C++ Coding Standards
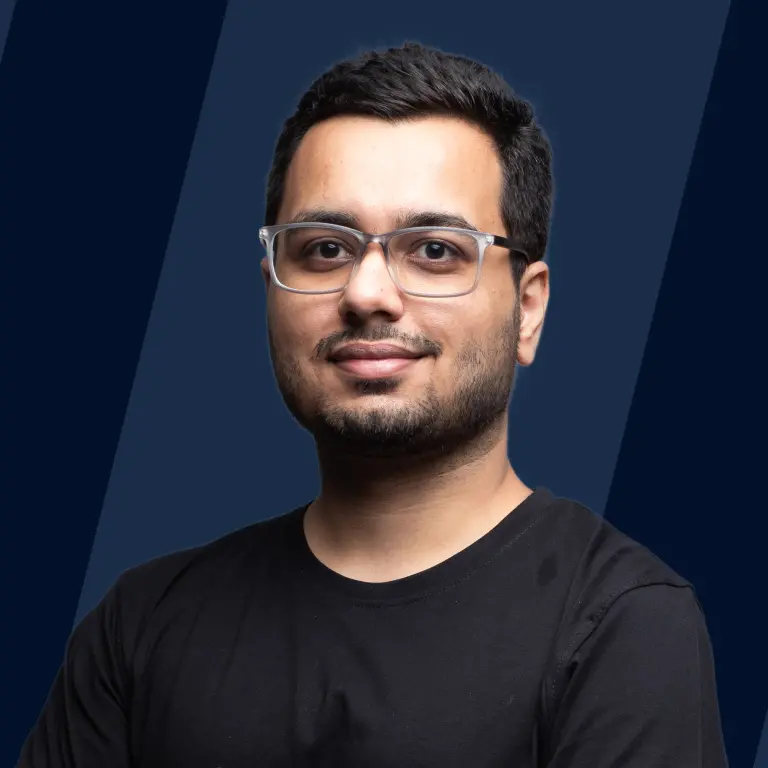
The formatting aids is used in the efficient reading of code. It appears more ordered, and when someone examines the code, it helps to give a positive impression of the coder. Coding standards can be defined as sets of rules and principles that define a programming language's programming style, techniques, and methods.
Coding Standards: Is It a Necessity?
C++ formatter is a tool or piece of software that allows you to format and beautify C++ source code in a preferred way. Many coding style schemes are provided by C++ formatter software, which aids in the formatting of source code with a suitable indentation in different styles as per the programmer's individual requirements. In the market, such code formatters are also known as beautifier tools. Formatting source code is essential because it facilitates learning and improves bug hunting, which saves time & expense.
Good C++ Coding Standards
Header Files
Header files play an important role in C++ Coding standards. Every .cc file should, in general, be accompanied by a .h file in C++. Unit tests and small .cc files with only a main() method are examples of common exceptions. The use of header files can dramatically improve the readability, size, and speed of your code. To learn more about Header Files in C++, click here.
Self-contained Headers
Header files should always be self-contained (i.e., they should compile by themselves) and terminate in .h. Non-header files intended for inclusion must end in .inc and must be used sparingly.
Each header file should stand alone. Users as well as refactoring tools should not be required to meet any particular requirements in order to incorporate the header.
Header files should always be self-contained (i.e., they should compile by themselves) and terminate in .h. Non-header files intended for inclusion must end in .inc and be used sparingly. Each header file should stand alone. Users and refactoring tools should not be required to meet any particular requirements to incorporate the header.
Template and inline function definitions should be kept in the same file as their declarations. These construct definitions must be included in every .cc file that utilizes them, or the program will fail to link in some build setups. If declarations and definitions are in separate files, the former should contain the latter by default.
The #define Guard
To avoid multiple inclusion, #define guards should be used in all header files. The indicator or the symbol name should be in the format <PROJECT>_ <PATH>_< FILE> _H_.
To ensure uniqueness, they should be established on the whole path in a project's source tree. For example, in project foo, the file foo/src/bar/baz.h must have the appropriate guard:
Include What You Use
If a source file or header file refers to symbols that have been defined somewhere, it should include a header file that is intended to get a declaration or description for that symbol. For any other reason, header files should not be included.
Use transitive inclusions sparingly. This allows people to remove #include statements from their no longer needed headers without breaking clients.
Forward Declarations
The forward declaration informs the code that follows that there are classes with the name Hello. When the compiler reads these names, it is satisfied. The linker will later locate the class definitions.
Inline Functions
Usually define methods inline if they are minimal, such as 10 lines or less.
The programmer can declare methods in such a way that the compiler expands them inline instead of invoking them through the standard function call method.
As long as the inline method is short, inlining a method can lead to a more efficient object code. Accessors as well as mutators, and also other small, performance-critical functions, are welcome to be inline.
Everything has its own pros and cons. Let's discuss some of the disadvantages of Inlining. Inlining too much can actually slow down programs. Inlining a function can raise or decrease the size of the code depending on its size. Inlining a tiny accessor function will usually reduce code size, however, inlining a large function will usually increase code size substantially. Check out this article to learn more about Inline Function in C++.
Names and Order of Includes
Most programmers prefer clean code with a set of habits or conventions to follow. The arrangement of #include directives is one such rule/habit.
Headers should generally be added in this particular order: related headers, C system headers, C++ standard library headers, headers from several other libraries, and headers from the programmer's project.
Without using UNIX directory aliases, all of a project's header files should be shown as descendants of the project's source directory...(the present directory) or..(the parent directory).
The most common order of includes used by programmers is as follows :
- Local headers
- Third-party headers
- STL headers
Scoping
The scope of a piece of code or a variable refers to the program component(s) in which that piece of code or variable can be accessed.
A language's scope rules are defined as the rules that determine which sections of the program a specific piece of code or data item is known and can be accessed.
In C++ coding standards, there are four different types of scopes:
-
Local Scope: Any variables declared in the local scope are local to that block and can only be utilized in that block and the blocks below it.
-
Function Scope in C++: Variables that are declared in a function's outermost block have method scope, which means they can only be accessible within the method that defines them.
-
File Scope in C++: A name declared outside of all blocks as well as functions has file scope. This means it can be used in any of the blocks as well as functions in the file where it is declared.
-
Class Scope in C++: A class member's name has the class scope and is unique to that class.
Namespaces
Let's go through a few important things to be kept in mind while using namespaces.
-
Namespaces contents are not indented.
-
We need to put the namespace as open curly '{' on the same line as the keywords namespace.
-
Place the close curly '}' of the namespace on its own line.
-
Place the comment at the end of the namespace curly.
-
A named namespace needs to be written in the following format: // namespace: name-of-namespace.
-
An unnamed namespace can be written in a simple way: // namespace
Class Definition
-
We can divide the sections into three categories: public, protected, as well as private, and each is indented four spaces.
-
There is no indentation between the public, protected, and private keywords as well as the class.
-
On the same line, use the keywords public:, protected:, private:.
-
There are no spaces between the colon and the BaseClassName, let's say for example: class NewClass: public Abc
-
Class NewClass: public Abc, for example, should have proper spacing between the colon as well as the access control keywords.
-
If the base class name and the subclass name do not fit on the same line, then break the lineup.
Class Constructor Initializer Lists
In C++ coding standards, an Initializer List is utilized in initializing the data functions of any given class. The list of functions or members which need to be initialized can be indicated with the constructor in the form of a comma-separated list further followed by a colon.
Lambda Expression
A lambda expression is defined as a small piece of code that accepts an input and produces a value. Lambda expressions are defined as identical to methods, except they don't require a name and can be used directly within a method's body.
A single parameter plus an expression make up the most basic lambda expression:
Need for C++ Formatters in Source Code
C++ formatter is a tool or piece of software that allows you to format and beautify C++ source code in a preferred way. Several coding style approaches are provided by C++ formatter software, which aids in the formatting of source code with a suitable indentation in different styles as per the programmer's individual requirements.
In the marketplace, we can call these code formatters beautifier tools. Formatting source code is essential because it facilitates learning and improves bug hunting, which reduces time and expense.
Let's try to understand the need for formatters in C++ with the help of a code where we add two integer values.
The given code is a straightforward addition of two integers. However, the manner in which it is written makes it unpleasant or difficult to comprehend. Correct indentation and sufficient spaces are required in the given code written above. After the bracket '{', the code should be formatted and indented properly to demonstrate that the required code block is included.
The goal of code formatting is to produce a consistent style format for your programs, not to limit your programming. This will assist you in debugging and maintaining your programs, as well as assisting others in comprehending them.
Maintaining a consistent style is vital as your applications expand in length and complexity. Proper formatters will benefit you in the long run. Any program that does not follow this style standard will have points subtracted from it.
Types of C++ Formatters
There are various types of formatters/beautifiers currently on the market for formatting C++ source code. When working on real-time projects, proper formatting and indentation are essential since they aid in a clear comprehension of the code and make it easier to detect problems and buried errors, particularly syntactical ones. It also aids the maintenance team in correctly maintaining the code and moving forward in a timely manner.
-
Artistic Styler - Artistic Styler is a well-known formatter as well as a beautifier for indenting C, C++, CLI, as well as Java source code. Artistic Style was created in April 2013 to address the issue of numerous formatters putting spaces in place of tabs (inability to discern between tabs and also the spaces in source code).
It's written in the programming language C++ and can appropriately re-format and re-indent the source code of a variety of languages. It can be used directly as a command line by programmers/testers, or it can be integrated into an existing program's library. This beauty tool is compatible with Windows, Linux, and Mac.
-
Jindent - The second C++ Formatter is Jindent. It is known to be one of the most prominent and widely used tools for improving the quality of Java, C, and C++ source code. It is responsible for the indentation of the code automatically as per the syntax as well as correct coding norms, which aids in the detection of flaws and saves time.
One of Jindent's great characteristics is that it has plugins for practically all common IDEs, such as Visual Studio, Eclipse, Netbeans, and others, so programmers and testers can utilize them. It is possible to call it from shell scripts. Jindent supports all operating systems, including Windows, Mac, and Linux.
-
Highlighter - Third is the Highlighter which is one of the most widely used formatters for languages such as C++, Perl, HTML, and a variety of other languages' source code. It is incredibly user-friendly and thus straightforward to operate. To have the needed formatting, users simply copy the source code into the desired text box, select the programming language C++, and select the Style selection.
It also has some unusual capabilities, such as the ability to see line numbers on the left side of the code and the ability to simply put the source code into an HTML page without having to add any extra CSS or JavaScript files.
Conclusion
You can use code formatting to communicate your intent to a reader in a variety of ways. The formatting of your code is frequently the reader's first experience with your system. It is deserving of our attention and concern. Code formatting enables -
-
Quick readability: The reader should be able to see the code's overall structure at a glance. The forms are created by text blocks to aid in the communication of the overall structure.
-
Detailed readability: The reader must be able to decipher a large amount of code.
-
Length: To make browsers as tiny as feasible, the number of lines must be kept to a minimum.
-
Width: The code's width must be kept to a minimum. Ordinarily, code should not spill all across the right margin, necessitating horizontal scrolling or breaking the text's shape using line-wrapping.