Scope, Visibility and Lifetime of a Variable in C
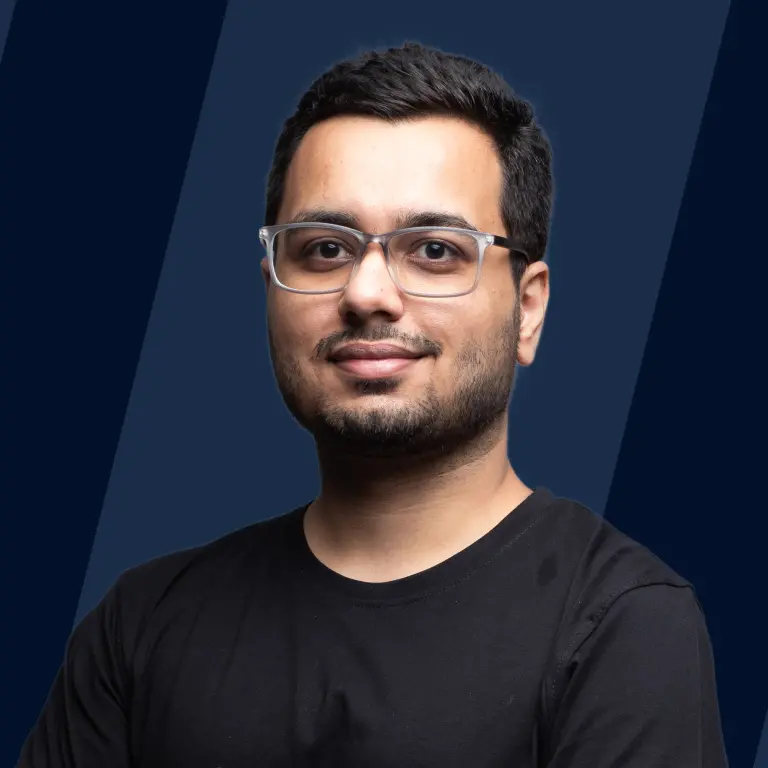
Overview
Scope, Visibility and Lifetime of variables in C Language are very much related to each other, but still, there are some different properties associated with them that make them distinct. Scope determines the region in a C program where a variable is available to use, Visibility of a variable is related to the accessibility of a variable in a particular scope of the program and Lifetime of a variable is for how much time a variable remains in the system's memory.
Introduction
Scope, Visibility and Lifetime can be understood with a simple real-life example of Netflix, Hotstar or Amazon Prime. There are Movies / TV Series present on these platforms that have local/global scope, visibility and a lifetime. Movies based on local languages like Hindi, Japanese, and Korean have a limited scope. They can be watched (accessible) in a limited area, while movies with global languages like English have a global scope and are available throughout the world to watch. These movies also have a lifetime, and once that lifetime is over these movies are removed from the streaming platforms.
Coming to the appropriate definitions for Scope, Visibility and Lifetime of a variable :
- Scope is defined as the availability of a variable inside a program, scope is basically the region of code in which a variable is available to use.
There are four types of scope:- file scope,
- block scope,
- function scope and
- prototype scope.
- Visibility of a variable is defined as if a variable is accessible or not inside a particular region of code or the whole program.
- Lifetime of a variable is the time for which the variable is taking up a valid space in the system's memory, it is of three types:
- static lifetime,
- automatic lifetime and
- dynamic lifetime.
Now, let's see what is the scope of a variable in C.
What is the Scope of Variables in C?
Let's say you reside in an apartment complex, and you only have one key to use to get access to your room. The apartment's owner/manager may also have a master key that grants access to all the rooms.
The scope of variables in C have a similar idea. The availability of a variable in a program or function is referred to as the scope of variable in C language.
A variable, for example, may be accessed only within a single function/block of code (your apartment key) or throughout the whole C program (the shared access key). We can associate the room keys with the local variables in C language because they only work in that single room. The term global variables refers to variables (keys) that are accessible to the whole program (apartment complex).
Now, let's see the four types of scope of a variable in C:
1. File Scope
File scope of variables in C is defined as having the availability of the variable throughout the file/program. It means that the variable has a global scope, and it is available all around for every function and every block in the program.
Example :
C Program :
You can run and check your code here. (IDE by InterviewBit)
Output :
Explanation : A global variable x is declared having file scope. main() function and func() function are able to access the variable x because it has file scope. In the code, first we have increased the value of x by 10 (x = 20 now) and then by 30 (x = 50 now), and we can see from the output that x preserves its value 20 after the changes made in the function func() because it has file scope.
2. Block Scope
Block scope of variables in C is defined as when the variable has a limited scope, and the memory occupied by the variable will be deleted once the execution of the block ends. The variable is not accessible or available outside the block. A block of code can be defined in curly braces {code_block}.
Example :
C Program :
You can run and check your code here. (IDE by InterviewBit)
Output :
Explanation : In the above program, the sum variable has block scope, we can't access the sum variable outside this block where sum is declared. If we uncomment the printf("Sum of a and b: %d", sum); statement then the compiler will throw an error of undeclared sum variable in the current scope.
3. Function Scope
Function scope of variables in C begins with the left curly brace { and ends with a closing right curly brace }. A variable declared inside a function has a function scope. It has been allocated memory when the function is called, and once the function returns something, the function execution ends and with it, the variable goes out of scope, i.e. it gets deleted from the memory.
Example :
C Program :
You can run and check your code here. (IDE by InterviewBit)
Error :
Explanation : We can see in the output that a compilation error is thrown to us because we are using an age variable outside the function from where it is declared. Compiler has thrown an error because age variable is not available outside the function as age variable only has function scope.
4. Function Prototype Scope
Function prototype scope of variables in C are declared in some function as its parameters. These variables are similar to the function scope variables where a variable's memory gets deleted once the function execution terminates.
Example :
C Program :
You can run and check your code here. (IDE by InterviewBit)
Output :
Explanation : When the findSum() function is invoked, space for variables a and b is allocated in the system's memory. Variables a and b are findSum() function parameters and these variables have function prototype scope, we can access these variables only in the function definition and not outside of it.
Example Showing Various Scope of Variables in a C Program
Let's see the various scope of variables in C program that we have seen above to get more clarity on the topic.
C Program :
You can run and check your code here. (IDE by InterviewBit)
Output :
Explanation :
- First, we have declared two variables int A, double B, outside of all the functions, i.e. as global variables, so these variables have file scope.
- Now, inside the main() function, double PI and int radius variables are declared, these variables have function scope and are not available outside the main() function.
- Now in the inner block of code, we have declared double Area, int A, double B, int C and int sum variables and these variables have block scope, these variables are not available outside this code block.
- In the block of code we have also called a function sumOfTwoNumbers() and we have defined the function as int sumOfTwoNumbers(int a, int b), here function parameters are a and b and these variables have function prototype scope, while the sum variable inside the function has function scope.
Scope Rules
Scope of variables in C also have some rules demonstrated below. Variables can be declared on three places in a C Program :
- Variables that are declared inside the function or a block of code are known as local variables.
- Variables that are declared outside of any function are known as global variables (usually at the start of a program).
- Variables that are declared in the definition of a function parameters as its formal parameters.
1. Local Variables
When we declare variables inside a function or an inner block of code, then these variables are known as local variables. They can only be used inside the function scope or the block scope.
Example :
You can run and check your code here. (IDE by InterviewBit)
Output :
Explanation :
We can see in the output that a compilation error is showing to us because we are using a and b variables outside the block where they are declared. It has thrown an error because a and b are not available outside the above block of code.
2. Global Variables
When we declare variables outside of all the functions then these variables are known as global variables. These variables always have file scope and can be accessed anywhere in the program, they also remain in the memory until the execution of our program finishes.
Example :
You can run and check your code here. (IDE by InterviewBit)
Output :
Explanation : As we have declared a side variable outside of the main() function, it has file scope or it is known as a global variable. We are using the side variable to calculate the area of the square in the main() function.
3. Formal Parameters
When we define a function and declare some variables in the function parameters, then these parameters are known as formal parameters/variables. They have a function prototype scope.
Example:
You can run and check your code here. (IDE by InterviewBit)
Output :
Explanation : We have defined a function square() to calculate the square of a number. The int n variable in the square() function parameters is a formal parameter having function prototype scope.
Rules of use
- Global variables have a file scope, i.e. they are available for the whole program file.
- The scope of a local variable in C starts at the declaration point and concludes at the conclusion of the block or a function/method where it is defined.
- The scope of formal parameters is known as function prototype scope and it is the same as its function scope, we can access the variables only in the function definition and not outside it.
- Although the scope of a static local variable is confined to its function, its lifetime extends to the end of program execution.
What is Visibility of a variable in C?
Visibility of a variable is defined by how a variable is accessible inside a program. A variable is visible within its scope and hidden outside the scope. A variable's visibility controls how much of the rest of the program may access it. You can limit the visibility of a variable to a specific section of a function, a single function, a single source file, or any place in the C program.
The visibility and Scope of a variable are very similar to each other but every available variable (in the scope) is not necessarily accessible (visible) in a C program. Let's see this with an example, where we declare two variables having the same name in two different scopes, the variable declared in a bigger scope is available in the block having smaller scope, but it is not accessible because the smaller scope variable has more priority in accessing the variable declared in the respective inner block of code.
C Program :
You can run and check your code here. (IDE by InterviewBit)
Output :
Explanation :
- We have declared an integer variable scope in the main() function and a same named variable scope having float data type in an inner block of code.
- int scope variable have function scope, while float scope variable have block scope.
- Even if the outer int scope variable is available inside the inner block of code, it is not accessible (visible) because a same name variable float scope is declared having block scope and a higher priority of accessibility in the respective block of code than the int scope variable.
- Assigning a value to the inner scope variable in the inner block of code doesn't affect the outer scope variable.
What is the Lifetime of a variable in C?
Lifetime of a variable is defined as for how much time period a variable occupies a valid space in the system's memory or lifetime is the period between when memory is allocated to hold the variable and when it is freed. Once the variable is out of scope its lifetime ends. Lifetime is also known as the range/extent of a variable.
A variable in the C programming language can have a
- static,
- automatic, or
- dynamic lifetime.
a. Static Lifetime
Objects/Variables having static lifetime will remain in the memory until the execution of the program finishes. These types of variables can be declared using the static keyword, global variables also have a static lifetime: they survive as long as the program runs.
Example :
The count variable will stay in the memory until the execution of the program finishes.
b. Automatic Lifetime
Objects/Variables declared inside a block have automatic lifetime. Local variables (those defined within a function) have an automatic lifetime by default: they arise when the function is invoked and are deleted (together with their values) once the function execution finishes.
Example :
auto_lifetime_var will be deleted from the memory once the execution comes out of the block.
c. Dynamic Lifetime
Objects/Variables which are made during the run-time of a C program using the Dynamic Memory Allocation concept using the malloc(), calloc() functions in C or new operator in C++ are stored in the memory until they are explicitly removed from the memory using the free() function in C or delete operator in C++ and these variables are said to have a dynamic lifetime. These variables are stored in the heap section of our system's memory, which is also known as the dynamic memory.
Example :
Memory block (variable) pointed by ptr will remain in the memory until it is explicitly freed/removed from the memory or the program execution ends.
Example Program with Functions and Parameters
C Program :
You can run and check your code here. (IDE by InterviewBit)
Output :
Explanation :
- Variables num2 and j as well as function parameters a and b are local to the function func1() and have function scope and prototype scope respectively.
- Variables declared in function func2() and main() function are not visible in func1().
- The variables num1 and num2 are global variables having file scope.
- num1 is visible in func1() but num2 is hidden/invisible by the local declaration of num2 i.e. int num2.
What is the Difference Between Scope and Lifetime of a Variable in C?
Scope Of A Variable in C | Lifetime Of A Variable in C |
---|---|
Scope of a variable determines the area or a region of code where a variable is available to use. | Lifetime of a variable is defined by the time for which a variable occupies some valid space in the system's memory. |
Scope determines the life of a variable. | Life of a variable depends on the scope. |
Scope of a static variable in a function or a block is limited to that function. | A static variable stays in the memory and retains its value until the program execution ends irrespective of its scope. |
Scope is of four types: file, block, function and prototype scope. | Lifetime is of three types: static, auto and dynamic lifetime. |
Conclusion
- The Scope, Visibility and Lifetime of variables are important concepts in C Language that determine how the data (variables/objects) and the functions interact with each other.
- Scope of a variable determines the region where a variable is available.
- Visibility of a variable controls how much of the rest of the program may access it.
- Lifetime of a variable in C is the time for which a variable stays in the memory.