Storage Classes in C++
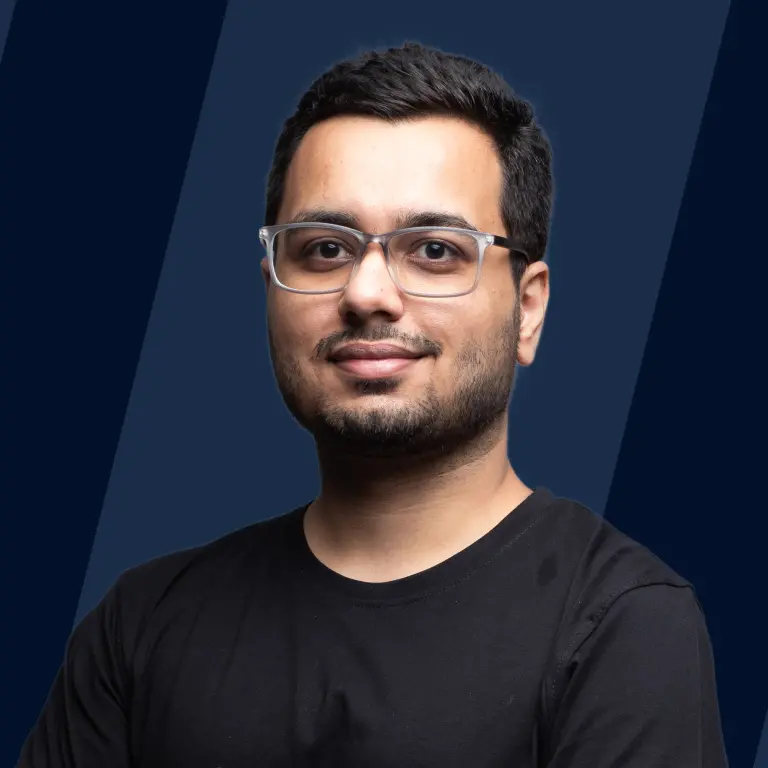
Storage classes are type specifier in C++ that helps specify the lifetime and visibility of variables and functions within a C++ program. C++ storage classes help determine the existence of a particular variable or function within a program's run time. They tell us which part of a program we can access a variable. Storage classes are especially significant because they determine how any variable may be used within a program or any method, especially in the context of their scope, lifetime, and storage.
Storage classes give us the following information about a variable or function:
- The scope or the part of the program up to which the function or variable can be used.
- The location where the function or variable is stored.
- The initial value of variables.
- The lifetime of a variable.
- Accessibility of a variable.
There are several storage classes in C++. They are as follows:
- automatic
- static
- register
- external
- mutable
- thread_local
Each of these storage classes implies different visibilities, lifetimes, and scopes of functions or variables that are declared with. The visibility or scope of a variable can be broadly divided into two categories:
- Local
- Global
Syntax:
The following syntax is used to define the storage class for a variable:
Where, storage_class specifies the storage class. var_data_type specifies the variable’s data type. var_name specifies the variable name.
The following keywords are used to specify the various storage classes:
Storage_Class | Keyword |
---|---|
Automatic | auto |
Static | static |
Register | register |
External | extern |
Mutable | mutable |
Thread Local | thread_local |
Types of Storage Classes in C++
In the following section, we look at the types of storage classes in C++.
Storage Class | Keyword | Lifetime | Visibility | Initial Value |
---|---|---|---|---|
Automatic | auto | Function Block | Local | Garbage |
External | extern | Whole Program | Global | Zero |
Static | static | Whole Program | Local | Zero |
Register | register | Function Block | Local | Garbage |
Mutable | mutable | Class | Local | Garbage |
Thread-Local | thread_local | Thread | Local |
1. Automatic
Description:
The automatic storage class in C++ is the default storage class for all local variables. The auto keyword is used to declare the automatic storage class for variables. The automatic storage class in C++ can also be used for the automatic deduction of data type and, as such, can be used while declaring a variable without specifying the data type.
Following C++11 standards, the auto keyword is now used for the automatic deduction of datatype in C++. The compiler automatically infers the data type of a variable declared auto based on the data assigned to it.
Properties:
Automatic storage class in C++ is the default storage class for variables declared without a specific storage class and has a local scope and lifetime.
The lifetime of an automatic variable is the function block within which the variable is declared, and the visibility of the variable is also local. Its initial value is a garbage value.
Auto variables are declared without specifying the storage class for the variable as:
Where, var_data_type specifies the variable’s data type. var_name specifies the variable name.
Example:
Output:
In the code snippet above, we have declared x inside the test() function as a local variable, and it has been automatically set to the auto storage class. The variable y has been declared with the auto keyword, which automatically infers its type using the assigned value of the variable. Within the main() function, we have declared two more variables, x and y, similar to the other two. Due to the scopes of the variables being local, calling the test function within main() does not change the values of x and y in main().
2. Static
Description:
The static storage class in C++ is used to declare a variable that, once initialized, is not deallocated when the variable goes out of scope. The lifetime of such a variable is the entire program; as such, the variables do not lose their values within function calls. It is beneficial in recursive calls as static variables are initialized only once within a function block and can be changed on each recursive call without getting initialized over and over. Global variables may also be declared static, but such variables cannot be used outside the file in which it is declared.
Properties:
Static storage class in C++ defines variables whose lifetime is equal to the program's lifetime, even if their scope is local.
The lifetime of a static variable is the entire program, but a static variable's visibility is local. Its initial value is zero.
Static variables are declared with the following syntax:
Where, var_data_type specifies the variable’s data type. var_name specifies the variable name.
Example:
Output:
In the code snippet above, we have declared a static variable, a, within the test() function. Within the main() function, we have again declared a static variable, a. When the test() function is called within main() twice, the local variable within test() is initialized only once, and it retains its value within function calls. The a variable declared within main() is not changed with calls to test() as it has a local scope.
3. Register
Description:
The register storage class in C++ utilizes CPU registers to store data to access data quickly in a function. The functionality of register variables is similar to automatic variables. The only difference is that the compiler tries to store the variable in the register instead of the memory if the registers are free. The compiler stores the register variables in the memory if no registers are free.
It is important to note that the address of a register variable cannot be accessed as they are stored in registers and not the memory. Register storage class in C++ is used to declare variables stored in registers by the compiler for faster access.
Properties:
Register variables are used when a program requires frequently accessing a variable. Registers, being a part of the processor, allow quick access to memory, much faster than standard memory. Register variables have local visibility, and their lifetime is the function block within which they are declared. Register variables are initialized with a garbage value.
Syntax: Register variables are declared with the following syntax:
Where, var_data_type specifies the variable’s data type. var_name specifies the variable name.
Example:
Output:
In the code snippet above, we have declared a register variable in both test() and main(), initialized separately as the register has a local scope.
4. External
Description:
The external storage class in C++ is used when a variable or function is declared on a separate function block from the definition. That is, the variable or function may be defined in one code block or even file and then be declared and used in another code block or file. Using the extern keyword tells the compiler that the variable or function is defined elsewhere. It specifies external linkage for a variable. In the case of variable declarations without definition using the external storage class, no memory is allocated to the variable until it is defined elsewhere.
Properties:
The external storage class in C++ is used to declare variables defined elsewhere and have their lifetime and visibility as global.
The external storage class is specified using the extern keyword. Its lifetime is the whole program, and its visibility is global, i.e., it is accessible by any block within the program. It is initialized with a value of 0. The external storage class is usually used when a variable or function is declared in separate files within a large program.
Syntax: External variables are declared with the following syntax:
Where, var_data_type specifies the variable’s data type. var_name specifies the variable name.
Example:
Output
In the code snippet above, we have declared the variable x as a global variable. The extern keyword within test() specifies that the variable x is declared somewhere else outside the current code block and, as such, allows us to access the global variable.
5. Thread_local
Description:
The Thread-local storage class in C++ defines variables local to each thread created in the program. In the case of a program with multiple threads, each thread receives its instance of variables declared as thread-local. Thread_local variables may seem like static or global variables, but their lifetime is only the duration of a particular thread. That is, thread-local variables are created on thread creation and are disposed of when the thread is exited.
Properties:
Thread Local storage class in C++ is used to declare variables local in scope and lifetime to the thread in which they are declared.
The Thread local storage class is specified using the thread_local keyword. Its lifetime is the same as the lifetime of a thread, and its visibility is local.
Syntax: Thread local variables are declared with the following syntax:
Where, var_data_type specifies the variable’s data type. var_name specifies the variable name.
Example:
Output
In the code snippet above, we have declared a thread-local variable with an initial value of zero. We have changed the thread-local val value inside the test variable and printed it and its address. Inside the main, we have changed the value of val and created a thread to run the test() function. We have also printed the variable and its address before and after the thread. We observe that a separate instance of the val is created in each thread with different initialization and address.
6. Mutable
Description:
The mutable storage class in C++ is used where any particular data member of a struct or a class must be modified in an otherwise const object. Any constant object of a class or structure will always have constant data members and functions. But using the mutable storage class allows us to change those variables and use them as non-constant. It is used when one wishes to change only a few data members in a class or structure without changing the value of the others.
Properties:
Mutable storage class in C++ is used to declare variables to be changed in an otherwise constant object or class.
The mutable storage class has a lifetime equal to that of the class, and it has local visibility. The initial value of a mutable storage class is a garbage value.
Syntax: Mutable variables are declared with the following syntax:
Where, var_data_type specifies the variable’s data type. var_name specifies the variable name.
Example:
Output
In the code snippet above, we have declared a class Test with several data members and functions. Within main(), we have declared constant objects of class Test. Thus all data members of Test are also constant. However, we have declared x as mutable; hence, it can be modified.
Conclusion
- C++ storage classes help define the lifetime and visibility of variables and functions within a C++ program.
- The scope of C++ variables or functions can be either local or global.
- There are several C++ storage classes, namely Automatic, Register, Static, External, thread_local, and Mutable.
- Storage Duration in C++ refers to the minimum time a variable exists within a program and might be usable or accessible.
- Linkage in C++ is the property that determines if a variable declared separately in multiple scopes refers to the same instance of the variable or separate.