What is var in JavaScript?
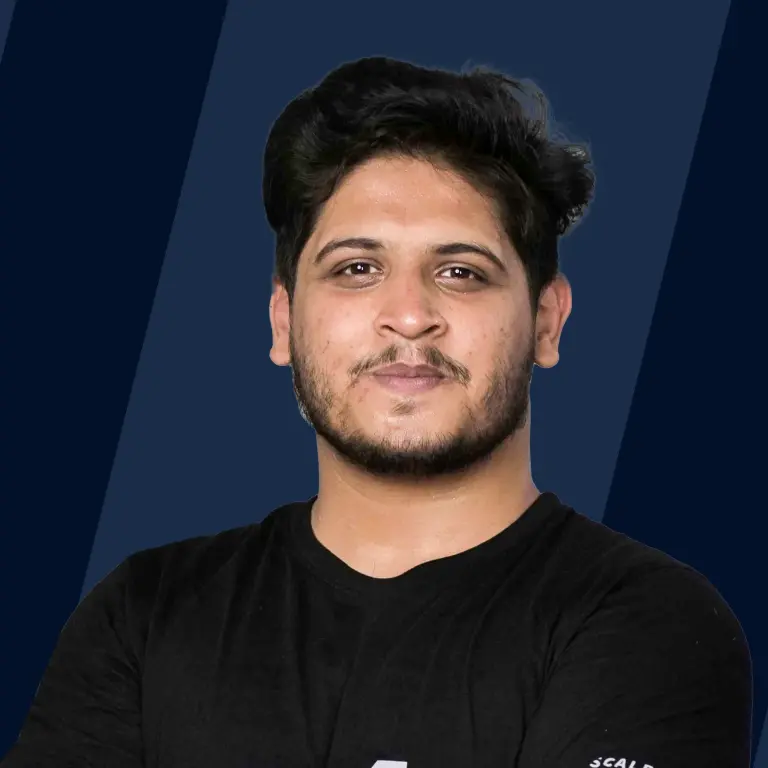
The var in JavaScript is used to store information; it can take any value for example integer, float, or string. We call it a declaration of a variable when the var is created.
The value stored in var is empty after the declaration. When we store any value in var, it is called initializing the value for var in JavaScript.
Syntax
The syntax of var in JavaScript looks something like this.
To assign a value, we use the "=" sign.
- name = The name given to the variable.
- value = The value assigned to the variable that can be anything.
var has No Block Scope
The var has a global scope which means that even if it is declared in a block, the variable can be accessed outside the block too.
For example:
Output :
The result is accurate because the value of var can be accessed outside the block. The var in JavaScript is globally scoped.
In the types of variable declaration, we have,
- let
- var
- const
Where var is globally scoped while let and const are locally scoped, which means we cannot access the variable declared using let outside the block.
Unqualified Identifier Assignments
The global variable is assigned at the top of the scope, which means the variable can be accessed at every scope where we don't have to qualify the names with globalThis. or window. or global..
For example, let's make a function where we will use an unqualified String where it is not necessary to type globalThis.String.
Today, ECMA5Script is set as the strict mode, which means there will be a Reference Error if we use assignment to the unqualified identifier. It is done to prevent other properties from being added to the global object.
Example :
NOTE: It should be noted that there are no implicit or undeclared variables in JavaScript.
var Hoisting
As we have already seen that var is globally scoped, which means we can declare var anywhere in the code, not necessarily on the top.
Using the variable before it is declared is called hoisting.
Example :
Output :
We have assigned the value to a variable without the variable declaration at the global top.
It is recommended to declare variables at the global top so it is easy to identify if the variable is globally scoped or function scoped (local).
Until the assignment of the variable is done the value of the variable is undefined that is given only in the case of var, in the case of let and const the default value before initialization is given in the temporal dead zone.
We should keep in mind that only a var declaration can be made anywhere, not its initialization. We can initialize only when the assignment is reached.
Examples
Some examples on var in JavaScript.
1. Declaring and Initializing Two Variables
We can declare multiple variables using the var function and initialize the values to them, as shown in the following code.
Output :
In the result, both the initialized values to the variables, a and b, are shown in the console.
2. Assigning Two Variables with a Single String Value
Let us take an example where we will assign a single string value to two different variables and get that string in the console for both variables using var.
Output :
The string value of x is assigned to y.
3. Initialization of Several Variables
In the following example, we will see how a globally scoped variable helps in declaring a locally scoped variable outside the function.
We will declare a global variable a and create a local variable inside a function. We will assign the value of the local variable to a and access it outside the function.
Output :
The above code is executed in non-strict mode. That is why there is no reference error thrown. If the code is executed in strict mode it will throw a reference error that means variable b cannot be leaked outside the function.
4. Implicit Globals and Outer Function Scope
In the following example, we will talk about implicit globals, which means declaring variables and accessing them outside the function. We will create three different variables where variable1 is a global variable, and variable2 is declared inside a function f1; therefore, it is local to a function. While variable3 is a global variable created inside function f2.
Output :
We can see that when we try to access the value of variable2, we see an error stating variable2 is not defined. In contrast, we see the values of variable1 and variable3 displayed in the console.
Conclusion
- The var is a type of variable declaration which is used to store information of any kind.
- The value stored in var can be accessed even outside the block, which means it is globally scoped while let (the type of variable declaration) is locally scoped.
- The var takes two parameters: first is the name that we want to give to the variable, and second is the value that we want to give to the variable name.