Virtual Base Class in C++
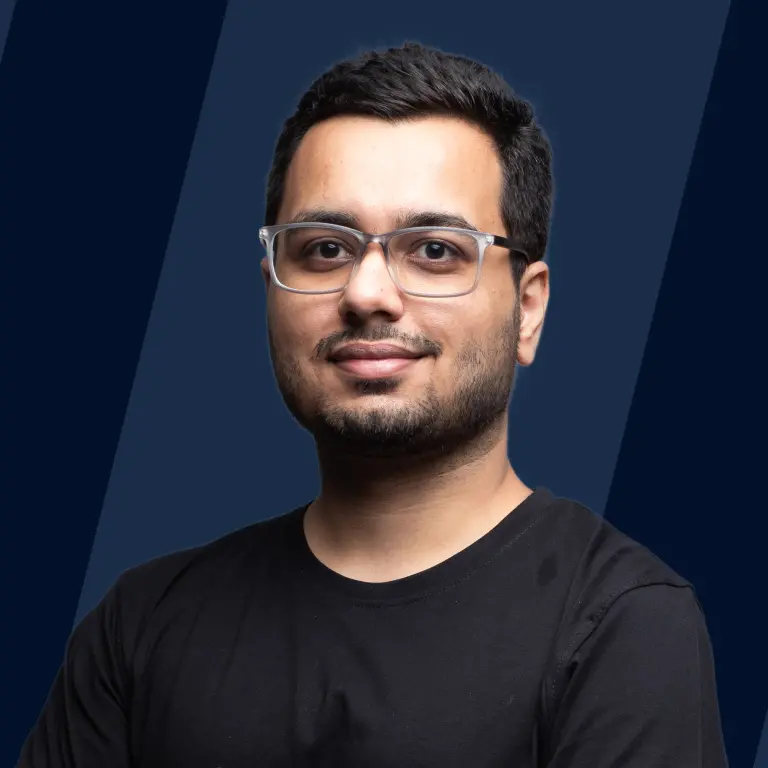
Overview
Virtual base classes in C++ are used to prevent multiple instances of a given class from appearing in an inheritance hierarchy when using multiple inheritances.
Introduction to Virtual Base Class in C++
Before getting into virtual base classes, let us revise the base class and inheritance concepts.
Base classes are the classes from which other classes are derived. The derived(child) classes have access to the variables and methods/functions of a base(parent) class. The entire structure is known as the inheritance hierarchy.
Let us consider the above image, Class A is the parent class, and Classes B and C are the derived classes from Class A. Thus Class B and Class C have all the properties of Class A.
Next, Class D inherits Class B and Class C. With our knowledge, Class D will get all the properties from Class B and C, which also has Class A properties. What do you think will happen when we try to access Class A’s properties through D? There will be an error because Class D will get Class A’s properties twice, and the compiler can’t decide what to output. You’ll see the term “ambiguous” when such a situation occurs.
What is Virtual Class?
Virtual Class is defined by writing a keyword “virtual” in the derived classes, allowing only one copy of data to be copied to Class B and Class C (referring to the above example). It prevents multiple instances of a class appearing as a parent class in the inheritance hierarchy when multiple inheritances are used.
Need for Virtual Base Class in C++
To prevent the error and let the compiler work efficiently, we’ve to use a virtual base class when multiple inheritances occur. It saves space and avoids ambiguity.
When a class is specified as a virtual base class, it prevents duplication of its data members. Only one copy of its data members is shared by all the base classes that use the virtual base class.
If a virtual base class is not used, all the derived classes will get duplicated data members. In this case, the compiler cannot decide which one to execute.
Let us look at an example without a virtual base class in C++ and see what the output looks like:
Output:
In the above example, we create a Class A and then two of its derived classes, Class B and Class C. Class A has a method that prints out a statement. All the derived classes must have inherited data members from Class A.
Next, we declare Class D, which inherits class B and class C. Since Classes B and C are child classes of A and then D is the child class of B and C, Class D inherits data members of Class A from both B and C. Hence, duplication occurs, and the compiler doesn’t know what to execute and throws an error.
Output After removing line object.display():
Explanation: If we remove the line object.display() in main, the program will compile successfully, and the above output will be printed. It means two objects of class A were created, one from B and the other from C. That's why the call is ambiguous.
But this situation is avoided if the virtual base class is used.
How to Declare Virtual Base Class in C++?
Syntax
If Class A is considered as the base class and Class B and Class C are considered as the derived classes of A.
Note: The word “virtual” can be written before or after the word “public”.
Let us see an example:
Output:
In this case, we are using a virtual base class in C++, so only one copy of data from Class A was inherited to Class D; hence, the compiler will be able to print the output.
When we mention the base class as virtual, we avoid the situation of duplication and let the derived classes get only one copy of the data.
There are a few details that one needs to remember.
- Virtual base classes are always created before non-virtual base classes. This ensures all bases are created before their derived classes.
- Note that classes B and C still have calls to class A, but they are simply ignored when creating an object of class D. If we are creating an object of class B or C, then the constructor of A will be called.
- If a class inherits one or more classes with virtual parents, the most derived class is responsible for constructing the virtual base class. Here, class D is responsible for creating class A object.
A Pure Virtual Function
A pure virtual function is a function that does nothing, which means that you can declare a pure virtual function in the base class that does not have a description in the base class.
Let’s take an example of a class Animal(base class) that doesn’t implement moving, but all the animals(derived classes) must know how to move, considering that all animals can move.
Syntax for Pure Virtual Function C++
Let’s see an example using a pure virtual function:
Output:
In this example, we have created a base class Animal, which consists of a Pure Virtual Function named move(). Next, we have created two derived classes of the base class Animal, namely Lion and Wolf. Since we have declared a pure virtual function in our base class Animal, it is necessary to define the function move() in our derived classes, Lion and Wolf.
Stand out in the coding world with a C++ certification. Join our Free course and unlock the door to becoming a proficient software developer.
Conclusion
-
The article covers all the aspects of Virtual Base Class in C++ and its properties. It’ll provide a simple explanation with the help of a source code and its output. It is used in the base class to ensure the function is overridden.
-
This article covers all the aspects of Pure Virtual Function in C++ with an example of Animal Base Class.
That’s all for now, folks!
Thanks for reading.