Callable Interface in Java
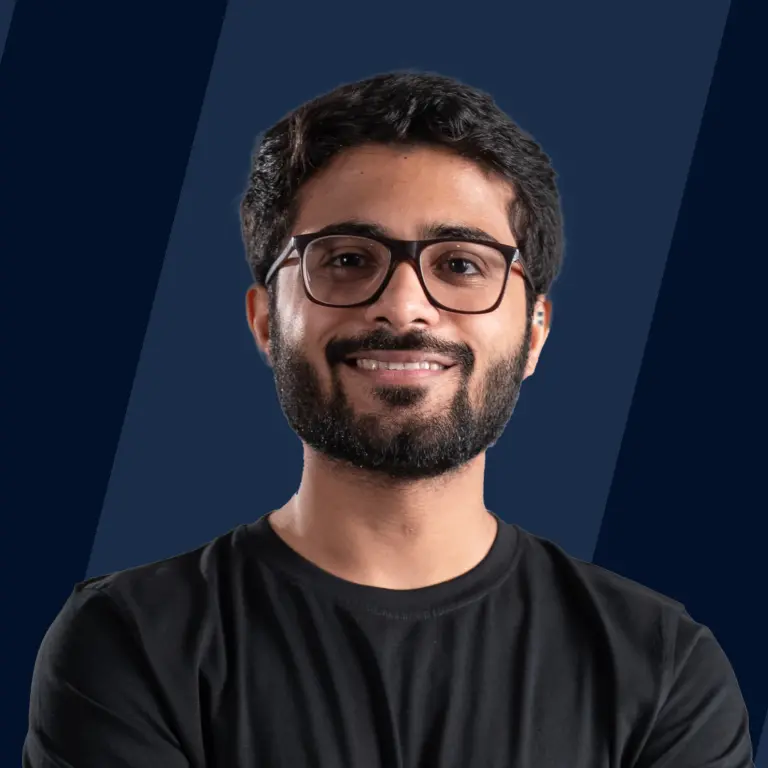
Overview
Callable interface in Java is used to make a class instance run as a thread by implementing it. The Callable interface is provided by the java.util.concurrent package. The Callable interface can return any object as a result and can throw an exception.
What is Callable Interface in Java?
Runnable Interface in Java
In Java, we can use threads to process time-consuming tasks parallelly and asynchronously. We can use the Runnable interface in Java to run a class instance in multiple threads. The skeleton for the Runnable interface is given below:
The Runnable interface has a single method run() that has to be overriden by the class that implements it.
In this example, we created a class Task that implements the Runnable interface and overridden the run() method. When a thread is created with the Task instance and executed (thread.start()), the run() method of the Task instance is called.
Limitations of Runnable Interface in Java
Below are the limitations of the Runnable interface:
- The run() method of the Runnable interface is of type void and cannot return any values.
- The Runnable interface doesn't allow to throw any checked exceptions (exceptions caught at compile time. Eg: Exception).
Callable Interface in Java
Callable interface was introduced to overcome the limitation of the Runnable interface. The Callable interface in Java has a single method call() that can return any object as result using generics. The class whose instance has to be run as a thread should implement the Callable interface and override the call() method. The skeleton of the Callable interface is:
Return Value of Callable Interface in Java
Unlike the Runnable, the Callable instance cannot be passed as an input while creating a thread. Instead, we must wrap it with a future (Eg. FutureTask) and pass it to the Thread class. The Thread returns a future with the result produced by the Callable instance. We can retrieve the result from the future. We will understand it with an example in the later Examples of Callable Interface in Java section.
Implementation of Callable Interface in Java
Below are the steps to implement the Callable interface in Java
- Create a class that implements the Callable interface.
- Override the call() method of the Callable interface in the class.
- Create a FutureTask object by passing the class object that implemented the Callable interface.
- Create a Thread object in the main class by passing the FutureTask object created.
- Call the start() method of the Thread object.
- Get the result returned by the callable class using the get() method of the FutureTask object.
Features of Callable Interface in Java
- The call() method of the Callable interface can return any objects.
- The call() method of the Callable interface can throw both checked (exceptions caught at compile time. Eg: Exception) and unchecked (exceptions occurring at runtime. Eg: RuntimeException) exceptions.
- The computation can be canceled using the cancel() method of the FutureTask object.
Examples of Callable Interface in Java
Consider we have a class MessageScheduler that prints the given message after the specified delay in seconds. We can schedule any number of messages using the MessageScheduler, and each message should be scheduled in a different thread. The scheduler should return true if the message is printed successfully; else, false. We can achieve this using the Callable interface as specified below:
MessageScheduler.java
The MessageScheduler class accepts message and delay as constructor parameters and implements the Callable interface. The call() method is overridden to print the given message after the given delay. The call() method returns false if there are any exceptions, else true.
Main.java
In the main() method, we schedule three messages with specific delays in seconds.
Output
Explanation
We pass the message and delay while instantiating the MessageScheduler instance. The call() method will be executed whenever the thread.start() is executed. The call() method returns true for the messages Hello and Hi and returns false for the message null because we are throwing NullPointerException if the message is null.
Conclusion
- The Callable interface in Java is used to make a class instance run as a thread by implementing it
- The Callable interface in Java overcomes the limitations of the Runnable interface.
- The Callable interface has a single method call that can return any object.
- The call() method of the Callable interface can throw both checked and unchecked exceptions.