When Do We Use an Abstract Class in Java?
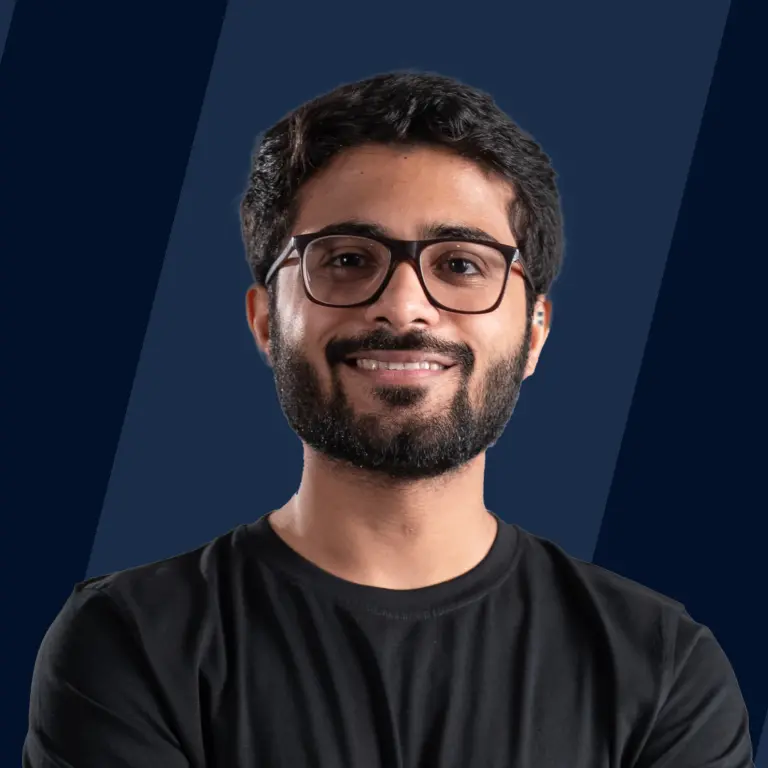
Below are the scenarios when we use the abstract class :
- If we are using inheritance and want to provide a common base class for derived classes, an abstract class can be used.
- If we want to declare non-public members, then abstract class is a better choice than interface because in an interface all methods must be public.
- If in case we need to add new methods to the class in the future, then an abstract class is a better choice than an interface because if we add new methods to an interface, then all of the classes implementing that interface will have to be changed to implement the new methods.
- If we want to declare non-static or non-final fields then the abstract class enables you to do this but in the interface, all fields are by default public, static, and final.
- We should use an abstract class if we want to make many versions of our component. Versioning our components is made simple and easy with abstract classes. All inheriting classes are automatically updated when the base class is updated. On the other side, once an interface has been developed, it cannot be modified. We must design a whole new interface whenever a new version of an interface is required.
- Use an abstract class if we want all implementations of our component to have the same implemented functionality. Interfaces include no implementation for any members, however abstract classes allow us to partially implement our class.
Note: In Java, abstraction can be achieved using abstract classes as well as interfaces.
Example:
Explanation: In this example, we have defined a Vehicle class which contains three public methods namely accelerate(), applyBrakes() and one abstract method changeGears(), also we have defined two more classes namely Car and Bike which extends the Vehicle class and we have defined the abstract method changeGears() in them.
Example of Abstraction in real-world:
Consider the case of a car, essential parts like gear, steering wheel, breaks, etc. are shown to the driver, but the driver need not know how these things are implemented internally because it is not necessary to understand the internal implementation details.
When (Not) to Use Java Abstract Classes?
- If you know that there are many unrelated child classes that require the functionality of your base class, then do not use abstract classes instead use interfaces.
- If you want to achieve complete abstraction, then do not use an abstract class instead, use an interface because an abstract class provides 0-100% abstraction, i.e., it is in our hands what percentage of abstraction we need but in the case of interfaces, 100% abstraction is there.
Example: Consider a case when we need to achieve complete abstraction, then we are using interfaces as shown in the code below.
Explanation In this example, we have defined an interface named Vehicle which contains three abstract methods, namely accelerate(), applyBrakes() and changeGears() also we have defined a class namely Car which implements the Vehicle class and we have defined all the methods in it.
Learn More
If you want to learn more about abstract classes in java then refer below : Abstarct class in java
Conclusion
- Abstraction is a way of hiding implementation details from the user and providing only essential details.
- We use abstract class when the child classes of the base class are closely related to each other.
- We don't use abstract classes when the child classes of the base class are not closely related to each other.