What are the Types of Constructor in C++?
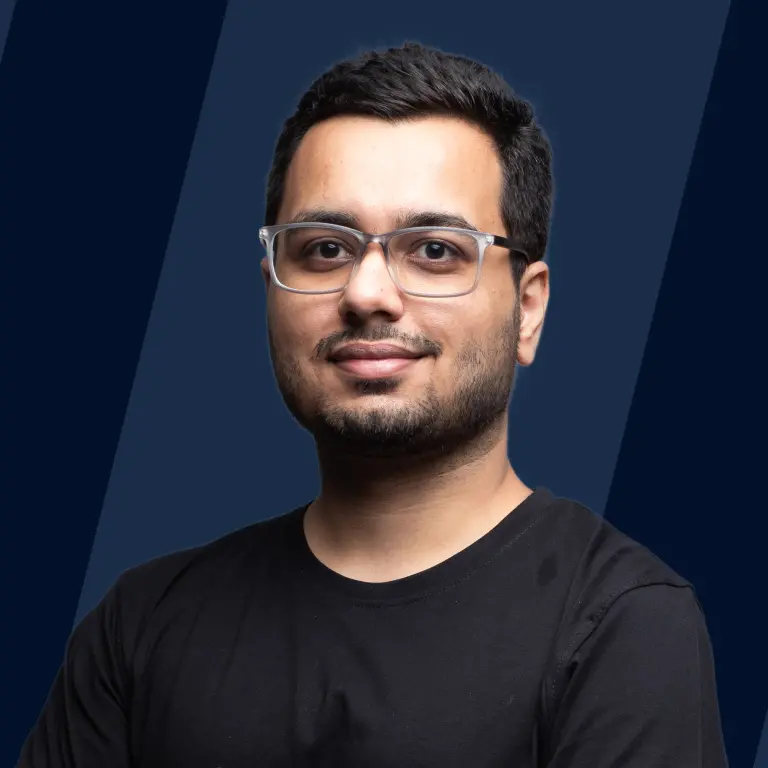
A Constructor is a special type of member function defined in the public scope of a class whose main task is to initialize the class object after its instantiation. The constructor is named as such because the constructor function constructs the values of class data members. A constructor gets automatically invoked at the time of object creation, and as soon as the constructor is invoked, it initializes the object member functions.
There are mainly three types of constructors in C++: -
- Default Constructor
- Copy Constructor
- Parameterized Constructor.
Apart from these three, there are three more types of constructors in C++:
- Dynamic Constructor
- Conversion Constructor
- Explicit Constructor.
Default Constructors
The default constructor is the most basic type of constructor. We can define the default constructor as the constructor that does not take any argument. The default constructor is also known as the no-parameter constructor.
The default constructor is one of the most important constructors. If we do not explicitly create any type of constructor in C++, then the compiler automatically creates (or rather generates) a default constructor for the particular object.
The default constructor is used to initialize all the class elements at the time of object creation.
Syntax:
Let us take an example to understand the working of the default constructor.
Output:
Parameterized Constructors
Parameterized constructor is a type of constructor in which we provide the parameters.
So, we can define the parameterized constructor as the constructor which can take the arguments that help in object initialization.
The parameterized constructor is used to initialize all the class elements at the time of object creation with the values present in the argument.
i) Uses To use a parameterized constructor, we add the parameters to the constructor function the way we would to any other function. When we define the constructor’s body, we can use the constructor's parameters to initialize the object.
We can also use the parametrized constructor to overload the constructor. We can use the parameterized constructor to initialize the various data members of the class with different values when the objects are created.
Syntax:
Let us take an example to understand the working of the parameterized constructor.
Output:
Note: When we define one or more parameterized constructors, a default constructor for the same class should also be defined explicitly, as the compiler will not provide any default constructor in such cases.
Copy Constructors
As the name suggests, a copy constructor is a constructor that initializes an object using another object of the same class. We usually use the copy constructor to copy data from one object to another.
Note:
- Similar to the default constructor, when the programmer defines no copy constructor, the compiler creates its copy constructor.
- It would be infinitely recursive if we passed the object by value. So, we pass on the object reference.
In simple terms, a copy constructor is a parameterized constructor with a class object as its parameter. Using the copy constructor, we can initialize an object with another object of the same type.
The copy constructor is used to initialize the class elements with the copy of the same class object.
Syntax:
Let us take an example to understand the working of the copy constructor.
i) Types of Copy Constructors
We can further categorize the copy constructor into two categories:
a. Default Copy Constructors (Does Shallow Copying) Similar to the default constructor (created by the compiler if the programmer has not created one), the default copy constructor is created by the compiler if the programmer has not created any default copy constructor.
The default copy constructor performs shallow copying. Shallow copy is copying an object into a new object whose instance variables are identical to the old object.
b. User-Defined Copy Constructors (Does Deep Copying) Similar to the parameterized constructor, a programmer defines user defied copy constructor.
The user-defined copy constructor performs deep copying. Deep copy creates an object by copying all the member variables with the same memory resources and the same value to the object.
d. Dynamic Constructors
We can also use constructors to allocate memory to the object while creating the objects. For the same purpose, we use default constructors. We can define the default constructor as the type of constructor that allocates the memory to objects at the time of their construction.
The new operator is used in C++ to allocate memory dynamically to any variable or object.
Syntax:
Let us take an example to understand the working of the dynamic constructor.
Output:
e. Conversion Constructors
The compiler uses the conversion constructors to perform the implicit class-type conversions. Conversion constructors are used to converting its parameter into a type of class.
If a class has a single parameterized constructor, then this constructor becomes a conversion constructor because such a constructor allows automatic conversion to the constructed class.
Syntax:
Let us take an example to understand the working of the conversion constructor.
Output:
f. Explicit Constructor
As we have seen in the previous section, if a class has a single parameterized constructor, then this constructor becomes a conversion constructor because such a constructor allows automatic conversion to the class being constructed.
We can avoid such implicit conversions by the compiler as the implicit conversion may lead to unexpected results in some cases. So, we use explicit constructors in such cases.
We can make the constructor explicit with the help of the explicit keyword. The explicit keyword tells the compiler not to convert types implicitly in C++.
Conclusion
- A constructor is a special type of member function defined in the public scope of a class whose main task is to initialize the class object after its instantiation.
- The name 'constructor' is given because the constructor function constructs the values of class data members.
- A constructor gets automatically invoked at the time of object creation.
- The constructor's name is the same as the class name as it helps the compiler differentiate the constructor from other member functions.
- A constructor function does not return anything.
- We cannot inherit constructors in C++, but a derived class or child class can invoke the parent class's constructor.
- A constructor can be defined inside or outside the class definition, but we cannot reference the address of a constructor.