Constructor in C++
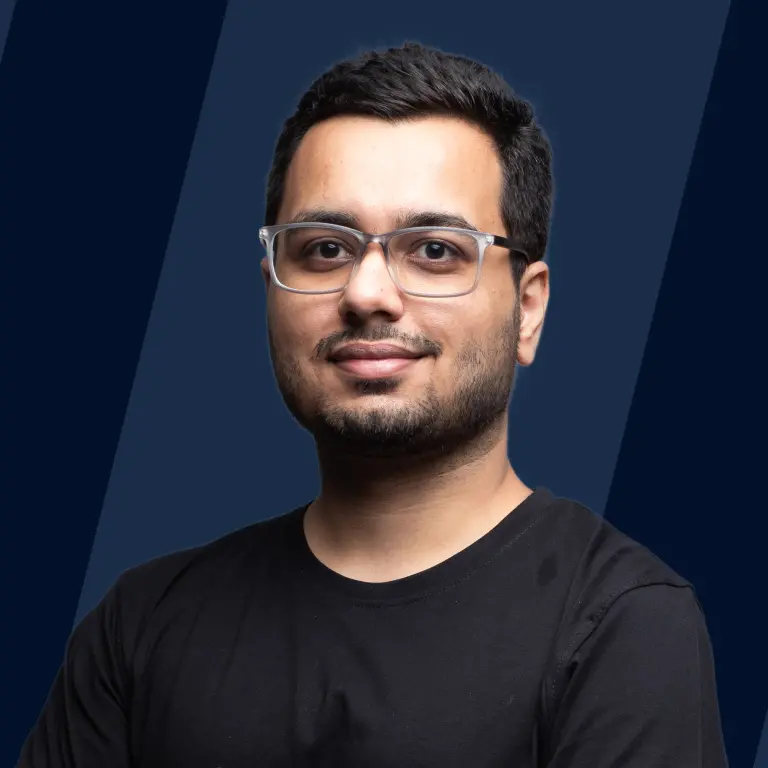
Overview
Constructors are essential in C++ for initializing objects within a class. They are special member functions that are in charge of establishing an object's initial state. Defining constructors may appear difficult at first, but it is an essential concept to learn.
Constructors in C++
Constructor in C++ is used for initializing objects within a class. They are special member functions that establish the initial state of an object. We can define a constructor in C++ as A special member function that is automatically called when an object of a class is created. It has the same name as the class and is used to initialize the object's data members or perform other setup operations.
How to Define Constructors?
To define a constructor in C++, we use the same name as the class, but without specifying a return type. When an object is created, it is automatically invoked to ensure appropriate initialization. Consider the class "Car" having properties like model, colour, and year. This class's constructor might look like this:
Example
The constructor in this example initializes the attributes using the values supplied during object creation.
What Makes a Constructor Unique From a Regular Function?
Constructor in C++ vary from regular functions in that they are called automatically when an object is instantiated. They ensure that an item is always in a valid state. Constructors, unlike functions, do not have return types, emphasizing their primary duty of initialization.
Characteristics of a Constructor
Constructor in C++, the must-learn concept of programming, plays a critical role in creating object behaviour and lifespan. Let's look at what distinguishes these code architects in their drive to initialize and set the scene for objects in a program.
- Initialization: Constructors guarantee that things begin their journey with preset values through our provided parameterization.
- Same Name as the Class: A distinguishing feature of constructors is that they have the same name as the class to which they belong.
- No Return Type: They're not like regular actions because they don't give back any results. Instead, their main job is to set up or create an object, putting it in a good starting state. So, while normal actions are all about getting things done and producing outcomes, constructors are more about the process of getting an object.
- Default Constructors: Constructors are adaptable, easily assuming the function as default constructors when no explicit constructor is given. This backup system ensures that objects are never left stranded in the absence of an initiation ceremony.
- Overloading Capabilities: Constructors demonstrate versatility by overloading, and supporting different parameter sets. This feature allows developers to customize object generation depending on unique requirements, providing flexibility to the building process.
- Inheritance Impact: Constructors also have an impact on the inheritance. During object creation, derived classes execute constructors not only from their class but also from their descendants.
Types of Constructors
A constructor in C++ is essential in programming, as it allows initializing objects within a class. Consider them as the architects of your code, in charge of laying the groundwork before your programme comes to life. Let's look at the different sorts of constructors and their duties.
1. Default Constructor:
In C++, a default constructor is a constructor that doesn't take any parameters. If a class does not have any constructor defined, the compiler automatically generates a default constructor for that class. The default constructor is responsible for initializing the object's data members to reasonable default values.
2. Parameterized Constructor:
This constructor allows you to pass arguments during object formation, allowing you to customize the initialization process based on your needs.
3. Copy Constructor:
The copy constructor in C++ is used to create a new object by copying the values of an existing object. It's similar to generating a clone while retaining the original's qualities.
Constructors are object-oriented programming's critical concept. Understanding these kinds gives you the ability to design your objects for maximum efficiency and functionality.
Please click here to discover more about the types of constructors.
Advantages of Constructors
Constructors are important in C++ programming because they provide various benefits that improve code functionality and maintainability. Here's a summary of their main advantages:
- Object Initialization: Constructors guarantee that objects are properly initialized, allowing you to provide default values and create a legitimate state for the object right from the start.
- Default Values: They allow you to provide default values for class attributes, which simplifies object creation and eliminates the need for manual initialization.
- Code Clarity: Constructors make code easier to comprehend by centralizing object initialization logic. This not only clarifies the code but also minimizes repetition and the possibility of mistakes.
- Overloading: C++ provides constructor overloading, which allows several constructors with differing argument lists to be created. This adaptability allows for a wide range of use cases and promotes code flexibility.
- Inheritance Support: Constructors make inheritance easier by guaranteeing that base class constructors are called appropriately when derived class objects are created. This contributes to the class hierarchy's integrity.
- Memory Allocation: Constructors enable dynamic memory allocation, allowing for heap-based object creation. This adaptability is critical for dealing with complicated data structures and optimizing resource usage.
- Initialization Lists: The constructor's initialization list feature enables efficient initialization of member variables, which contributes to higher speed and code efficiency.
Finally, constructors in C++ greatly contribute to code organization, readability, and flexibility, making them an essential component for efficient object-oriented programming.
Limitations of Constructors
Constructors are important in C++ for initializing objects, but they have limits. Let's go over these constraints one by one:
- No Return Type: Constructors lack a return type, making it impossible to return values. This constraint limits its applicability in some dynamic initialization settings.
- Single Invocation: Unlike conventional functions, constructors may only be called once during object formation. This constraint might cause difficulties when attempting to reinitialize an object after it has been created.
- Inability to Allocate Memory: Constructors are unable to allocate memory dynamically. Additional methods or operators are required if your class requires dynamic memory allocation, which adds complexity.
- Limited Overloading: Overloading constructors is a strong feature, but the parameter types or numbers must differ. In some circumstances, this constraint may limit the flexibility of constructor overloading.
- No Constructor Inheritance: By default, derived classes do not inherit constructors from their base classes. Because constructors must be explicitly declared in the derived class, this might result in repetition.
Understanding these limits aids in the creation of strong and adaptable C++ programs that make appropriate use of constructors while reducing their inherent constraints.
FAQs
Q: In C++, what is a constructor?
A: In C++, a constructor is a specific member function that is called when an object is formed. It initializes the data members of the object and allocates resources.
Q: What distinguishes a constructor from a regular member function?
A: Constructors have the same name as the class and are automatically called when an object is created. They initialize the object, whereas regular member functions are expressly invoked and execute specified responsibilities.
Q: Can a C++ class have multiple constructors?
A: Yes, several constructors with separate argument lists can exist in a C++ class. This is known as constructor overloading because it allows for greater object construction flexibility.
Q: What is the purpose of a copy constructor?
A: The copy constructor is used to create a new object by copying the values of an existing object. When an object is supplied by value, returned by value, or expressly called to generate a copy, it is invoked.
Conclusion
- Constructors are essential in C++ for initializing objects during their construction, ensuring that objects begin with valid and intended states.
- One of the most important characteristics of constructors is their automated invocation during object formation. This automated setup speeds up the coding process and eliminates mistakes.
- Constructor overloading is the ability to create numerous constructors within a class in C++. By offering alternative parameter lists, developers may respond to a variety of object generation circumstances.
- The copy constructor in C++ is designed primarily for making copies of things. It assures data accuracy when objects are supplied or returned by value, or when explicit copy creation is necessary.
- Constructors help to reduce code complexity by enclosing initialization logic within the class. Following encapsulation and abstraction concepts improve code maintainability and readability.