Static Member in C++
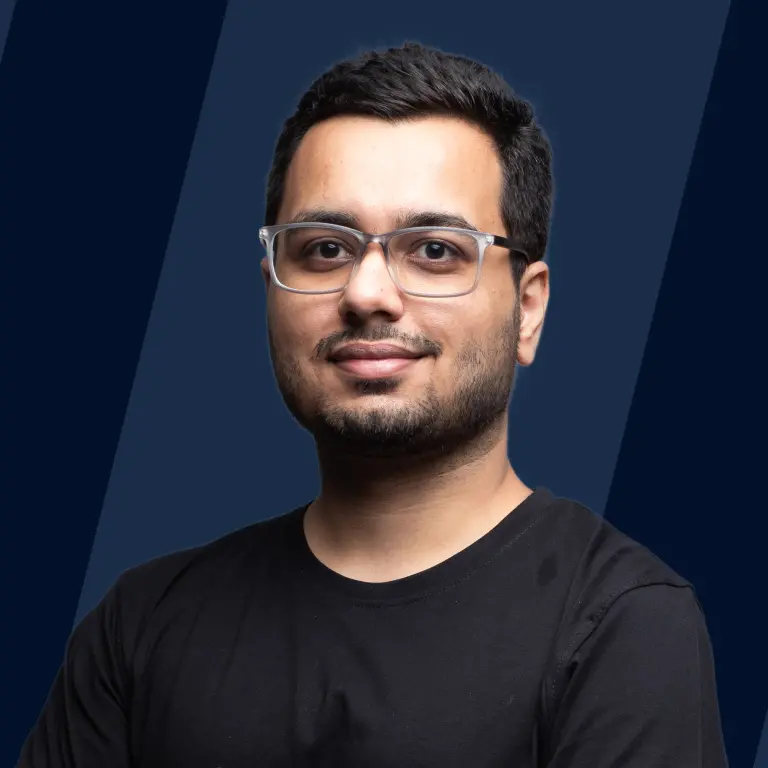
What is Static Data Member in C++?
A static data member in C++ is a class member that is shared by all objects of the class. There is only one copy of a static data member, even if multiple objects of the class are created. Static data members are initialized before any object of the class is created.
Syntax
The syntax for declaring a static data member is as follows:
where data_type is the type of the data member and data_member_name is the name of the data member.
Illustration
The following C++ program demonstrates the use of Static data member in c++:
Output:
Explanation
In the above program, the count data member is a static data member of the MyClass class. This means that there is only one copy of the count data member, even though two objects of the MyClass class are created. The count data member is initialized to 0 before any object of the MyClass class is created.
In the main() function, two objects of the MyClass class are created. The first object initializes the count data member to 1. The second object does not initialize the count data member, because it is already initialized by the first object.
Therefore, when the code prints the value of the count data member, it prints the value 2.
Accessing a Static Member in C++
To access a Static data member in C++, we can use either of the following methods:
1. Using a class object:
Output:
2. Using the scope resolution operator (::) Static data members can be accessed even without creating an object of the class. Here is an example of how to access a static data member without creating an object of the class using scope resolution operator (::)
Output:
How to Define a Static Data Member
Static data member in C++ are declared by using the static keyword inside the class, but as they have the lifetime until the program runs and is accessible to every class object they must be initialized outside the class. We initialize static data members after the class declaration and before the main function. The scope resolution operator is used to access them.
The memory block of static data members is shared by every object of the class. So, if there is any change made in the static data member, it will be updated for every other object of the class.
Static data member in C++ can be accessed anywhere in the program after the declaration of class either using the class instance or using scope resolution, class name, and variable name. There is no need to create objects to access them.
Let’s take an example to see the syntax and to get an idea about initialization:
Output :
In the above code, we have created a class which consists of a static data member var. Then, we have initialized var after the declaration of class and before the main function. Take a look over the syntax for initialization of static data members:
Syntax:
In the main function, we created two objects of class myClass. We made the changes in the value of the var by one object and showed that by another object.
Static Member Functions
As we have discussed about the static data members, similarly we have static member functions which are defined using the static keyword. Since, we have defined these functions as static, so they consists of class properties rather than object properties. We can access static member functions by using the class name and scope resolution operator.
Syntax :
As we can call static member function by just using class name and function name, they can be called even if no object of class be created.
A static member function can only access static data members, other static member functions, and any other functions from outside the class. Static member functions do not have access to this pointer because they have class scope.
Let’s see the example of static data members to get a better understanding of it:
Output :
In the above code, we have created a class that consists of static data and function members. Then we have initialized the static data member before the main function and in the main function using the static member function printed the value of var without even creating the object of our class myClass.
Constructor Calling
To initialize the member variables of a class instance or class object there are special member functions known as constructors. According to requirements (which member variable we want to initialize), we can define our own constructors in a class. Now, if we have a class that consists of a member object, to initialize the object of this class we will call the constructor that leads to multiple constructor calls. Initially, the constructors of all member objects are called after that program calls the constructor of the parent class which consists of all member objects. First, all member objects will be created and initialized then our complete object will be created and initialized. We will see this with an example in the next section.
Initialization of Member Objects
As we have discussed the constructors in the above section, we can conclude that the initialization of an object of any class totally depends upon the constructor.
Let’s take an example to get an idea about initialization.
We will create two classes:
- First Class: First class contains a member variable S with the data type string, a default constructor, a parameterized constructor, and a copy constructor.
- Second Class: This class contains three member variables, the first one is an integer variable, second and third both are member objects as they are objects of the first class. Further, there are two constructors, one is default and another one is parameterized. At last, there is an initializer list used to initialize all the class data members at the same time without assigning them values.
Output:
In the above example, we have taken three cases of initialization of a member object and object of a class that has a member object.
Case1(obj1) : By using default constructors, created and initialized both object and member object.
Case2 (obj2) : By using the default constructor for member object and parameterized constructor, we created and initialized object of the class.
Case3 (obj3) : Used initializer list to initialize both member objects and data object of our second class. In the above two cases, member objects first call their default constructor for initialization but in this case, the member object will directly get the value given in the initializer list and skip the default constructor call.
The above example provides three cases, but there could be many more, totally depending on the constructors defined by the user.
Has-A Relationship
There are two ways by which a class can use the properties of another class or we can say, there are two types of relations between classes.
- Is-A relation: When a class uses the properties of another class by means of inheritance, then the relation between them is Is-A relation.
- Has-A relation: When a class uses the properties of another class by having its object as a member variable, then the relation between them is Has-A relation.
The main motive of both the relations is to re-use the properties to avoid re-declaration.
Conclusion
- Member objects of a class are the class member variables that are objects of another class that is already defined.
- When a class has a member object, the constructors of the member objects are called before the constructor of the main class when creating an object. This ensures that the member objects are initialized first.
- If a class has an object of another class as a member variable that binds them in the Has-A relation.
- Static members are defined using the static keyword. For static members only once the memory is allocated.
- Static data member in C++ are declared inside the class but they are initialized outside of the class.
- Static member functions can be accessed using class name and scope resolution operator. Also, we can call the member function without creating any object of the class.