What are the Applications of Linked List?
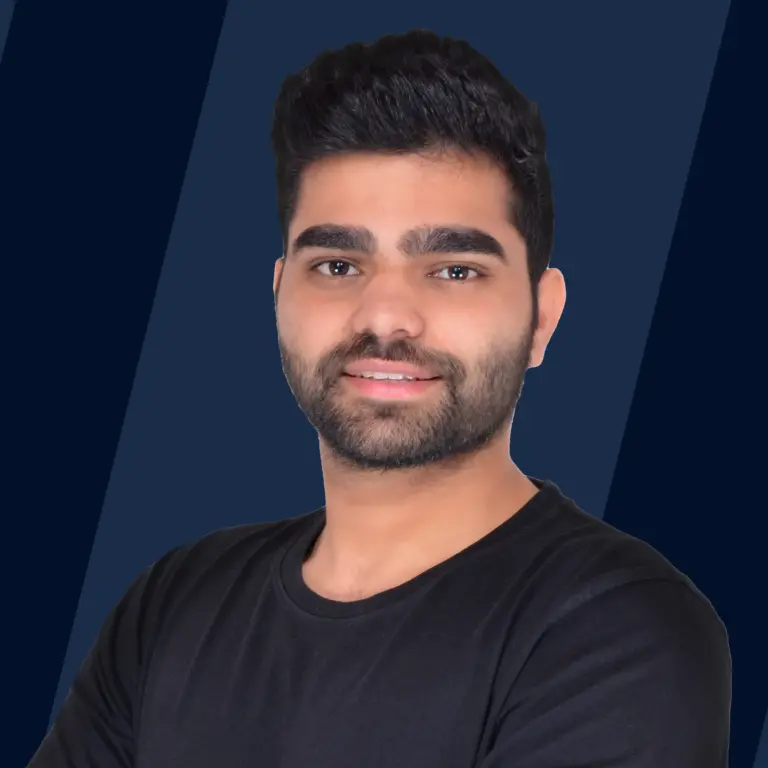
What is a Linked List ?
A linked list is a linear data structure. It is a collection of nodes, and a node contains data and addresses the next node. Linked lists do not use contiguous memory allocation for storage, unlike arrays.
What are the Applications of Linked List ?
There are many applications of linked lists, be it in computer science or the real world.
Some of these Applications are :
Applications of Linked List in Computer Science :
- Linked lists can be used to represent polynomials.
- Using a linked list, we can perform the polynomial manipulation.
- Arithmetic operations like addition or subtraction of long integers can also be performed using a linked list.
- The linked list can be used to implement stacks and queues.
- The linked list is also used in implementing graphs in which the adjacent vertices are stored in the nodes of the linked list popularly known as Adjacency list representation.
Applications of Linked Lists in the Real World :
- In music players, we can create our song playlist and can play a song either from starting or ending of the list. And these music players are implemented using a linked list.
- We watch the photos on our laptops or PCs, and we can simply see the next or previous images easily. This feature is implemented using a linked list.
- You must be reading this article on your web browser, and in web browsers, we open multiple URLs, and we can easily switch between those URLs using the previous and next buttons because they are connected using a linked list.
Applications of Circular Linked Lists :
- The circular linked list can be used to implement queues.
- In web browsers, the back button is implemented using a circular linked list.
- In an operating system, a circular linked list can be used in scheduling algorithms like the Round Robin algorithm.
- The undo functionality that is present in applications like photo editors etc., is implemented using circular linked lists.
- Circular linked lists can also be used to implement advanced data structures like MRU (Most Recently Used) lists and Fibonacci heap.
Applications of Singly Linked List :
- The singly linked list is used to implement stack and queue.
- The undo or redo options, the back buttons, etc., that we discussed above are implemented using a singly linked list.
- During the implementation of a hash function, there arises a problem of collision, to deal with this problem, a singly linked list is used.
Application of Doubly Linked Lists :
- The doubly linked list is used to implement data structures like a stack, queue, binary tree, and hash table.
- It is also used in algorithms of LRU (Least Recently used) and MRU(Most Recently Used) cache.
- The undo and redo buttons can be implemented using a doubly-linked list.
- The doubly linked list can also be used in the allocation and deallocation of memory.
Polynomial Manipulation
Polynomials are algebraic expressions that contain coefficients and variables. Polynomial manipulation is doing mathematical operations, like addition, subtraction, etc., on polynomials.
Polynomials are a very important part of mathematics, and there aren't any direct data structures present that can be used to store polynomials in memory. Thus, we take the help of a linked list to represent a polynomial.
To represent the polynomials using a linked list, we assume that each node of the linked list corresponds to each term of the polynomials.
Let us see how a polynomial is represented in a linked list.
The node of the linked list contains three parts :
- the coefficient value,
- the exponent value, and
- the link to the next term.
For example the polynomial can be represented as
C++ Program for Addition of Two Polynomials
To add two polynomials, we first represent both of them in the form of a linked list. And then, we add the coefficients having the same exponent.
For example, suppose we want to add two polynomials that are : and .
C++ Program :
Output :
Time Complexity :
The time complexity of the program would be , where a will be the Number of nodes in the first linked list and b will be the Number of nodes in the second linked list since we are traversing both lists at once.
Addition of Long Positive Integer Using Linked List
In most programming languages, there is always a limit on the maximum value of an integer that it can store. But sometimes, there might be cases where we need to add two numbers, and the resultant number exceeds the limit of the integer.
We can do this by representing the numbers in the form of a linked list and then performing the addition on the linked list and storing the result into the resultant linked list.
To perform addition, we traverse both the linked lists parallelly, and then we add the corresponding digits and carry obtained from the previous edition, and we store that value in the resultant linked list.
For example, suppose we want to add two numbers : 543467 and 48315.
C++ program for the addition of two polynomials using Linked Lists :
Output :
Time Complexity :
The time complexity of the program would be , where a will be the Number of nodes in the first linked list and b will be the Number of nodes in the second linked list since we are traversing both lists at once.
Polynomial of Multiple Variables
In polynomials, we can have more than one variable. Such polynomials can also be represented using a linked list in the same manner. In the case of multiple variables, each node of the linked list contains a separate part for each exponent. That is, if there are three variables, then there will be three parts for exponents.
Suppose we have polynomial .
It can be represented as a linked list :
Simple C++ program to multiply two polynomials :
To multiply two polynomials that are given in the form of an array, we simply traverse the first polynomial and multiply its every term with each term of the second polynomial.
Output :
Time Complexity :
The time complexity of the given program will be , where a is the size of the first array and b is the size of the second array.
Some Other Applications of Linked List
Some important applications of a linked list include :
- Allocation of Memory
- Email applications
- Reducing file sizes on disk
- Implementation of advanced data structures
Advantages of Linked List Over Arrays
A few advantages of linked lists over arrays are :
- Dynamic size
- Efficient implementation of data structures
- No memory wastage
- Efficient insertion and deletion operation
Learn More
After reading this article, you might be getting curious to learn more about linked lists, or there might be some topics that you did not understand. To learn more about the linked list, you can refer to other articles present on Scaler topics on Linked list.
Conclusion
So far, we have discussed a lot about linked lists and their applications.
A few important points that we covered are :
- Linked lists have many applications both in computer science and in the real world.
- Some computer science applications include polynomial manipulations, implementation of advanced data structures, etc.
- Few real-world applications include web browsers, back buttons, music players, image viewers, etc.
- We can perform operations like addition, multiplication, etc. on polynomials with the help of linked lists.