abs() in C++
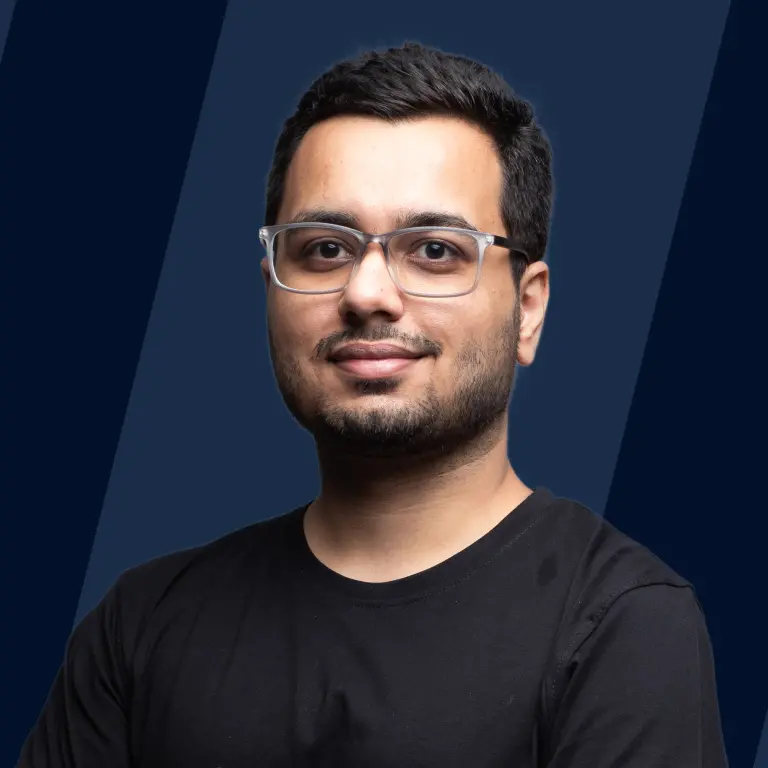
Overview
The abs() function in C++ returns the absolute value of an integer number. The absolute value of a negative number is multiplied by -1, but the absolute value of positive numbers and zero is that number itself. The absolute value is similar to modulus operator in Mathematics: abs(num) = |num|. The abs in C++ is described in <cstdlib> header file.
Syntax of abs() in C++
The syntax of abs in C++ is:
Parameters of abs() in C++
The abs in C++ take only one parameter, but it can be of three data types:
- int
- long int
- long long int
Return Values of abs() in C++
- The abs() in C++ return the absolute value of a number.
- The data type of returned value by abs in C++ will be equal to the data type of the argument passed, which means it could be int, long int, or long long int.
Example for abs() in C++
Output:
Explanation
- As we have discussed, abs() in C++, return the absolute value of the value passed. In the above code, for integer number num = -42, the absolute value of -42= |-42| = 42, so abs(-42) will return 42.
- For integer number num1 = 42, the absolute value of 42 = |42| = 42, so abs(42) will return 42.
- For integer number num2 = 0, the absolute value of 0 = |0| = 0, absolute value of 0 is 0, so abs(0) will return 0.
abs() Prototypes
There are the following three prototypes of abs in C++.
- If the num passed is of int type, then the return will be of int type.
- If the num passed is of long type, then the return will be of long type.
- If the num passed is of long long type, then the return will be of long long type.
Exceptions of abs() in C++
No-throw guarantee: this function throws no exceptions.
If the result cannot be represented by the returned type in an implementation with two's complement signed values, it causes undefined behavior.
For Example: abs is called on INT_MIN = -2147483648. The range of int in C++ is from -2147483648 to 2147483647. So if abs(-2147483648) is called, it will do (-1)*(-2147483648), which will result in it being out of range of int. Thus it will give undefined behavior.
What is cstdlib abs() in C++?
The abs() in C++ returns the absolute value of an integer number.
- If the number is negative, it will return the positive value (with the same magnitude), for example, abs(-1) = 1.
- If the number is already positive or zero, it will return the number as it is, for example, abs(1) = 1.
What is cstdlib fabs() in C++?
The fabs() in C++ returns the absolute value of a integer, float, or double-type value. In case of char type, it returns the ASCII code of that character.
- If the number is negative, it will return the positive value (with the same magnitude), for example, abs(-1) = 1.
- If the number is already positive or zero, it will return the number as it is, for example, abs(1) = 1.
Example: fabs() with char input
Code
Output
Difference Between abs() and fabs() in C++
abs() | fabs() |
---|---|
The abs() is used for integer data type. | The fabs() is used for int, float, double, and char data types. |
The abs() function is described in <cstdlib> header file. | The fabs() function is described in <cmath> header file. |
The prototype of abs() in C++ is:
The prototype of fabs() in C++ is:
abs() Overloading
The abs() in C++ can be overloaded to be used for floating, complex types .
- cmath header file is to be included for floating-point types.
- complex header file is to be included for complex numbers.
Don't miss this chance to become a certified C++ expert. Enroll in our C++ online course and gain a competitive edge in the coding landscape.
Conclusion
-
The abs in C++ returns the absolute value of an integer number. The absolute value of a negative number is that number multiplied by -1, but the absolute value of positive numbers and zero is that number itself.
-
The abs() function is used to get the absolute value of int, long, and long long data types.
-
If abs() is called for INT_MIN = -2147483648. The excepted result will be 2147483648, which is not in the range of int in C++, that is, -2147483648 to 2147483647. Thus it will give undefined behavior and throw an overflow runtime error.
-
The fabs() is used to get the absolute value of int, float, and double values.