Nested Classes in C++
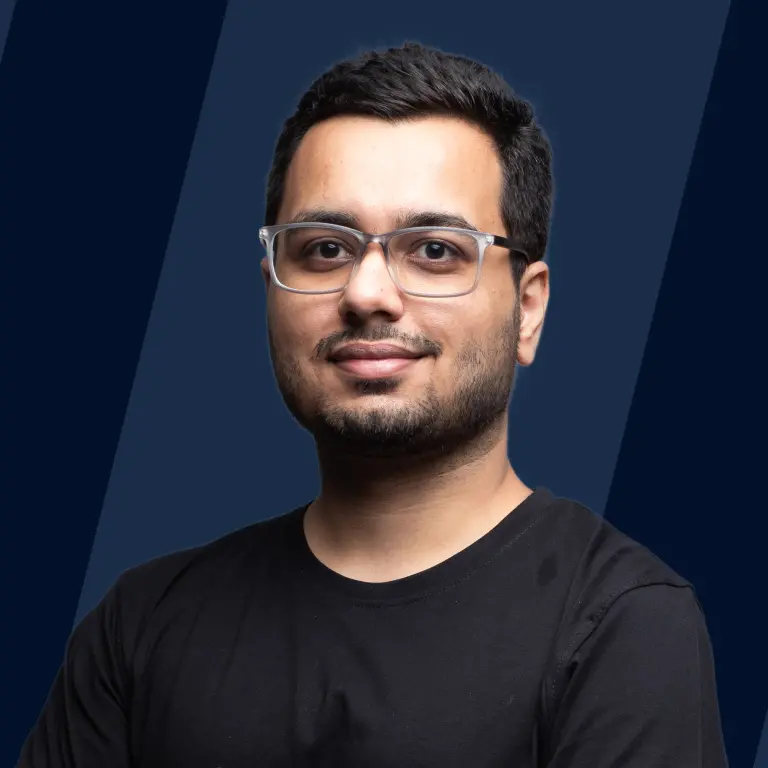
Nested class in C++ is a type of class that is declared inside another class. The class inside which a nested class is declared is called an enclosing class.
The data members declared inside the enclosing class do not have any special access to the nested class data members. Similarly, the members declared inside the nested class have usual-usage access to the members of the enclosing class. The nested class in C++ will be considered a member of the enclosed class.
Note: Nested class in C++ can be accessed outside the enclosing class using scope resolution operator(::).
Syntax
Examples
Let's take a look at some of the examples to understand the concept of nested classes more clearly.
Example 1
In the program, we will discuss how a member class can access class members of the enclosing class.
Code
Example Explanation: In the above program, we have defined a nested class inside the enclosing class. Then we have defined a member function inside a nested class that accesses the data member of the enclosing class without any error as the private data members of the enclosed class can be accessed by the nested class.
Example 2
In the program, we will discuss how an enclosing class cannot access the private members of the nested class.
Code:
Output:
Example Explanation: Here, we have again defined a nested class inside the enclosing class. Inside the enclosing class, we defined a member function that is accessing the data member of the nested class which will throw an error as the data member inside the nested class is private.
Member Functions in Nested Classes
Member functions that are declared inside the nested function can be accessed outside the class using the scope resolution operator(::).
Let's see an example to understand the concept of a member function in a nested class:
Code:
Example Explanation:
In the above program, we have declared an enclosing class, inside which a nested class is also declared. After that member functions are declared inside the nested class but they are not defined. The member function is then defined outside the class scope using the scope resolution operator(::).
Friend Functions in Nested Classes
A friend function is a type of non-member function in C++ that has some privileged access to access private and protected members of a class. It is declared as a friend of the class using the keyword friend.
We can use the friend function for nested class also which does not have any special access to the member of the enclosing class.
Let's take an example to understand the concept more clearly:
Code:
Scope Visibility of Nested Class Declaration
Nested classes in C++ are designed to be declared within the scope of the enclosing class and are available for use in the entire scope.
A fully qualified name must be used for the usage of a nested class in a different scope other than the scope of the immediately enclosing class.
If a type name is declared with a forward declaration then there can be an exception in the scope visibility of the nested class. In this exception, the forward declaration of the class name is visible outside the enclosing class, with its scope defined as the smallest enclosing non-class scope.
How to Define Nested Class as Private Member of Enclosing Class?
Inside an enclosing class, the nested class can be declared as the private member of that enclosing class. Therefore, the member functions of the nested class can be accessed by the objects of the enclosed class.
Let's take an example to understand the concept more clearly:
Code:
Output:
Example Explanation:
In the above program, we have declared an enclosed class and a nested class. The object of the enclosing class i.e., enc is accessing the member function print which is defined inside the nested class, and printing the required message as seen in the output.
How to Define Nested Class as Public Member of Enclosing Class?
Inside an enclosing class, a nested class can be declared as a public member of that enclosing class. The object of the enclosing class can access the public member functions of the nested class directly.
Let's take an example to demonstrate how a nested class can be defined as a public member.
Code:
Output:
Explanation:
In the above program, we have defined an enclosed class and a nested class. The object of the enclosing class i.e., en is directly accessing the public member function of the nested class i.e., print with the help of the scope resolution operator(::), and printing the required message as seen in the output.
How to Define Member Functions of Nested Class Outside the Enclosing Class?
The member function of a nested class can be initialized outside the scope of the enclosing class with the help of the scope resolution operator(::).
Let's take an example to understand the concept of defining nested classes outside the scope of enclosing classes.
Code:
Output:
Explanation: Here, we have defined the member function of the nested class outside the scope of an enclosed class. The scope resolution operator is used to define the member function and then to call the member function from the main method.
How to Define Nested Class Outside the Enclosing Class?
We can define the whole nested class outside the enclosing class with the help of the scope resolution operator.
Let's take an example to demonstrate the concept:
Code:
Output:
Explanation:
In the above program, we have defined the whole nested class outside the enclosing class. Here, again scope resolution operator is used to define the class.
Learn More
-
Storage Class:- Storage class in C++ is used in specifying the visibility and lifetime of the functions and variables within a C++ program. To learn more about Storage classes in C++, visit Storage Classes in C++.
-
Friend Function:- A friend function is a type of non-member function in C++ that has some privileged access to access the private and protected members of a class.
Learn more about Friend Function in C++ with Scaler Topics.
Conclusion
- When a class is declared inside another class then it is known as Nested class in C++. The class inside which a nested class is declared is called an enclosing class.
- Nested class in C++ can be declared as both a private and public member of an enclosing class.
- If a nested class in C++ is defined as a public member of the enclosing class then scope resolution operator(::) is used to create an object of nested class inside the main method.
- The data members declared inside the enclosing class do not have any special access to the nested class data members.
- Nested classes in C++ are designed to be declared within the scope of the enclosing class and are available for use in the entire scope.