JavaScript Function call() Method
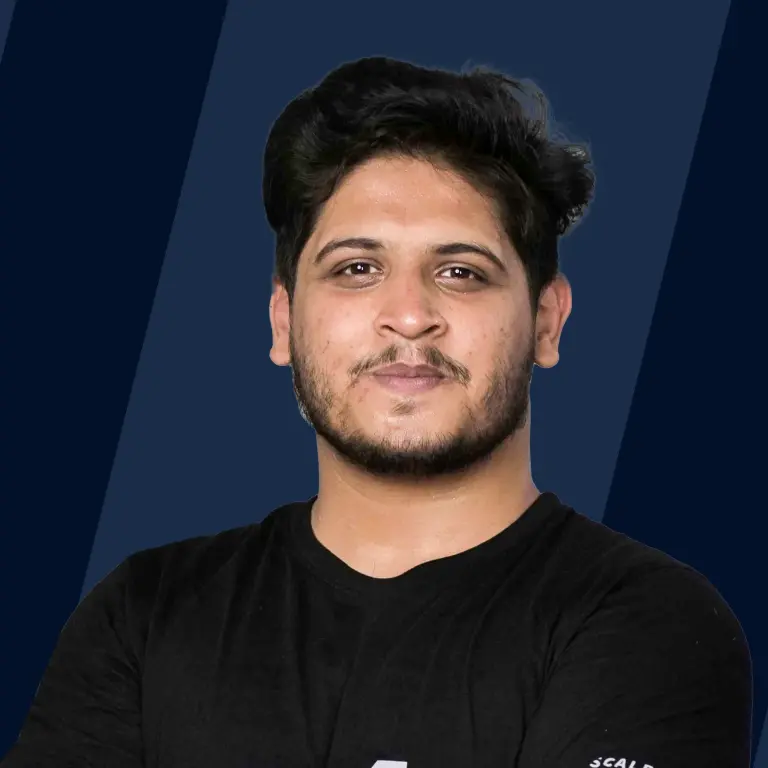
This article call() in JavaScript explores how the call() method facilitates the invocation of functions from one object to another object. With Function.prototype.call(), you can effortlessly control how a function behaves and what it can access. It's like giving a function a new set of instructions, allowing it to tap into different data and settings. Whether you want to borrow methods from one object to use in another or create flexible functions that adapt to different situations, Function.prototype.call() is your secret weapon.
Syntax
The call method invokes a function with a specified context (thisArg) and arguments. Its syntax is as follows:
The call method immediately invokes myFunction with arguments and thisArg as the context. thisArg is optional and determines the execution context. If omitted, this defaults to a global object (non-strict mode) or undefined (strict mode). Additional arguments can be passed. The return value of call is the same as the invoked function.
Parameters
The following are the parameters of call() in javascript:
- thisArg (optional): the this value (context) when invoking the function.
- ...argN (optional): function arguments as they are present in the calling function.
The call method takes a thisArg (context) and a list of arguments, similar to the calling function. The thisArg can be any context or object, including the global context, and is optional. The arguments should be passed unchanged, unlike apply where a specific argument list is used. The function parameters are also optional.
Return Value
Return Type: same as the return type of the calling function
The return value of the call method is the return value of the calling function with the passed thisArg value and the arguments.
Description
The Function call is a standard JavaScript mechanism for writing methods for various objects. The method is called with the owner object as a parameter. this corresponds to the "owner" of the function or object to which it belongs. In JavaScript, all functions are defined as object methods. If a function isn't classified as a method of a JavaScript object, it becomes a method on the global object.
Exceptions of call() Function in Javascript
- In some cases, thisArg may not be the actual value seen by the procedure.
- In non-strict mode, null and undefined are replaced with the global object, and primitives are converted to objects if the method is a function.
- The syntax of call() differs from apply() in that it takes individual arguments, while apply() takes an array of parameters.
- Passing an object as the this value is not required, but it's done here for demonstration.
Example of Call Method Invocation
Here is a short example to understand the fundamental working of the call method
In the above example, we can define a function greet that logs the greeting, but the greeting comes from the this, which is the context that will be supplied, and the name is a parameter that is passed as an argument. The obj declared above has a property called sal. So when we invoke greet by passing obj’s context compiler will refer sal from the obj context, and the output will be "hello Michael".
More Examples
Example 1: Constructor Chaining Using the Call Method
Call in JavaScript can be used to chain constructors for objects. Defining the logic once in a function that can be repeated again.
Constructor chaining using the call method allows for reusing logic by defining it in a function that can be repeated. In the given example, the Person constructor is defined, and the Student and Teacher functions utilize the Person logic by calling it with the call method. This initializes properties and sets the specific object's additional properties.
Example 2: Using Call to Invoke Anonymous Functions
You can use the call method on anonymous functions to immediately invoke them.
Here we create an anonymous function that prints the values along with their index. We use call() to invoke on each of the objects in the array.
Example 3: Using call() to Invoke a Function and Specifying the Context for 'this'
We can specify the this context on the call method by passing a specific object as the thisArg.
This is the previous example where we pass obj as the thisArg, thus binding the this to obj.
Example 4: Using call() to Invoke a Function without Specifying the First Argument
The call method can be invoked without providing the first argument, in which case the call method takes the global context (window object in the browser and global in NodeJS).
Here, on invoking send.call, the msg from the global context is used as the this gets bound to the global object when not specifying the thisArg.
Browser Compatibility
Google Chrome >= 1.0,
Firefox >= 1.0,
Microsoft Edge >= 12.0,
Internet Explorer >= 5.5,
Opera >= 4.0,
and Safari >= 1.0.
Conclusion
- The Function call in JavaScript is a standard mechanism for writing methods for various objects.
- The syntax to invoke the call method is to pass in the context and the arguments list. The call method takes a thisArg (the context) and the list of arguments as they are in the calling function.
- The return value of the call method is the return value of the calling function with the passed thisArg value and the arguments.
- Although the syntax is nearly identical to that of apply(), the main distinction is that call() takes an argument list, whereas apply() takes a single array of parameters.