Append to File Python
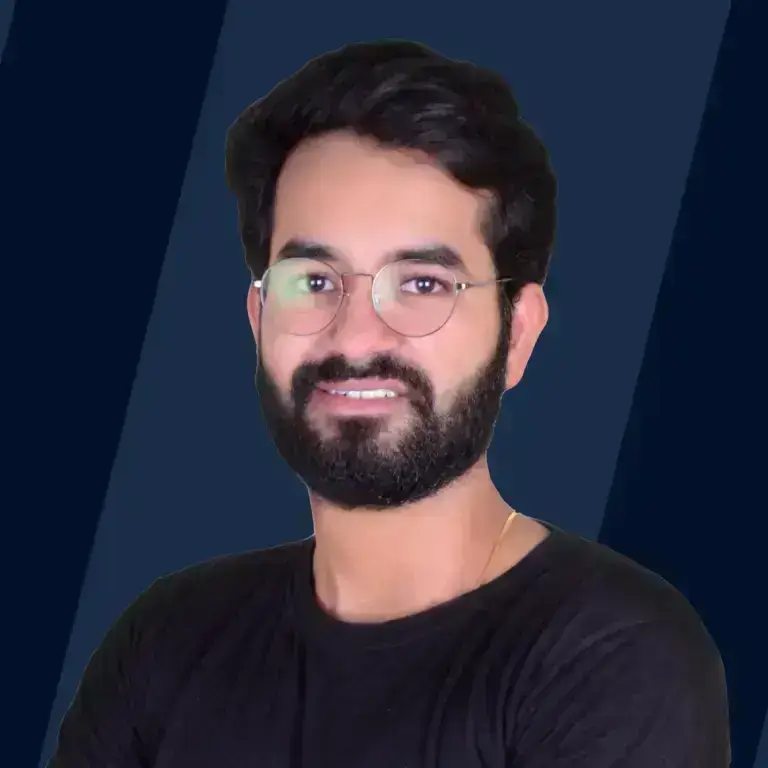
Overview
Files are used to store data permanently in non-volatile memory(like a hard disk). When we want to read or append some text to a file in Python, we first have to open it. When we are done with reading or writing the text, we must close the file so that the resources associated with it can be released. Let’s say you want to write some text to a file. First, you have to open the file in write mode by specifying "w" as the mode(the mode determines where the file is positioned and what functions are allowed), then append or write some text, and finally, close the file.
How to Append to a File in Python?
While reading or writing, we take care of access modes. Because access modes refer to how the file will be used once it’s opened, Say, we want to append to a file Python. So to do this, we have to first open the file with access mode "a", so the text will be inserted at the end of the file. Below is the command to open a file in append mode in Python. If we don't define any mode, then it will be equivalent to reading in text mode('r' or 'rt' mode).
So when the file opens with access mode ‘a’, the open() function will first check whether the file exists or not. If the file doesn’t exist, then it will create a new empty file and open it. whereas if the file exists, it will open that file in an append mode. The write file pointer will be pointing to the end of the file that is opened, and the text that we want to append to the text file will be inserted at the end of the file.
Before append:
After append:
As you can see in the above images, In the first image, we have some text, and in the second image, we append some text to the file at the end of the file.
Append Only Mode
Append-only mode is used to insert the text at the end of the file. It opens the file in append mode, and when the file is opened in append mode, the file pointer points to the end of the file. Therefore, when we pass any string in the write() function, it appends the string to the end of the file.
Let’s take an example: Suppose we have a file (the name is ) that contains some data and we want to append it to the file Python, so let’s see what would happen.
Let’s say we have some text present on our text file, the text is given below:
Scaler Topics
Scaler Academy
Scaler by InterviewBit
And, we want to append the text "Made with Love Scaler". The text will be inserted at the end of the file.
Code:
As you can see in the above code, first we open a file in an append mode, then using the write() function, we write some text to the file, and finally close the file.
Below is the output after appending the given text.
As you can see in the above output, the text is inserted at the end of the file.
Note: Sometimes only passing the text file name doesn’t work because, perhaps, the file could be in another directory. So do not make any mistakes and just pass the whole path of the text file(say ), and it will not through any error.
Append and Read Mode
mode is used to open the file for both reading and appending the text. If we want to open a file using mode and the file exists on our local system, then the file will open and the file pointer will point at the end of the file. And, if the text file is not present, then it will create a new file for reading and appending. The main difference between and mode is that in mode, when we append new text to the file, we can also read that text after appending, whereas in mode, we can only append text to the file and cannot read any text from it after appending.
Let's say we want to read something from the text file and also want to write some text (say "Here, I am again") to the text file. Now, let's see how we can do this with the help of the mode.
Code:
So, let's understand the above code line-by-line:
Line 1: Opening the file in mode(to read and write). Line 2: Write some text into the file. Line 3: Place the pointer at the start of the file so that we can read the whole text of the file. Line 4: Reading the whole text of the file. Line 5: Finally, close the file.
In Line 2, I have used one special character, "\n(newline character)". In the article below, you will see why we use this special character when we have to write text to the file.
Output of write:
[IMAGE_3 We read the text from the text file and displayed the output on the terminal.] Output of read:
Append Vs Write Mode in Python
Introduction
In general, write mode and append mode are the same, but they are different in some scenarios. Let’s discuss them one by one.
Write Mode
It opens the text file in write mode, if the file is already present. If the file is not present, then it will create a new file and open it in the write mode. In write mode, the file pointer is always pointing to the beginning of the file. So, we can say that when the file is opened in write mode, all the content that was previously present in the text file will be overwritten due to the file pointer having its position at the beginning of the file.
Append Mode
As we have already discussed above, when we want to append to file Python, we use the append mode because in the append mode, the file pointer points to the end of the file. As a result, passing any string to the write() function appends the string to the end of the file.
So, in append mode, the file pointer will be at the end of the file and any existing data won't be overwritten.
Program
Output
Explanation
As we can see in the above code that, we have an empty file and we want to write the text "Hi, Scaler by InterviewBit!" in our text file. So after writing this text, the output will look like what is given below:
Now, in our file , we have some text, and again If we want to write some other text(say "Scaler Topics"), then the text previously present in the text file will be overwritten by the text "Scaler Topics", because when we open the file in write mode, the file pointer always points to the beginning of the file.
Now, if we want to append some text to our file, remember that our text file has the text "Scaler Topics" and you want to append the text "Blogging Site". So our file will have the text "Scaler TopicsBlogging Site".
Append Data from a New Line
Introduction
In the above cases, we can see that the data is not appended from the new line. This can be done by writing the newline character(\n) to the file. So, how can we do?
We can open the file in append or write mode, so the file pointer will point to the end of the file. Then, using the write() function, append the newline character(\n) at the end of the file, which moves the file pointer to the next line so that when the new text is inserted, it will be inserted from the new line. Then, append the given text to the file, and finally, close the file.
Program
In the above code, as you can see, first we have open a file in append mode, then write some text to the file. After that, we have added a new line so that the next sentence will start from the new line. And after that, close the file.
Output:
Explanation
As you see in the above image, first we open the file in append mode, then we write some text to the file. After that, we added a new line (\n) and then again added some text. So the text that we will append after a new line character will be appended from the new line.
Append using “with the statement” in Python
Introduction
As we know, in Python, to read or write a file, we need to first open the file either in write or append mode using the open() function, and then, after performing read or write operations, we have to close the file. But what if someone forgets to close the file at the end?
The file will remain open and its objects will consume memory. Or else, the data may not be completely flushed to the file.
Now, when we append texts to the file using the "with" statement, there is no need to explicitly close the opened file, which means there is no need to use the close() function because with statement closes the file automatically. When the execution block of the "with" the statement ends, the file automatically gets closed.
Program
Output:
Explanation
We have opened the file in write mode to write some text since we are using the "with" method, so there is no need to close the file explicitly, it will automatically get closed.
Append Multiple Lines to a file in Python
Introduction
Above, we have seen that if we have to write many sentences in a text file, we have to use the write() function repeatedly. But this is not good practice. In Python, we can append multiple lines to a file in a single go. Let's see how we can do this.
We can use the writelines() method to append multiple lines to a file. We can only pass iterable objects such as a list, tuple, or set to a writelines() method.
Program
First, we open a file in append mode, then we can make a list of sentences or tuples of sentences. After that, use the writelines() method to append all the sentences in a text file.
Output:
Explanation:
As you can see in the above code, in the writelines() method, we have passed a list of arguments. And at the end of every sentence, there is a newline character that will move every other sentence to the new line, as you can see in the above output. In the above code, first we passed a list as an input to the writelines() function, and then a tuple as an input. So, we can only pass iterable objects, i.e., either a list, tuple, or a set.
Conclusion
Let's conclude our topic append to file Python by mentioning some of the important points.
- Files are used to store data permanently in non-volatile memory(like a hard disk).
- While reading or writing, we take care of access modes. Because access modes refer to how the file will be used once it’s opened.
- Append-only mode is used for writing the text in a file. It opens the file in append mode, and when the file is opened in append mode, the file pointer points to the end of the file.
- mode is used to open the file for both reading and appending the text.
- When the file is opened in write mode, all the content that was previously present in the text file will be overwritten due to the file pointer having its position at the beginning of the file.
- In append mode, the file pointer will be at the end of the file and any existing data won’t be overwritten.