Templates in C++
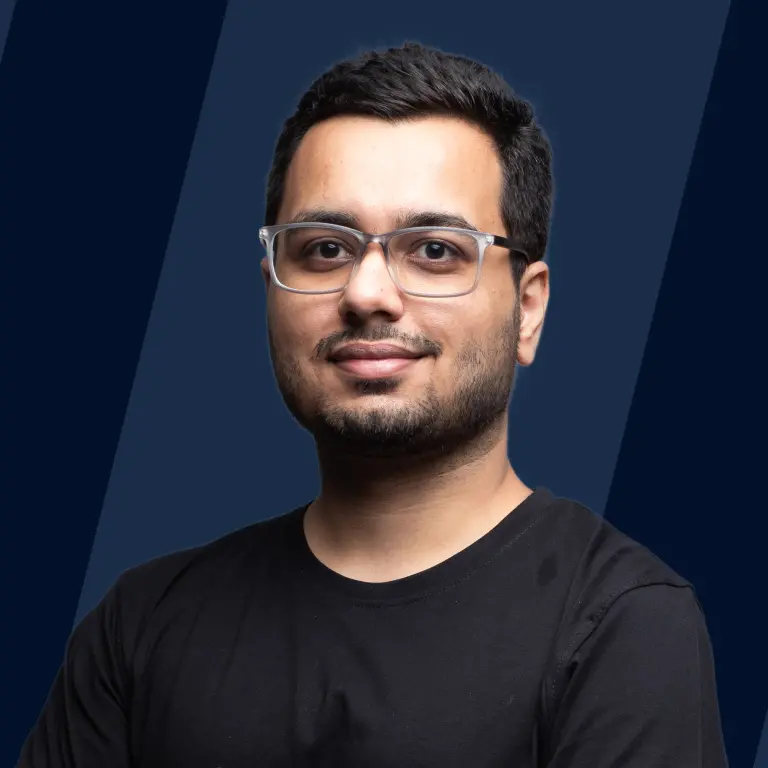
The templates are one of the most powerful and widely used methods added to C++, allowing us to write generic programs. It allow us to define generic functions and classes. It promote generic programming, meaning the programmer does not need to write the same function or method for different parameters.
We can define a template as a blueprint for creating generic classes and functions. The idea behind the templates in C++ is straightforward. We pass the data type as a parameter, so we don’t need to write the same code for different data types. Refer to the image below for better visualization.
We use two keywords - template and typename in templates in C++ to achieve generic programming.
Note: The typename keyword can always be replaced by the keyword class.
Some of the most common examples of templates in C++ can be:
- Library containers like iterators. We can define iterators of different data types by passing the data type as a parameter to the iterator.
- Sorting algorithms defined for STL in C++. We can have the data sorted in an order irrespective of the data type.
Examples of templates in C++:
- vector <int> vec;
- vector <char> vec;
- stack <string> s;
- queue <int> q; etc.
How Templates Work?
We use templates in C++ to create generic methods and classes. It get expanded at compile time, just like any macros (example #define PI 3.14), and allow a function or class to work on different data types without being rewritten.
Refer to the image below to see the compile-time working of templates in C++.
To use templates in C++, we need to use the two keywords - template and typename. We should first write the keyword template which tells the compiler that the current function or class is a blueprint or template. After writing the template, we mention the keyword typename and a placeholder name (T) for a data type used by the function or class.
Templates in C++ can be implemented in two ways, i.e., Function Templates and Class Templates. Refer to the next section for a detailed explanation and implementation.
Types of Templates in C++
As we know, we can use templates in C++ to define generic functions and classes. We can represent templates in C++ in two different ways, namely - function templates and class templates. Let us learn about both representations in detail.
1. Class Templates
Just like the function templates in C++, we can also use class templates to create a single class that can work with the various data types. Just like function templates, class templates in C++ can make our code shorter and more manageable.
Syntax of the template function:
In the syntax above for the class template:
- T is a placeholder template argument for the data type. T or type of argument will be specified when a class is instantiated.
- class is a keyword used to specify a generic type in a template declaration.
Note: When a class uses the concept of template in C++, then the class is known as a generic class.
Some pre-defined examples of class templates in C++ are LinkedList, Stack, Queue, Array, etc. Let’s take an example to understand the working and syntax of class templates in C++.
Example:
Output:
In the example above, we have defined a template class (Test) that returns the number of various data types. We have a return type T, meaning they can be of any type.
2. Functional Templates
Function templates are similar to normal functions. Normal functions work with only one data type, but a function template code can work on multiple data types. Hence, we can define function templates in C++ as a single generic function that can work with multiple data types.
Note: We can also overload a standard function to work on various data types.
Functional templates are more powerful than overloading a normal function as we need to write only one program, which can work on all data types.
Syntax of the template function:
In the syntax above:
- T is the type of argument or placeholder that can accept various data types.
- class is a keyword used to specify a generic type in a template declaration. As we have seen earlier, we can always write typename in the place of class.
Some of the pre-defined examples of function templates in C++ are sort(), max(), min(), etc. Let’s take an example to understand the working and syntax of function templates in C++.
Example:
Output:
In the example above, we have defined a template function, namely add(). We can provide multiple data types as arguments for the function.
Template Arguement Deduction
Template argument deduction automatically deduces the data type of the argument passed to the class or function templates. This allows us to instantiate the template without explicitly specifying the data type.
For example, consider the below function template to multiply two numbers:
In general, when we want to use the multiply() function for integers, we have to call it like this:
multiply<int> (54, 4);
But we can also call it:
multiply(46, 10);
We don’t explicitly specify the type ie 1,3 are integers.
The same is true for the template classes(since C++17 only). Suppose we define the template class as:
If we want to create an instance of this class, we can use any of the following syntax:
Note: It is important to note that the template argument deduction for a classes is only available since C++17, so if we
Example of Template Argument Deduction
The below example demonstrates how the STL vector class template deduces the data type without being explicitly specified.
Output
Note: The above program will fail compilation in C++14 and below compiler since class template arguments deduction was added in C++17.
Function Template Arguments Deduction
Function template argument deduction has been part of C++ since the C++98 standard. We can skip declaring the type of arguments we want to pass to the function template and the compiler will automatically deduce the type using the arguments we passed in the function call.
Example: In the following example, we demonstrate how functions in C++ automatically deduce their type by themselves.
Note: For the function templates which is having the same type for the arguments like template
Class Template Arguments Deduction (C++17 Onwards)
The class template argument deduction was added in C++17 and has since been part of the language. It allows us to create the class template instances without explicitly definition the types just like function templates.
Example: In the following example, we demonstrate how the compiler automatically class templates in C++.
Output
Overloading of Template Function in C++
Overloading is the feature that allows the specification of more than one function of the same name in the same scope.
So, by overloading template functions in C++, we can define function templates in C++ having the same name but called with different arguments.
Let us take an example to understand the overloading of the Template Function in C++.
Output:
In the example above, we have defined a template function named display(), which takes one argument and performs the instruction written inside it. We have also overridden the display() function with an integer argument. So, when we provide an integer value as a parameter to the function, an overloaded function (i.e., display(int t)) will be called rather than the template function. The template display method will be called for the rest of the data types, i.e., display(T t1).
Difference between Function Overloading and Templates in C++
Before learning about the difference between function overloading and templates in C++, we should first know what polymorphism is in C++.
Polymorphism means having many forms. Polymorphism is an important concept of Object-Oriented Programming. We can define polymorphism as the ability of a function or message to be displayed in more than one form.
Both function overloading and templates in C++ are examples of polymorphism in C++. We should use functional overloading when we need to define multiple functions performing similar operations. On the other hand, we should use templates in C++ when we need to define multiple functions performing identical operations.
One of the most important differences between function overloading and templates in C++ is that templates cannot take a varying number of arguments, but an overloaded function can take a varying number of arguments.
typename VS class keyword
The typename and class are keywords used in templates in C++. There is no difference between the typename and class keywords. Both of the keywords are interchangeably used by the C++ developers as per their preference. There is no semantic difference between class and typename in a type-parameter-key.
There is a special scenario or case where we cannot use typename at the place of class. When declaring a template of a template parameter, we must use class. Refer to the syntax below for a better understanding.
Invalid Usage:
Valid Usage:
Conclusion
- The templates are one of the most powerful and widely used methods added to C++, which allows us to write generic programs. It will enable us to define generic functions and classes.
- To use templates in C++, we use the two keywords - template and typename. We can also use the class keyword instead of typename.
- It removes code duplication and helps us to make generic callbacks.
- It helps us to write very efficient and powerful libraries. Example: STL in C++.
- It gets expanded at compiler time, just like any macros.
- Function templates are similar to normal functions. Function templates in C++ are single generic functions that can work with multiple data types.
- Just like the function templates in C++, we can also use class templates to create a single class that can work with the various data types.
- As templates are calculated at compile time rather than run time when template functions or classes are large and complicated, they can slow the compile time.