Close File Python
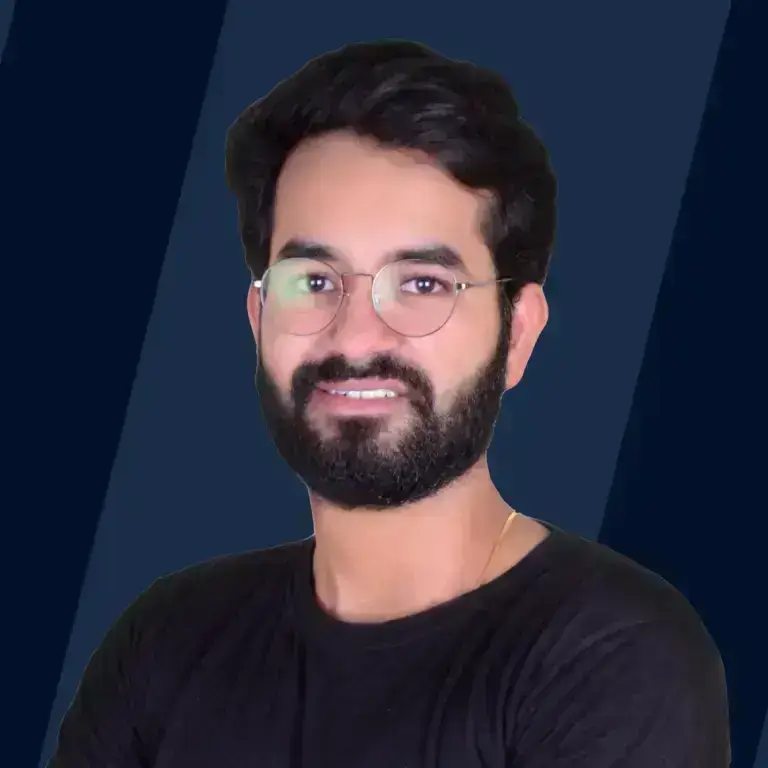
Overview
The close() file Python method can be defined as not closing the file in python which may lead to variability when running your scripts. Hence, implementing the close() file Python method over the opened file in python will help us to save the program resources which we shall be learning about with various code examples.
Syntax of close() file python
Below is the syntax for close() file python:
As seen above in the syntax, we close the opened file in Python by using the close() file Python method and specifying the file that we want to close.
Parameters of close() file python
As can be seen from the syntax that we studied above, there is no such parameter that we need to pass mandatorily.
Quick Note: When we open the files using the 'with' statement in Python we don't need to explicitly close the file. The file opened gets automatically closed without any manual intervention. The files can be opened using various modes like read, write, append, etc. after the operations are performed even if we move to another file and come back to the same file and perform some more operations. We shall see that no issue or error popups up. This happens as the file gets closed automatically upon no interaction with a file.
Return Value of close() file Python
The close() file Python method does not return any value. The return type for the close() file Python method is <class 'NoneType'> that is, it returns nothing. With the close() file Python, you can close the already opened file. Also, you can call the close() file Python more than once, but its important to note that when the close() file Python is used over an already closed file then you might encounter the ValueError exception that we shall be studying about as we travel through the article.
Exceptions of close() file Python
The exception that is encountered while working with the close() file Python is the ValueError: I/O operation on closed file. exception which is aroused when we perform the close() file Python over an already closed file. While the close() file Python method can be called more than once for an opened file but if any operation is performed over a closed file it shall raise a ValueError exception.
Let us dive into below code example to understand this exception:
Algorithm:
- We opened a file using the open() method in python.
- Then we read the file using the read() method in python.
- We move forward to the close() file python as shown in the code. We use the close() file python as we have limited resources that are being managed by the operating system. Hence, closing the files properly helps to protect against hard-to-debug errors like file handles running out or experiencing corrupted data.
- Finally, when we try to write into the closed file we encounter the exception.
Code:
Output:
Explanation: As seen above, we see that the ValueError exception is encountered when the write() method is applied over an already closed file (using the close() file python method). We see that we start by opening the file using the open() method in python and then reading the file using the read() in python. After this, we close the already opened file using the close() file Python method. After this, when we try to write in a closed file we encounter the ValueError exception. Also, we can call the close() function more than once as well. This won't throw any error too when the close() function is called over a closed file.
What is the close() file in Python?
While coding in Python, you might be interacting with python when you need to read, open, wrote, append, and close files in Python. With Python's inbuilt functions, you can easily start creating, writing, and reading files.
When you open a file to perform a certain function as per the scenario, it's important to note that closing a file after each use is important. When the file is not closed once the interaction is completed, this not only wastes the program resources but also prevents other file interactions that can sometimes also lead to inconsistencies when you run your Python scripts with different Python versions. As we have limited resources that are being managed by the operating system it's always best to close the file after every use.
It's always recommended to close a file while in some cases it's observed that due to buffering when changes are made to a file then it may not necessarily reflect until you have closed the file. Though with Python the file is automatically closed when the reference object of the file is allocated to another file, as a standard it is appreciated to close an opened file as it reduces the risk of being unwarrantedly modified or read. The close() file Python method can be called more than once but when any operation is performed on a closed file it raises a ValueError exception.
As there is always an upper limit to the number of files a program can open, it becomes important to explicitly close each of the open files, once the job with the open file is completed. If the limit to the number of files that can be opened is exceeded, there is no reliable way of recovery, causing the code program to crash.
The important point to note, the close() file Python method is not entirely safe as when an exception occurs while performing some operation with the file, then the code exits the programs without closing the file. hence, it is always better to use a try...finally block. When the with statement is used, then it's one of the safest ways to handle a file operation in Python as the with statement makes sure that the file gets closed when the block inside the with statement is exited.
Uses of close() File Python Function?
Talking about the uses of the close() File Python function, the method is used to close the opened file. Also, a closed file cannot be read or written, and doing so can raise errors like ValueError exceptions. We can also call the close() file Python more than once. Hence, it is a good practice to always use the close() file Python method to close a file.
The below diagram showcases the use of the close() file python function:
When we use the close() file Python method over a file object then it flushes any unwritten information and eventually closes the file object. This makes sure that no more writing can be done in that file.
Quick Note: When long and extra information is to be appended using this Python script to a log file having valuable customer data or data that might have taken months to web scrape, then in that case the file corruption would become a costly problem.
Why Close a File if Python Does it Automatically?
One question that might pop up in your curious mind, can be that when Python is flexible enough to close the file once the operations are performed then why is there a need to explicitly close a file?
When working with the with statement (The with statement in Python automatically closes the opened file when the interaction with that file has ended. This makes the code cleaner, simplifies the management of resources like file streams, and makes it much more readable as well.) keyword to open a file as shown below, once the operation is done then Python automatically closes the file when it finds that you left the indented region (source).
Code:
As seen above, you don't need to explicitly call the file.close() file Python whereas then it would be considered as bad style and redundant code. And hence, Python automatically closes the file for you.
Now, closing a file is not strictly necessary in all circumstances, but it's recommended when you are not using the with statements. Like when your code terminates, then the file is closed automatically by the Python runtime environment. This way Python will also close the file automatically when you reassign a referencing variable to another file. But when you don’t close the file in Python, then you must know that a lot can go wrong, as well.
Like you may write data into a file and assume that the data is now in the file. However, Python may buffer the data in the machine’s memory for a while before it flushes it into the file. But when you access and try to read the file content, you may observe the unmodified data. And this may then lead to an ambiguity that even after you wrote the new data into the file it's not visible yet.
Hence, close() file Python when you don’t need it. By closing the file, Python will flush all data in the temporary memory buffer into the file on the disk. This will make sure that all the system’s memory is released from handling the file which you just closed.
The File needs to be handled while working in Python:
More Examples
Let us dive into some scenarios where we shall see how we can use the close() file Python method with various coding scenarios:
Code Example 1
Our first code example would be when we use the close() file Python to simply close the open file.
Algorithm:
- We opened a file using the open() method in python.
- Then we read the file using the read() method in python.
- We move forward to the close() file python as shown in the code.
Code:
Output:
Explanation: We see that we start by opening the file using the open() method in python and then reading the file using the read() in python. After this, we close the already opened file using the close() file Python method. We see that once the file is closed, the message File is closed. gets printed.
Code Example 2
Our second code example would be to explain the problem of what happens when we are not closing the file correctly. For this, we open, read and try to append a line in the file which was previously not closed and so the append fails. Then, we also fix the issue by properly closing the opened file and then again opening the file to append the line.
Algorithm:
- We open the test_1.txt file which has the message - "This is the text of file 1." written in it using the read (r) mode.
- Then, we read the file into Python, after which we even display the text, and append a new line to the message using the append (a) mode.
- Then we incorrectly close the file by using the close() file Python function.
- Now as we have only closed the file after the most recent open file. We try to close each file individually at the end as a result we see that appending to the file from file_2 isn't getting reflected in file_3.
- We have potentially corrupted the file, which has prevented it from being read or used again in the future.
- Now, as we have seen the issue let us fix it by simply making sure to close the file after writing in it before trying to read it again as can be seen in the example.
Code:
Output:
Explanation: As seen above, we started by opening the 'test_1.txt' file using the read (r) mode. Then, we read the file into Python and append a new line to the message using the append (a) mode. Then, we purposely close the file incorrectly using the close() file Python function. We try to close each file individually at the end as a result we see that appending to the file from file_2 isn't getting reflected in file_3. We have potentially corrupted the file, which has prevented it from being read or used again in the future. Now, as we have seen the issue let us fix it by simply making sure to close the file after writing in it before trying to read it again as can be seen in the example.
Code Example 3
For this code example, we shall be exploring the most straightforward way to close the file by using the with statement whenever opening a file. This is a fundamental concept in Python and this pattern is also known as using a 'context manager'.
Algorithm:
- We now use the statement to open the file - test_1.ttxt in the read, append and read format. This code with the use of context managers that is the with statement gives the same functionality with less coding required.
- We use the open() statement as this will automatically close a file once the block code has been executed. We mostly prefer to use the with open() but with ranging scenarios you may also call for manually closing files using the close() file Python method.
- We then encounter the 'Valueerror' exception when we are working with context managers, as shown in the proceeding code that we get ValueError: I/O operation on closed file.
- As seen we shall get the error as we used the write() file method outside of the with open() function.
- We easily fix this error by maintaining everything under the with open() scope as shown below. And this way the script executes successfully, and can write the message to the file before closing it.
Code:
Output:
Explanation: As seen above, we started opening the file - test_1.ttxt in the read, append and read format using the with statement. This code with the use of 'context managers' that is, the with statement gives the same functionality with less coding required. We use the open() statement as this will automatically close a file once the block code has been executed. We mostly prefer to use the with open()but with ranging scenarios you may also call for manually closing files using the close() file Python method. The 'ValueError' exception that might be encountered when we are working with context managers is like ValueError: I/O operation on closed file.. We shall get the error as we used the write() file method outside of the with open() function. Then, the context manager automatically closes the file, and Python will not perform any subsequent file operations. We easily fix this error by maintaining everything under the with open() scope as shown below. And this way the script executes successfully and can write the message to the file before closing it.
Quick Note: By using the 'context managers' that is, the with statement gives the same functionality with less coding required. This automatically closes the file once the block code has been executed. hence, using the context manager frees us from remembering to close files manually, but also makes it much easier for others that are reading the code to see precisely how the program is using the file.
Conclusion
- The close() file Python method closes an open file. It's always recommended to always close a file while in some cases it is observed that due to buffering when changes are made to a file then it may not necessarily reflect until you have closed the file.
- The close() file Python method can be called more than once but when any operation is performed on a closed file it raises a ValueError exception.
- The close() file Python method is not entirely safe as when an exception occurs while performing some operation with the file, then the code exits the programs without closing the file. hence, it is always better to use a `try...finally block.
- The easiest and most straightforward way to close the file is by using the with statement whenever opening a file. This is a fundamental concept in Python and this pattern is also known as using a 'context manager'.