with Statement in Python
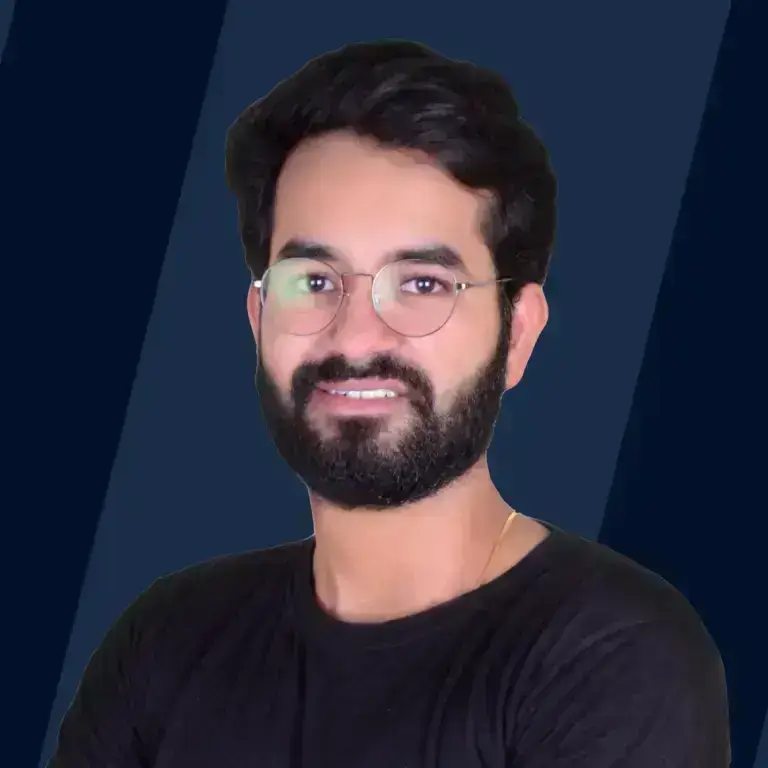
Python's with statement is used for resource management and exception handling. It simplifies the management of shared resources like file streams, ensuring that resources are properly cleaned up after use, regardless of whether an exception is raised. This statement makes the code cleaner and much more readable.
Here's a concise example using the with statement for file handling:
In this example, the with statement opens a file named 'example.txt' in read mode. The file is bound to the variable file. After the indented block is executed, where the file's contents are read, the with statement ensures the file is automatically closed, even if an error occurs within the block.
Using “with” Statement in User-defined Objects
“with” supports a lot of built-in methods like open()(used for opening files), but we can also use it in our custom methods. We must follow some conditions to use it in our custom methods. Let’s see how it can be done.
The “With” statement can help in many tasks that must be performed at the start or end of a procedure.
E.g. In the case of file handling, you can define methods that can open and close the file for you. Or you are creating an app where you need to contact a database; you need to start a connection when the data transfer takes place and end the connection when the transfer is done.
“with” has a condition that it works with objects that support context management protocol, or it works with objects that have an __enter()__ and __exit()__ method.
We can make our class with these methods, and that will enable us to use “with”.
Here is an example of a simple class.
Output:
In the above code, we created a class MyContextManager and created two methods named __enter__() and __exit__(). When we call this class using “with” we can see that the __enter__() runs first and then __exit__() runs.
In the code *args and **kwargs are used because __exit__() takes three arguments
- execution_type
- execution_value
- traceback
These arguments should occur in this order.
- execution_type is the exception’s class.
- execution_value is an exception instance that indicates the type of exception like Zero Division Error, Floating Point Error
- traceback is traceback object
When there are no exceptions raised, then all these args will return None, None, None.
Using “with” Statement to Close a File
Using Python's with statement to close a file is an efficient and error-proof method. It eliminates the need to explicitly call the close() method on a file object. This is particularly useful for ensuring that files are closed properly, even if an error occurs while processing the file.
When you open a file using the with statement, Python automatically closes it when the code block under the with statement is exited, whether it usually exits or due to an exception.
Here's an example demonstrating its usage:
In this example, example.txt is opened in read mode. The file is accessible within the block of the with statement. After the block is executed, the file is automatically closed by Python, regardless of whether the operations were successful or an exception occurred. This automatic management of resource cleanup makes the with statement a preferred choice for file handling in Python.
Replacing try-catch Blocks Using “with” statement
Replacing traditional try-catch blocks with the with statement in Python can streamline error handling and resource management in certain scenarios, particularly when dealing with resource-intensive operations like file handling, network connections, or database access.
The with statement simplifies code by abstracting the complexity of try-catch blocks, especially for ensuring the proper acquisition and release of resources. It's commonly used when resources must be properly closed or released, regardless of whether an exception occurs.
Here's an example of replacing a try-catch block with a with statement for file handling:
Traditional try-catch Block
Using with Statement
In the traditional try-catch block, you have to manually open and close the file, handling any exceptions that might occur. The finally block ensures the file is closed, whether an exception is raised or not.
In contrast, the with statement automatically handles the opening and closing of the file. It ensures that the file is closed when the block is exited, even if an error occurs. This makes the code cleaner, more readable, and less prone to errors like forgetting to close the file.
The Contextlib Library
contextlib library has a “contextmanager” decorator, providing an alternative and simple way to implement context management protocol. This reduces a lot of boilerplate code as it automatically provides __enter()__ and __exit()__ methods.
Let’s look at an example.
Output:
First, you will notice that no “class” is used here. The decorator
“@contextmanager” allows us to create a function-based context manager.
There are two parts to the function. The code before yield behaves like __enter()__ and the code after yield behaves like __exit()__.
Yield is a keyword used like return, except the function where it is used will return a generator.
Conclusion
with is a valuable keyword but should be used carefully.
Some advantages of with keyword are:
- It makes resource management safer
- Cleans up the code compared to try…finally
- Helps in avoiding resource leaks
The only thing to remember is that with only works with the objects that follow context management protocol.