Multiset in C++
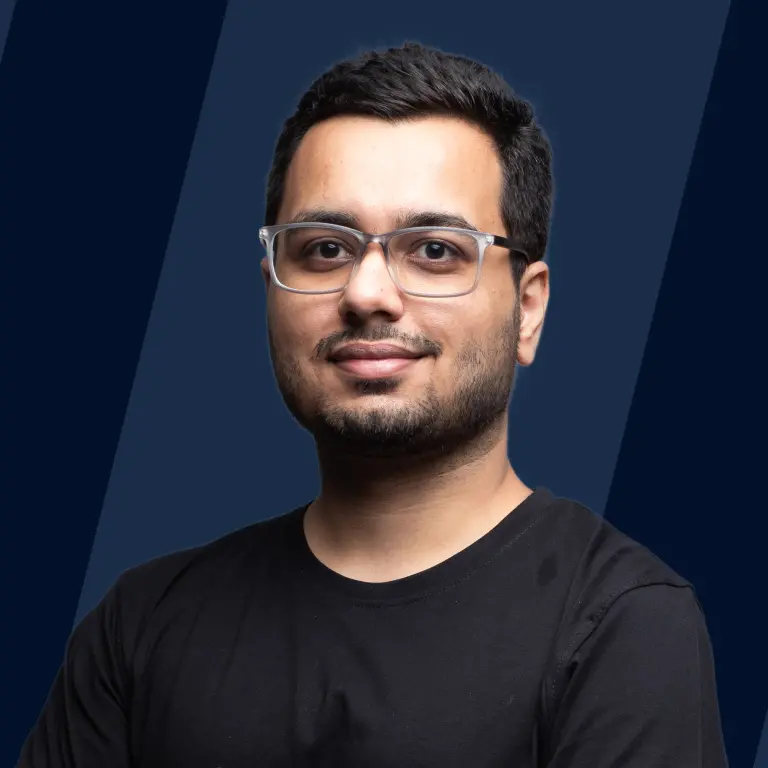
A Multiset in C++ is a container storing elements in a predefined order and allowing multiple elements to have the same value. The value of an element also identifies it in a multiset (the value is the key of type T). Multisets allow you to store elements in a specified sequence, with more than two items or many elements having comparable values. These values in the multiset identify the elements with their key value and pair value, and they cannot be changed once they have been placed into the multiset. However, one requirement can be met: more items can be inserted and retrieved at any time.
C++ Multiset Declaration
Syntax:
Where T = multiset:: which defines the type of value or the key type
When working with multiset in C++, it's critical to include the set's header file.
#include <set> to make all multiset methods work.
If you need the sorting features of a set but also want to allow repetitions in the container, utilize the multiset class in C++. For multisets, we can use the same functions we used for sets.
A multiset in C++ is used when you don't need a key/value pair but still need the search features of a set with several elements per key.
Here's an example demonstrating the same:
Output:
Functions on Multiset
Let's go through the various member functions in multiset in C++:
1. begin()
Returns an iterator to the first element in the multiset.
- Syntax: multiset.begin()
- Parameter: None
- Return: An iterator to the first element in the multiset.
2. end()
Returns an iterator to the theoretical element that follows the last element in the multiset.
- Syntax: multiset.end()
- Parameter: None
- Return: An iterator to the theoretical element that follows the last element in the multiset.
3. size()
Returns the number of elements in the multiset.
- Syntax: multiset.size()
- Parameter: None
- Return: The number of elements in the multiset.
4. insert(x)
Inserts a new element into the multiset.
- Syntax: multiset.insert(element)
- Parameter: The element to insert into the multiset.
- Return: None.
5. max_size()
Returns the maximum number of elements that the multiset can hold.
- Syntax: multiset.max_size()
- Parameter: None
- Return: The maximum number of elements that the multiset can hold.
6. empty()
Returns True if the multiset is empty, and False otherwise.
- Syntax: mmultiset.empty()
- Parameter: None
- Return: True if the multiset is empty, and False otherwise.
7. erase(x)
Remove all elements from the multiset that have the same value.
- Syntax: multiset.erase(x)
- Parameter: The element to remove from the multiset.
- Return: None.
8. clear()
Removes all elements from the multiset.
- Syntax: multiset.clear()
- Parameter: None.
- Return: None.
Implementation of Functions
Examples
1) Removing Elements from Multiset
Let's learn how to remove an element from a multiset in C++ with some value:
Output:
2) Swapping Multisets Using swap()
Output:
Let's try swapping two string multisets.
Output:
3) Finding the Size of a Multiset in C++ using size()
Output:
4) Removing all the Elements from the Multiset Container Using the clear() Function.
Output:
FAQs
Q: What is a multiset in C++?
A: A multiset is a container in C++ that allows you to store multiple occurrences of the same value in a sorted order. It is part of the C++ Standard Template Library (STL) and is implemented as a balanced binary search tree.
Q: How is a multiset different from a set in C++?
A: A set in C++ stores unique elements in sorted order, allowing each element to appear only once. In contrast, a multiset allows duplicate values and stores them in sorted order.
Q: How do I implement a multiset in C++?
A: There are two ways to implement a multiset in C++:
- Use the standard std::multiset class.
- Implement your own multiset class.
Q: When should I use a multiset?
A: Multisets can be used in a variety of applications, such as:
- Counting the number of occurrences of each element in a sequence
- Finding the median of a sequence
- Implementing priority queues
- Maintaining a leaderboard
Conclusion
- The C++ STL ( STL stands for Standard Template Library) includes multisets.
- Multisets are associative containers similar to Sets that store sorted values (the value is the key of type T).
- Unlike sets, multisets can have various redundant keys.
- Multisets compare the keys by default with the operator.
- The value of a multiset's items can be added or removed but not changed (The items are always const).
- The content of a multiset is a key, which is of type T, but unlike Sets, they can contain redundant keys.