capitalize() in Python
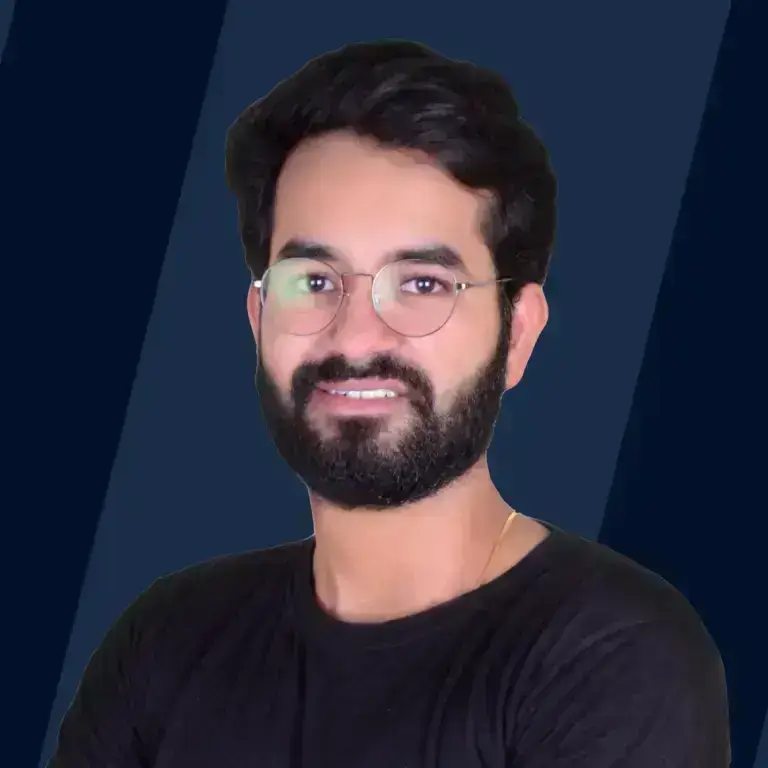
Overview
The capitalize() function in Python converts the first character of a string to uppercase and the remaining characters to lowercase. The function returns the updated string without modifying the actual input string.
Syntax
Parameters
The capitalize() function takes no parameters. We call capitalize() on the string or variable which we want to modify.
Return Type
The capitalize() function returns a string. It takes an input string, converts the first character to upper and the remaining character to lower case and then returns the modified string.
capitalize() does not modify the actual input string but returns a newly updated string.
:::
Example
Output
Explanation
In this example, we apply the capitalize() function on a string "pyThoN". It creates a new string that is stored in the variable capitalizedStr. In this new string, the first character p of input string inputStr is converted to uppercase, and the remaining characters yThoN are converted to lowercase. It results in our final output "Python". Note that the original string inputStr remains unchanged, and the output is returned in a new string capitalizedStr.
What is capitalize() in Python?
The capitalize() function in Python converts the first character of a given input string to uppercase. All the remaining characters of the string are converted into lowercase. This type of case conversion is similar to using the sentence case in word processors like MS Word and can be used to write headlines (or maybe fix a broken keyboard!!)
Examples of capitalize() in Python
We have already seen an example of converting a word using capitalize() in Python. Let us go through some more examples like converting a sentence, and non-alphabetical characters using capitalize().
Using capitalize() in a sentence
A major use case of capitalize(), as mentioned earlier, is in writing headlines and captions or in converting a mixed case text to a more readable sentence case text.
Code
Output
Explanation
Isn't it a little challenging to read the input string since it is written in a toggle case? To make this text more readable, we will use the capitalize() function in Python. Now, the first character of the sentence is converted to upper case, and the remaining characters are converted to lowercase, making our string more human-readable.
Remember that capitalize() converts only the first character of the string to uppercase. It is different from title case, which converts the first character of every word in a string to uppercase.
Exception: Using capitalize() when the first character is not an alphabet
Let us consider a different example where the string's first character is not an alphabet. Suppose a first character is a number or a special character. Now, the case of a special character cannot be changed. Do you think capitalize() will instead convert the first occurring English alphabet in the string to uppercase? In case you were wondering, the answer is NO!
It is an exception scenario where the first character will not be converted to uppercase. The other alphabets in the string (if any) will still get converted to lowercase, as expected.
Code
Output
Explanation
The first character # is not an alphabet and thus cannot be converted to uppercase. The remaining characters, although, will get converted to lowercase as expected. Similarly, if the string starts with a number, space or a newline character, the first character will not be converted to uppercase.
Alternative to capitalize() in Python
We can also imitate the functioning of capitalize() using the concept of string slicing and other Python functions like .upper() and .lower(). Let's see how!
EXAMPLE
Output
Explanation
We slice the input string using the subscript [0:1] to return its first character and apply the .upper() function in Python to convert it to uppercase. Next, we will fetch the remaining characters in the input string using [1:] and convert them to lowercase using the .lower() function. Finally, the two substrings are concatenated, and the output is stored in a new variable.
We know how the capitalize() function works internally, but it's advised to use the in-built capitalize() function to make your code more straightforward, concise, and easy to read.
Note: Even with the string slicing method mentioned above, we will still face the exception scenario where the first character will not be converted to uppercase if it is not an alphabet.
Conclusion
- capitalize() in Python is used to convert the first character of a string to uppercase and the remaining characters to lowercase.
- The capitalize() function takes no parameters.
- capitalize() does not modify the actual input string but returns a new updated string.
- Exception scenario: If the first character is a number, special character, space or a newline character, the first character will not be converted to uppercase. Although the remaining alphabets in the string will get converted to lowercase, as expected.
- We can imitate the functioning of capitalize() using the concept of string slicing and other Python functions like .upper() and .lower().