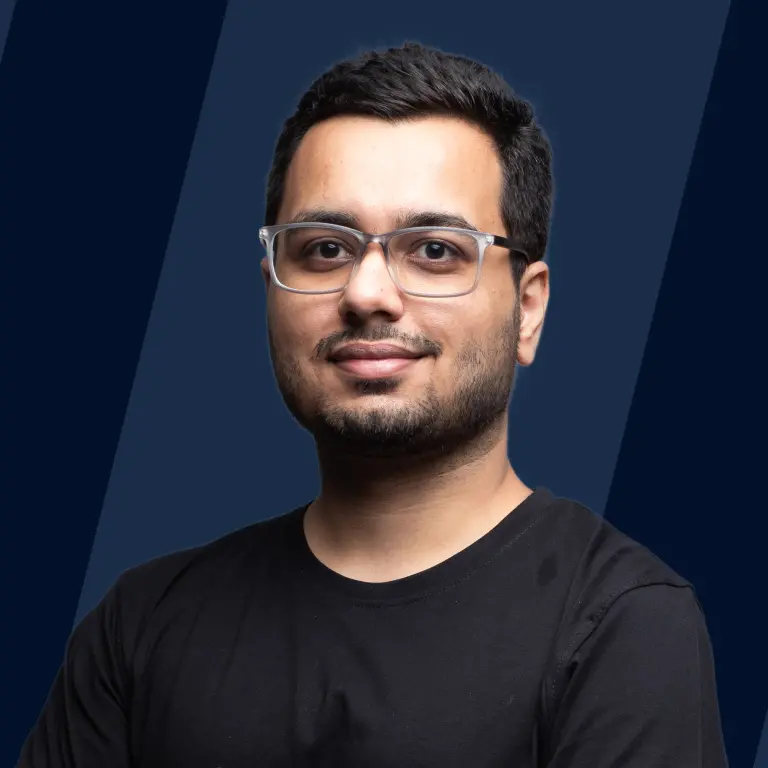
Arrays are classified into two types based on their dimensions : single-dimensional and multi-dimensional. Logically, a single-dimensional array represents a linear collection of data, and a two-dimensional array represents a mathematical matrix. Similarly, a multidimensional array has multiple dimensions.
All elements in the memory are stored in a linear order; it's simply a concept called memory addressing that allows us to access the array dimension-wise.
Single Dimensional Array
The single-dimensional array is one of the most used types of the array in C. It is a linear collection of similar types of data, and the allocated memory for all data blocks in the single-dimensional array remains consecutive.
Syntax for Declaration of Single Dimensional Array
Below is the syntax to declare the single-dimensional array.
- data_type : is a type of data of each array block.
- array_name : is the name of the array using which we can refer to it.
- array_size : is the number of blocks of memory array going to have.
Initialization of Single Dimensional Array
We can initialize an array by providing a list of elements separated by commas and enclosed by curly braces.
Important Point: What if we provide fewer elements than the size of the array?
In this scenario, the rest of the memory block will be filled with the default value, which is null for string array, 0 for integer array, etc. See the example below for further illustration.
Output :
Arrangement
Accessing Elements of Single Dimensional Array
We can access the element of the single-dimensional array by providing the index of the element with the array name. The index of the array starts with zero.
Example
C Program to Enter 5 Numbers and Print them in Reverse Order.
Output :
Explanation :
- First of all, we have created an array of size 5 with some initialized values.
- Later, we are traversing on that array from the last index to the first index, which is 0.
Note :
-
The last index of an array is array length - 1. Beginners make the mistake of considering the last index as 5 if the array size is 5; that's why it is an important point as a takeaway.
-
Inside the loop, we have only one statement, and we are printing the element at index.
To learn more about one-dimensional arrays follow this link.
Multi-Dimensional Array
Multidimensional arrays are an advanced version of a single-dimensional array that can be nested up to multilevel. From another perspective, you can think about it as, an array of an array with one less dimension i.e. an array of a one-dimensional array is a two-dimensional array or an array of a two-dimensional array is a three-dimensional array.
Syntax for Declaration of Multi-Dimensional Array
The syntax of a multidimensional array is only a little bit different from the single-dimensional array.
Two-Dimensional Array
A two-dimensional array is a specialized form of a multidimensional array which have two dimensions. It is widely used among all types of arrays in C programming to represent a matrix in a data structure.
Syntax and Declaration of Two Dimensional Array
array2d is an int type array having 5 rows and 10 columns.
Initialization of Two-Dimensional Array
A two-dimensional array can be initialized by providing a list of the single-dimensional arrays in proper syntax,
Arrangement
Accessing Individual Elements of Two-Dimensional Array
The individual element can be accessed by providing both row and column index of the array,
Passing the Entire 2D Array
There exist different techniques to pass the several types of arrays in C to a function, a few of them are enumerated below, A 2D array can be passed to a function using the following techniques,
1. When Row and Column Both Sizes are Globally Available
Note : We can also skip the row size in the function parameter.
Output :
Explanation :
- In this example, everything is pretty simple, like we are initializing an array and then looping on it to print the elements.
- One thing which requires attention is how we are passing the array to function.
- The rows and columns of the array are available globally and we are passing the array name during the function call.
2. When Column Size is Globally Available
We can omit the Row Size while receiving the parameter in the function definition.
Output :
Explanation :
- We can omit the Row Size while receiving the parameter in the function definition, but the column size is mandatory.
- But still, we have passed the row as a second parameter so that we can get to know about the rows in the array inside the function.
- In this way, we can create a function in which there is no need to globally specify the rows of a two-dimensional array.
3. Using Pointer Approach
In the function definition, a pointer can be used to access the memory of the passed array during the function call.
Output :
Explanation :
- In this example, we are passing the pointer to the array during the function call.
- That pointer will contain the base address of the array and can be used to access the entire array.
- Inside the printf statement we are doing some calculations to find the proper index. We are just finding all addresses by incrementing in the base address.
- This is the best way to pass an array to the function. Because there is no dependency on the size of the array while defining function.
Passing 2D Array as Parameters to Functions
Passing a Row
The function definition can accept a single-dimensional array, and we can provide the array by accessing the row during the function call.
Output :
Explanation :
- In this example, we have passed the single-dimensional array by accessing a particular index of a two-dimensional array.
- So, in the function definition, we can simply accept a single-dimensional array.
Passing Individual Elements
We can simply access the individual elements of the 2D array and give it to the function call.
Output :
Explanation :
- We are accessing an element with the proper indexing and then passing it to a function.
- The function is receiving an int variable, which is being printed later.
Example
C Program to find the sum of all the elements of a matrix.
Output :
Explanation :
- Here initially, we have initialized a two-dimensional array.
- Up next we are looping with nested for loops, where the outer loop is running on rows, and the inner loop is for columns.
- We are accessing the matrix/2d Array with the column and row index.
- Subsequently, there is a sum variable that is used here to accumulate the sum of all elements of a given two-dimensional array.
Three-Dimensional Array
Three-dimensional arrays are also a specialized form of a multidimensional array, generally, they are used to represent three-dimensional coordinates in programming. We could also say that three-dimensional arrays are an array of two-dimensional arrays.
Syntax for Declaration of Three Dimensional Array
Example
C program to show the concept of a three-dimensional array.
Input :
Output :
Representation :
Conclusion
-
If we analyze through the dimension perspective, there are two types of the array in C : Single Dimensional and Multidimensional.
-
Most of the time, we use, one dimensional, two dimensional, and three dimensional arrays from all possible types of the array in c.
-
Generally, a one-dimensional array is used to store the linear collection of data, a two-dimensional array is used to represent matrices and a three-dimensional array is used to represent the coordinates.
-
While working with functions and commonly used types of the array in c, we could pass a whole array or its single element as a parameter.
-
The methods to pass a single-dimensional array to function are enumerated below.
- Passing a fixed size array by providing the size of the array as global.
- Omitting the size of the array by providing only blank brackets []
- Using the pointer to store the base address of the array, later we can use this pointer in the function.