Copy Constructor in C++
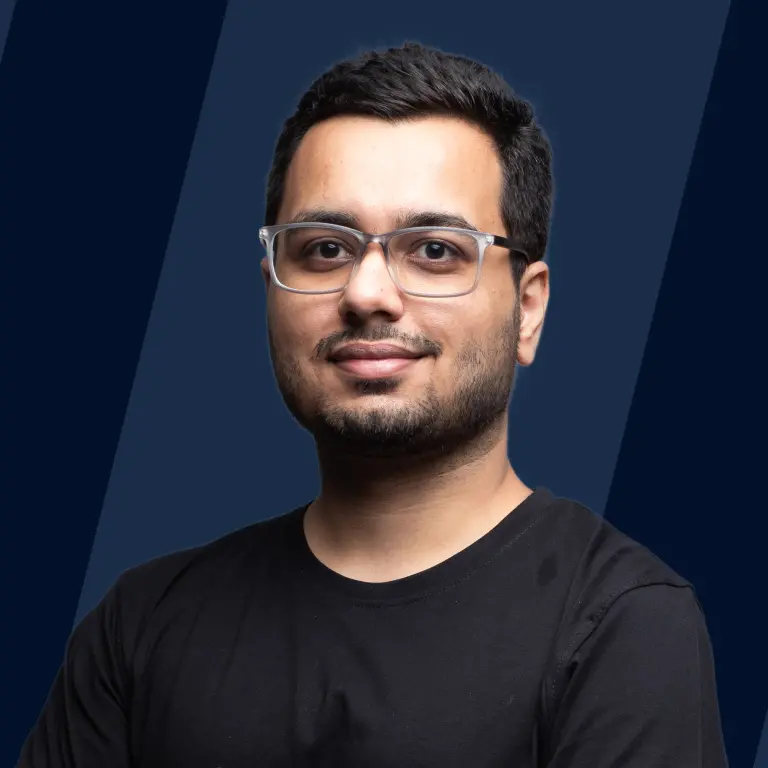
A constructor is used to initialize an object. A copy constructor in c++ is a member function of a class that initializes an object with an existing object of the same class. In other words, it creates a copy of an already existing object and stores it in a new object. Consider an object of class Car, WagonR_1. A copy constructor can create another object of this car, which will be the same as WagonR_1, say WagonR_2.
New Object, i.e., WagonR_2, is the exact copy of the existing object. It is possible to make changes in WagonR_2 further and make it entirely different based on what kind of copy it is. Keep reading to understand what that means.
Syntax of Copy Constructor in c++
Like any other constructor in C++, the Copy constructor does not have a return type, not even void. Instead, the member function for the Copy Constructor is created using the following syntax:
Example of Copy Constructor in c++
The following code describes an example of a simple Copy Constructor in C++:
To find a complete code sample, please go through the provided link to get a well-documented code sample of a sample class describing the workings of the Copy Constructor in C++.
Characteristics of Copy Constructors in C++
-
A copy constructor serves the purpose of initializing a new object's members by copying the members from an already existing object.
-
This constructor takes a reference to an object of the same class as its argument, facilitating efficient copying.
For example:
-
This process of member initialization using a copy constructor is known as copy initialization.
-
Copy constructors are often referred to as member-wise initializers because they initialize an object based on an existing object, copying each member individually.
-
While the compiler can automatically generate a copy constructor, programmers can define their own custom copy constructor for specific requirements. If not defined by the programmer, the compiler generates a default copy constructor.
Types of Copy Constructors in C++
Default Copy Constructor
C++ automatically provides a default copy constructor if you don't define one. This constructor performs a shallow copy of each non-static member of the class.
Uses:
- For classes without pointers or dynamic memory allocation, where shallow copying is sufficient.
- In classes that don't require custom copying logic, relying on the default copy constructor saves time and code.
Example:
Explanation: In this example, SimpleClass does not explicitly define a copy constructor, so the compiler provides one. This default copy constructor performs a shallow copy, which is sufficient here because SimpleClass does not contain any dynamically allocated memory or complex resources.
User-defined Shallow Copy Constructor
A user-defined shallow copy constructor explicitly defines how objects of a class are copied. It's useful for adding custom logic to the copying process while still performing a shallow copy.
Uses:
- When you want to monitor object copying.
- When shallow copying is sufficient but you need to execute additional operations during the copy (e.g., incrementing a static counter to track the number of copies).
Example:
Explanation: The Widget class defines a shallow copy constructor that copies the id field. It also includes a print statement to demonstrate when the copy constructor is invoked. This is a shallow copy because it only copies the value of id, which is a simple data type.
User-defined Deep Copy Constructor
A deep copy constructor is necessary when your class manages dynamic memory or resources. It ensures that each instance has its own copy of the resources, preventing issues like double-free errors.
Uses:
- For classes that manage resources such as dynamic memory, or other resources that require distinct copies to avoid resource sharing or double-free errors.
- In scenarios where each object copy must have its own copy of the resources to maintain proper control and avoid side effects.
Example:
Explanation: DeepClass manages a dynamically allocated char array. Its deep copy constructor allocates new memory for data in the copy and then copies the content, ensuring that obj1 and obj2 do not share the same data memory.
Copy Constructor with Copy-and-Swap Idiom
The copy-and-swap idiom provides a unified approach for copy construction and assignment operation, leveraging a swap function to manage resources safely and efficiently, especially in exception-prone situations.
Uses:
- Ensures that objects remain in a valid state even if an exception is thrown during the copy or assignment process.
- By unifying the logic for copy construction and assignment, code duplication is reduced, making the class easier to maintain.
Example:
Explanation: SwapClass implements a copy constructor that creates a deep copy of the data pointer. The swap function then allows for the efficient swapping of resources. This pattern is particularly useful when implementing exception-safe copy assignment operators.
Deleted Copy Constructor
Deleting the copy constructor makes a class non-copyable. This is useful for classes that should not be copied, such as singletons or classes managing unique system resources.
Uses:
- To ensure that a class can have only one instance.
- For classes that manage unique system resources where copying does not make sense or could lead to resource conflicts or leaks.
Example:
Explanation: NonCopyable explicitly deletes its copy constructor, making it impossible to create a copy of an NonCopyable object. Attempting to copy obj1 into obj2 would result in a compilation error, enforcing the non-copyable behavior.
When a copy constructor is called?
Let's consider a simple Car class as an example to illustrate the copy constructor.
Scenarios of Calling the Copy Constructor
-
Direct Initialization: When one object is initialized with another existing object of the same class.
Here, car2 is directly initialized using car1, which invokes the copy constructor.
-
Passing Object by Value to a Function: When a Car object is passed to a function by value, the copy constructor is called to create a copy of the object in the function's parameter.
-
Returning Object by Value from a Function: When a function returns a Car object by value, the copy constructor is called to create a return value from the function's local object.
In this scenario, the copy constructor may be called when car4 is returned by value. However, due to Return Value Optimization (RVO), modern compilers might optimize away this copy.
-
Creating a Copy Explicitly: When creating a new object as a copy of an existing object explicitly.
Here, car7 is explicitly created as a copy of car6, which directly invokes the copy constructor.
Copy Elision
Copy elision is an optimization technique used by C++ compilers to reduce the number of copy operations required to move objects around, particularly to and from functions. This optimization can significantly improve performance by minimizing unnecessary constructor and destructor calls, and it's especially beneficial in cases where objects are large or complex.
Example Consider a simple class and a function that returns an object of this class:
In this example, when createWidget is called, and w is returned, the compiler may apply RVO, directly constructing w in the memory space of myWidget, thus avoiding the need to call the copy constructor and then destroy the temporary object. Consequently, you may only see the output from the parameterized constructor, and no output from the copy constructor, indicating that copy elision has occurred.
Copy Constructor Vs Assignment Operator in C++
The comparison between Copy Constructor and Assignment Operator in C++ ,
Characteristic | Copy Constructor | Assignment Operator |
---|---|---|
Purpose | Creates a new object as a copy of another existing object. | Assigns a new value to an already initialized object from another existing object. |
Memory Allocation | Allocates new memory storage for the new object. | No new memory allocation; it operates on the already allocated memory of the object. |
Syntax | MyClass(const MyClass& other) | MyClass& operator=(const MyClass& other) |
Called with Initialization | Called when a new object is created from an existing object, often during object initialization. | Called when an existing object is assigned a new value. |
Example | MyClass t1; MyClass t2 = t1; | MyClass t3; t2 = t1; |
Example of a Class Where a Copy Constructor is Required
A user-defined copy constructor is needed when an object has pointers or any runtime allocation of resources like file handles or network connections. It's essential to ensure that the copy constructor creates deep copies, making pointers of copied objects point to new memory locations.
In the provided example, the String class requires a custom copy constructor to handle deep copies. Here's an explanation:
This custom copy constructor ensures that when a new String object is created as a copy of an existing object, it makes a deep copy of the string data, creating new memory storage for the copied object. This is necessary to prevent sharing the same memory with the original object.
The provided program demonstrates the use of the custom copy constructor by creating two String objects, str1 and str2, and making sure that changes to one object do not affect the other.
FAQs
Q. Can we make the copy constructor private?
A. Yes, making the copy constructor private can prevent direct copying of objects, which is useful in some design patterns, like the Singleton pattern.
Q. Why must the argument to a copy constructor be passed as a reference?
A. Passing by reference avoids making a duplicate copy of the object, ensuring efficient copying and memory savings.
Q. Why should the argument to a copy constructor be const?
A. Declaring the argument as const ensures that the original object remains unchanged during the copy operation, promoting safety and preventing accidental modifications.
Conclusion
- A copy constructor initializes an object with an existing object, creating an exact copy.
- It can be user-defined or automatically generated by the compiler.
- Copy constructors are called when creating objects based on existing ones, passing objects by value, or returning objects by value.
- A default copy constructor creates shallow copies, while user-defined copy constructors enable deep copies, preventing shared memory.
- User-defined copy constructors are required for objects with pointers or runtime resource allocation.
- Copy constructors use the syntax ClassName(const ClassName &object).
- Private copy constructors are used in design patterns like Singleton.
- Passing arguments by reference in copy constructors avoids duplicate copies.
- Declaring copy constructor arguments as const ensures the original object remains unchanged during copying.
With this, we hope you better understand Copy constructors in C++. Thank you for reading!
Happy Learning 🙂