Calendar Module in Python
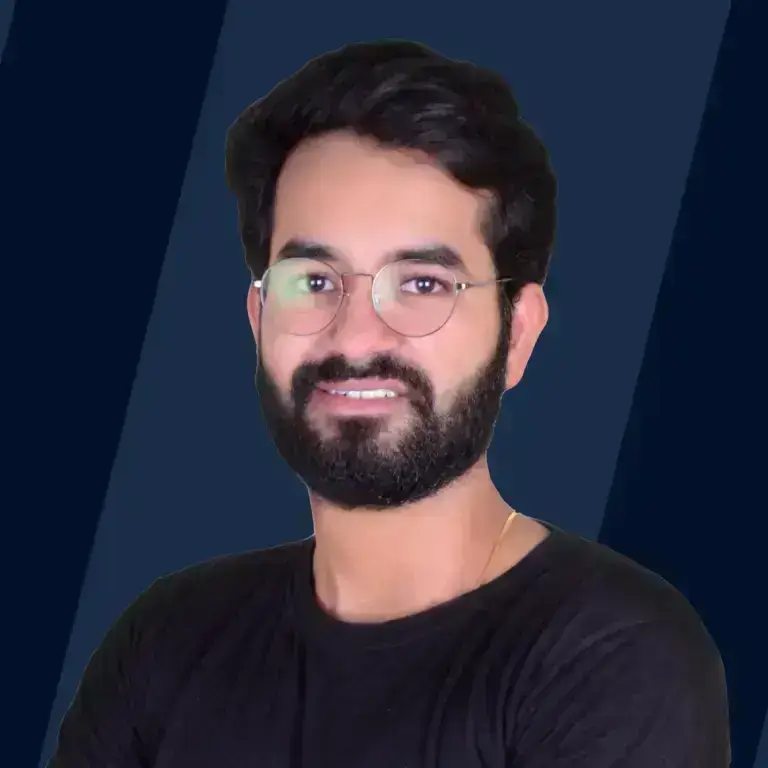
Overview
The calendar module helps in working with calendars i.e. dates, months and years in Python. This module also enables the user to format (beautify) the calendar as per their requirements and output it in the form of text or in HTML.
Introduction to Calendar Module in Python
If you want to set up a personal planner, or maybe practice your coding problem of the day, your friendly garden snake language python has you covered. How so?
Python has a built-in module called the Calendar module which allows you to perform date, month, and calendar-related operations while even letting you manipulate your code for some specific day or month of the year.
The Calendar module in python uses the idealized calendar which is the current Gregorian calendar. It is extended in both directions (past and future) indefinitely. These calendars have the first day of the week as Monday and the last day of the week as Sunday.
For example, look at the image below, where we have the month of August 2019, starting from Monday and ending on Sunday.
Now in this calendar module in python, you have 2 classes - the TextCalendar class and the HTMLCalendar class which you can use to edit the calendar according to your requirements. We will get into these details later, let's first look at how to display the calendar of a given year and month.
First, we must import the Calendar module, declare a particular year and month and then print the calendar using the "month" function in the calendar module. The month function takes the year and month as input and outputs a well-formatted month, i.e. with proper spacing between the columns as you can see in the example below.
Output:
As you can see, in the output we have a beautifully formatted month that starts on Monday. You can change the year and month to test it out yourself.
Now if you wanted to print the calendar of an entire year, you could make use of the "calendar" function inside the calendar module in python. It will take the year as input.
Test it on your machine to view the entire calendar for the year 2022.
The Calendar Class
Let's now take a look at the calendar class of the calendar module in python. This class creates a Calendar object which provides us with multiple methods that can be used to prepare the calendar data for formatting purposes. The formatting is not done by this class itself, we have various subclasses for that like, the HTMLCalendar, the TextCalendar class, and the simple calendar class.
Using the calendar class, we can perform calculations for various tasks that are based on months, dates and years. Here are the methods of the Calendar class:
Function | Description |
---|---|
iterweekdays() | For all the week day numbers that would be used for one week, one iterator is returned |
itermonthdates() | An iterator for all the months from 1 - 12 in the year is returned |
itermonthdays() | This returns an iterator of the month and year specified |
itermonthdays2() | This function is similar to the previous one however it returns days in the form of tuples that consist of the day of the month and week day number |
itermonthdays3() | This function is similar to itermonthdates() and it returns an iterator for the month of the year, but like itermonthdays2() it returns days in the form of tuples consisting year, month and day of the month numbers |
monthdatescalendar() | Function returns a list of the weeks of a particular month, with each week being a list of datetime.time objects, which are 7 in number (7 days in a week). |
monthdays2calendar() | This function is same as monthdatescalendar() however, here weeks are tuples of day and week numbers |
monthdayscalendar() | A list of weeks in the particular month of that year is returned. Here, the weeks are lists of the 7 day numbers |
yeardatescalendar() | A list of month rows is returned which is essentially data for a particular year, ready to be formatted |
yeardays2calendar() | This function is similar to the yearsdatescalendar() function however, the weeks are formatted in the form of tuples of day and weekday numbers |
yeardayscalendar() | Similar to the yeardatescalendar() as well, this function has the entries of the week as lists of day numbers. Any day numbers that are outside this month have the value 0 |
To better understand all these functions, it is recommended that you try them on your own and see their outputs.
Take a look at the implementation of the monthdatescalendar() function:
Output:
As you can see in the output above, we have a list of lists. Each list contains 7 datetime.date objects for every day in the week of 3,2022 i.e. March 2022. The outer list denotes the month and every list inside denotes the week.
TextCalendar class
Another class present in the calendar module is the calendar. TextCalendar class which has functions that help in the generation of plain text calendars. We did a similar thing without using the textcalendar class in the beginning when we printed the month of march of year 2022, however, with this class you will be able to edit the calendar as well and use it as per your requirement.
The following are the functions available in the calendar.TextCalendar class:
Function | Description |
---|---|
formatmonth() | This method is used to get the calendar object of a month in the form of a mult iline string. Same as calendar.month, however you can edit the number of lines per week and, first week day and the width of date columns |
prmonth() | This is a helper function used to print the calendar that is returned by formatmonth() |
formatyear() | Similar to the formatmonth() method, this allows us to get the calendar of the entire year, with m columns (you are required to specify m in the input parameters) |
pryear() | This method is also a helper that aids printing the object returned by the formatyear() method |
Take a look at an example implementation of the formatmonth() and prmonth() methods:
Output:
You can see that the output of the month method directly prints the month calendar. You can also make changes to the values of w and l to see the spacing in the calendar change.
HTMLCalendar Class
The calendar module has another class, called the HTMLCalendar class which generates the code for calendars in HTML.
It has the following methods:
Method | Description |
---|---|
formatmonth() | This method returns the calendar of a particular month in the form of an HTML table |
formatyear() | This method returns the calendar of a particular year in the form of an HTML table |
formatyearpage() | Using this method we can get the complete year's calendar as a complete HTML page |
Let's look at an example of the formatmonth() method:
Output:
March 2022 | ||||||
---|---|---|---|---|---|---|
Mon | Tue | Wed | Thu | Fri | Sat | Sun |
1 | 2 | 3 | 4 | 5 | 6 | |
7 | 8 | 9 | 10 | 11 | 12 | 13 |
14 | 15 | 16 | 17 | 18 | 19 | 20 |
21 | 22 | 23 | 24 | 25 | 26 | 27 |
28 | 29 | 30 | 31 |
Simple Text Calendars
Now that we have discussed the classes provided by the calendar module, let's look at some of the helpful methods in the simple calendar module which are separate from the above two classes.
Method | Description |
---|---|
setfirstweekday() | This method is used to set the first day of the week. The days of the week are provided in the function as MONDAY, TUESDAY ... SUNDAY for convenience, however, you can also make use of numbers 0 - 6 where 0 is Monday and 6 is Sunday |
firstweekday() | With the use of this method we can get the current weekday that is set as the first day of the week |
isleap() | As the name of the method suggests, it tells us whether a year is leap or not. It returns true if the year is a leap year and false, if not |
leapdays() | This method returns the number of leap years present in a specified range of years given as input. |
weekday() | This method returns the day of the week on a particular date. For example, 15 may 2016 as input to this method will return 6 = sunday |
weekheader() | We can use this method to get a header that contains the weekday names in abbreviated format |
monthrange() | This method returns the weekday of the first day of the month and the number of days in that month as a tuple for any specified year and month given as input |
monthcalendar() | Returns a matrix that represents the calendar of a month where every row is representative of the week, and the days outside the specified month are represented as zeroes |
prmonth() | This method is used to print the calendar of a month with formatting as per user. The user can format the width between two columns and the number of blank lines between rows |
month() | Returns the calendar of a month in the format of a multiline string |
prcal() | Used to print the calendar of the complete year with options for formatting the output |
calendar() | This method is as we discussed in the beginning of the article, used to print the 3 - column calendar of a year |
Let's test out the isleap() method:
Output:
Try out the methods of all these classes on your own to better understand the calendar module in python. You now know all there is to the calendar module.
Conclusion
- The calendar module helps in working with calendars in Python. This module also enables the user to format the calendar as per their requirements and output it in the form of text or in HTML.
- It has the following classes - calendar, TextCalendar, and the HTMLCalendar class.
- The HTMLCalendar class is used to generate calendars in HTML
- The TextCalendar class is used to create plain text calendars
Click Here, To know more about format() in python.