Classes and Objects in C++
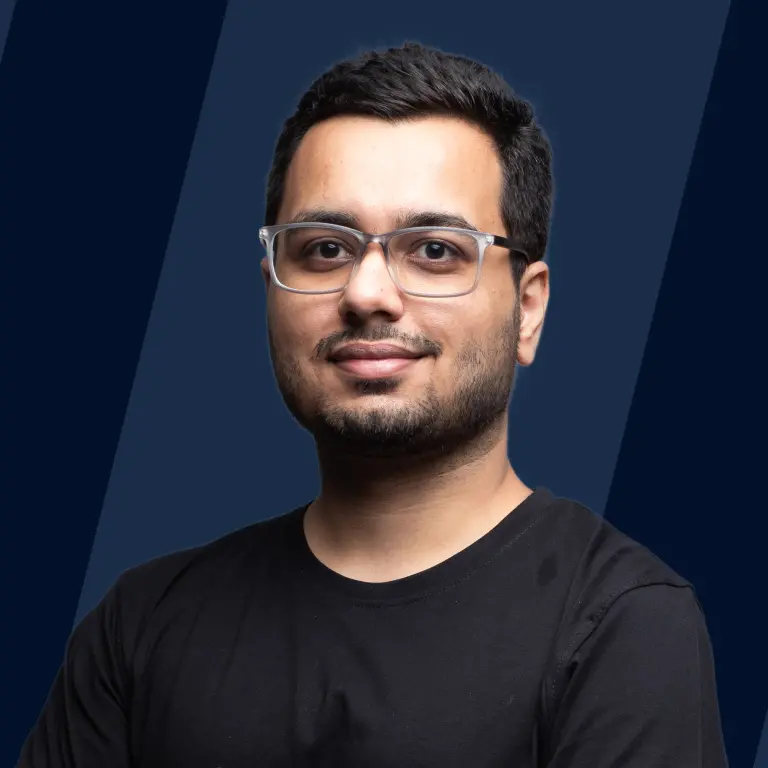
Overview
C++ is an object-oriented programming language that is used to model real-world entities into programs. Object-oriented programming languages achieve this using classes and objects.
Class in C++
A class in C++ is a user-defined type or data structure declared with a keyword class that has data and functions as its members. A class can be used by declaring an instance of that class which is nothing but the object in C++.
In real life, we see dogs with different breeds or classes but there are some common characteristics such as they have tails, they bark, they sniff, etc. So, instead of creating different classes for every dog, we can define a single class with common properties of dogs. Hence, Classes act as a user-defined data type to create objects with similar properties.
Defining Class in C++
A Class is defined by the keyword class followed by a class name (user's choice) and a block of curly brackets with a semicolon after the block. The block starts with access specifiers followed by data members and member functions.
Note: Access specifier defines how the members of the class can be accessed. In C++, there are 3 types of access specifiers: public, private and protected.
- public: members can be accessed outside the class.
- private: members cannot be accessed outside the class.
- protected: members cannot be accessed(viewed) from outside the class, but can be accessed in inherited classes(sub classes).
Syntax:
Lets take an example of Dog class having data members breed and color followed by member functions.
Object in C++
A class is merely a blueprint of data. An Object is a data structure that is an instance of a class. So when a class is created no memory is allocated but when an instance is created by declaring an object, memory is then allocated to store data and perform required functions on them. We can visualize trees, birds, and dogs as different classes and their instances like neem tree, peacock, and German shepherd dog respectively. Hence, classes and objects in C++ are the main ingredients of object-oriented programming.
Declaring Objects in C++
When a class is defined only the blueprint of data structure is defined no memory is allocated. To use data and its member function we can declare objects. We can declare objects by mentioning the class followed by user-defined object name.
Syntax:
Example:
Access modifiers
Now, we will learn about the access modifiers in detail. Data hiding is one of the most important characteristics of object-oriented programming languages like C++.
Note: Data hiding refers to limiting access to a class's data members. This is done to keep other functions and classes from messing with the data in the class. However, some member functions and member data must be made public so that hidden data can be modified indirectly.
C++'s access modifiers let us choose which class members are available to other classes and functions and which aren't. In C++ there are 3 access modifiers:
- Public: As the name suggests a public data member can be accessed by anywhere in the program by (.) operator.
- Private: Private data members cannot be accessed from outside the class. We need to use some special member functions of that class to access the data members called getters and setters. We will discuss these member functions later in this article.
- Protected: A protected member variable or function is similar to a private member, but it has the added benefit of being accessible in child classes or subclasss derived from the class.
Accessing Data Members
Access to a data member is solely determined by the data member's access control.
-
If the *data member is public, the object of that class can readily access it using the direct member access (.) operator. Let's take a C++ program to understand accessing of public data members:
-
If the data member is set to private or protected, we can't access the data variables directly. Then, in order to access, use, or initialize the private and protected data members, we'll need to construct unique public member functions. These member functions are known as getter and setter functions. Lets take an example of another class:
Here we can access the data members indirectly by the help of getter and setter like getBreed() and setBreed respectively.
Protected data members can be simply accessed by the (.) operator by the subclass of the class; whereas for a nonsubclass, we have to implement getters and setters in the same way as we accessed private data members above.
Member Functions in Classes
Member functions of a class are used to access, use, or modify the data members of that class. We can define member functions in two ways:
-
Inside Class definition: We can define a member function inside the class directly without declaring it first in class.
-
Outside Class definition: To define a member function outside the class we need to declare it first inside the class and then define it outside the class according to following syntax:
Example:
Objects as Function Arguments
To pass an object to a function we pass the object in the same way as we pass any other primitive data(int, string, etc). We just mention the object in the function's argument and call the function. Syntax:
Example: Here, we are printing all the properties(breed, color) of object dog by passing it to printProperties() function.
Constructors in C++
Constructors are special member functions in C++ that are used to initialize objects of a class. They share the same name as the class and do not have a return type, not even void. Constructors are automatically invoked when an object is created.
Types of Constructors:
- Default Constructor: It does not take any arguments. If no user-defined constructor is provided, the C++ compiler supplies a default constructor.
- Parameterized Constructor: It takes one or more arguments to initialize an object in a specific manner.
- Copy Constructor: It is used to create a copy of an existing object of the same class.
Example:
Destructors in C++
Destructors are also special member functions in C++ that are used to deallocate memory and perform clean-up activities for objects. Like constructors, they share the same name as the class, prefixed with a tilde ~, and they do not have a return type. A class can have only one destructor, and it is automatically invoked when the scope of the object ends.
Example:
In the example above, the constructor is called when the object d is created, and the destructor is called automatically when the program exits the main function, causing the object d to go out of scope.
Conclusion
- A class in C++ is a user-defined type that encapsulates data for the object and functions that operate on that data. It acts as a blueprint for creating objects.
- Objects are instances of a class. When a class is defined, no memory is allocated until objects of the class are created. Objects allow for the manipulation of the data within a class.
- These define the visibility and accessibility of class members. Public members can be accessed from anywhere, private members can only be accessed within the class, and protected members can be accessed within the class and its subclasses.
- Public data members can be accessed directly using the direct member access operator (.), whereas private and protected data members should be accessed using getter and setter functions.
- Functions defined inside or outside a class that operate on the data members of the class.
- Objects can be passed to functions in a similar manner as primitive data types.
- Special member functions for initializing and cleaning up objects. Constructors are called when an object is created, and destructors are called when an object goes out of scope or is explicitly deleted.
- Memory for objects is allocated when they are created and deallocated when they go out of scope or are explicitly deleted.
- Classes and objects promote the principles of object-oriented programming, leading to code that is more modular, reusable, and easier to maintain.