Java Command Line Arguments
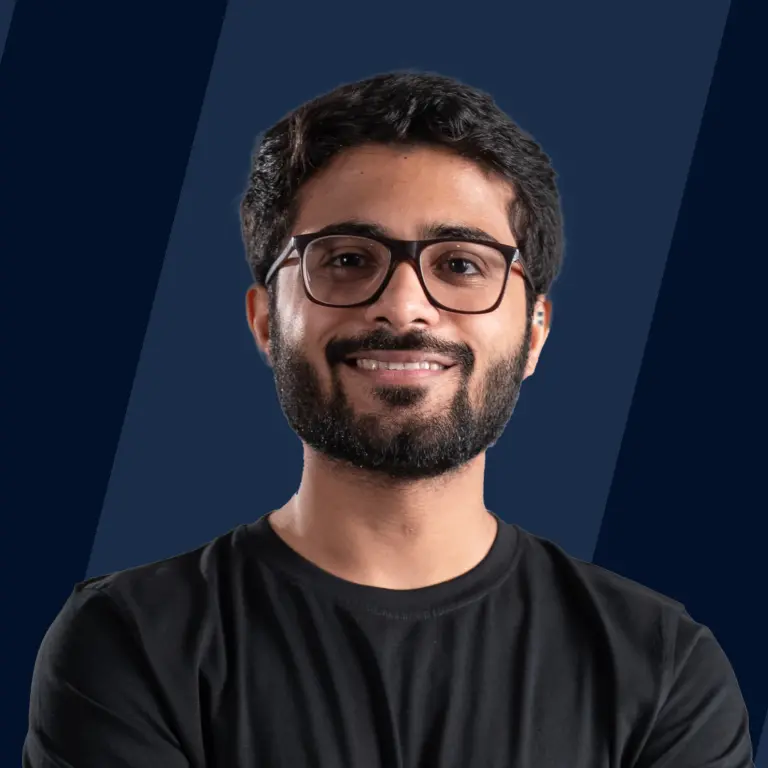
Overview
Command line Argument is a way to provide input to Java applications through the command line when compiling and running the program. These are the inputs provided after the execution command when running a Java program. They are passed to the main function as strings and stored in the args parameter.
Command line arguments are processed by the Java Virtual Machine (JVM) before the main function is invoked. They are bundled together and supplied to the main function as an array of strings.
Command line arguments are read as strings, and certain characters like #, %, and & may cause errors when entered as command line arguments.
Working of Command-line Arguments
There are various ways to provide input to our program. One of these ways is to provide input through the command line, which we also use to compile and run our program. These inputs are passed to the main function at runtime even before our main() begins to execute. The image below represents the two ways by which we can write our main function in Java. The argument String[] args or String… args can be broken down as follows.
In the context of command line arguments in Java:
- String: It represents the data type of the array elements passed to the main function. In Java, command line arguments are received as an array of strings. The String data type is used because Java commonly uses strings for input and output operations. Each command line argument is treated as a string, regardless of its original data type.
- args: The local name given to the array variable holds the command line arguments in the main function. It serves as a reference to the array, allowing access to and modifying the values stored in it. Using the args variable, the program can process and manipulate the command line arguments as needed during runtime.
The command line arguments that are given to the program are stored in these main() function parameters.
Java programs are compiled and run by:
Compile > javac filename.java
Run > java filename
To execute our program, we need to compile the .java file using the above-stated command. This converts our .java file to a .class file in a machine-readable language. Later, we execute this file using the run command.
If we provide inputs following this command, the JVM (Java Virtual Machine) wraps all these inputs together and supplies them to the main function parameter String[] args or String... args. This process occurs even before our main() is invoked. These inputs are referred to as command-line arguments. Since these inputs are passed to our application before its execution, they provide us with a way to configure our application with different parameters. This capability is particularly useful for efficient testing of our application.
To pass command line arguments in Java, follow these steps:
- Write your Java program and save it with the .java extension in your local system.
- Open the command prompt (cmd) and navigate to the directory where you saved your program.
- Compile your program using the command javac filename.java. This will convert the .java file to a .class file.
- Write the run command java filename and continue the command with all the inputs you want to give to your code.
- After writing all your inputs, run the command to execute your program.
The command line arguments given to the program are bundled and supplied to the main function parameter even before the invoking of the main function. These inputs are stored in a string array since Java deals with strings when performing I/O operations.The inputs are distinguished by the blank space ' ' between them. The command line reads one character at a time and stores it. When it encounters a space, it saves the already collected text as an input value and repeats the process until all characters are read.
Simple Example of Command-line Argument in Java
Output: Here, after our execution command the following text is given:
The figure below gives a step-by-step walkthrough of how the command line inputs are read.
As soon as the run command gets executed, the inputs following it are read as strings into the main function parameter.
This is done in such a way that the JVM reads every string individually when it encounters a character. As it reads a space character, it saves the string and starts with another string. This restricts the user from entering sentences as a command line input.
More Examples of Command Line Arguments in Java
The command line inputs are read as strings by the compiler and stored in an array. We can modify them by using the reference operator '[]'. The following program shows an example where we read the command line arguments and later modify them.
Here, the input is accessed using the ‘[]’ operator, and then we assign a new value to it. This assigned value overwrites the previous input.
Code:
Output:
Conclusion
- Command line arguments in Java provide an alternative way for users to input data into our programs.
- They are easy to access and manipulate, similar to normal arrays. Command line arguments can be passed as strings to the main() function parameter and are useful for testing and running applications.
- These inputs, which are provided before execution, allow us to initialize our application with data values of our choice, providing a method for easy initialization.
- Certain characters like '#', '%', and '&' may cause errors when entered through the command line.
- Command line arguments are passed as strings to the main function parameter: args.
- The command line reads spaces, separate characters one by one, and input values. Each time a space is encountered, the collected text is saved as an input value.