Auto in C++
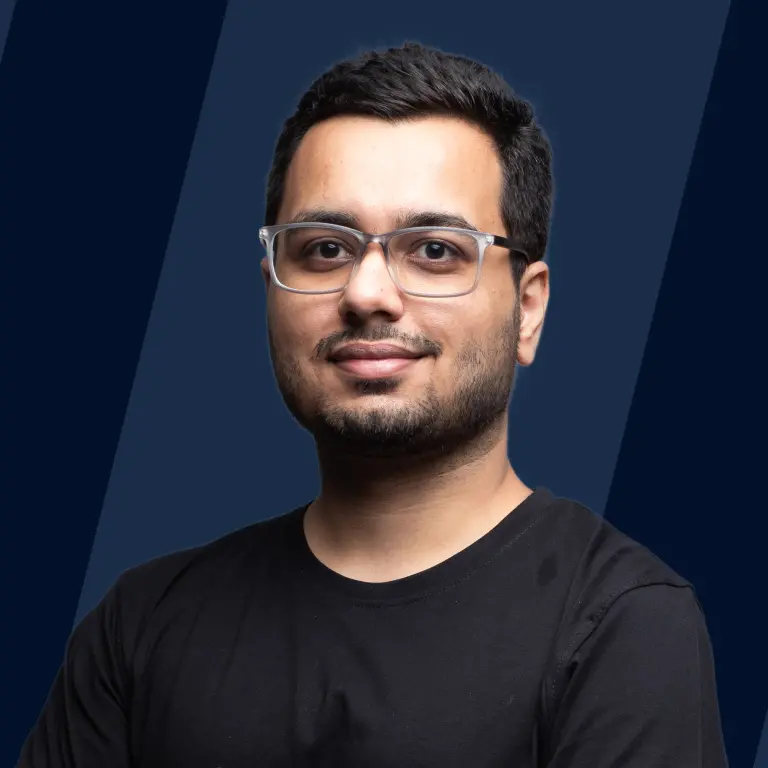
Overview
The auto keyword in C++ automatically detects and assigns a data type to the variable with which it is used. The compiler analyses the variable's data type by looking at its initialization. It is necessary to initialize the variable when declaring it using the auto keyword. The auto keyword has many uses, including but not limited to variables, functions, pointers, iterators, templates, and many more.
What is the Auto Keyword in C++?
Have you ever tried to declare an iterator for a map in C++? The exact syntax for the same will be
The above syntax looks tiny enough, but imagine you have a map of pairs of vectors. Also, consider the case where you must declare more than one iterator. Writing this piece of extended code, again and again, can be cumbersome. But there is a solution: the auto keyword in C++.
The auto keyword automatically detects the data type of a variable by its initialization. Not only this, but the auto keyword can also be used to declare the return type of a function. Let us look at this keyword in detail.
How Does The Auto Keyword Work?
Consider the C++ line auto x = 5;. The auto keyword deduces the type of x from the type of 5, the same way template type deduction works for function templates. For example, consider a function template:
At pos A, a type has been assigned to T based on the value passed for the parameter to func. When you do auto x = 5;, the same type deduction is used to determine the type of x from the type of 5 that's used to initialize it.
As explained above, the auto keyword in C++ detects the data type of a variable by itself. This means that we can replace the data type of a variable with the keyword auto in C++. The online C++ compiler will automatically detect the variable's data type at compile time. Also, the auto keyword is not only limited to variables. We can also use it as the return type for a function.
Let us look at both of these.
Auto Used with Variables
The auto keyword in C++ will analyze the variable's data type when used with variables. The compiler decides the data type for a variable by looking at its initialization.
Let us look at the syntax for the same:
Here var is the name of the variable. So at compile time, the compiler will reach the auto keyword and automatically assign the data type integer to var as it has been initialized with an integer value.
Note: When using the auto keyword with variables, the variable should be declared and initialized together. If the variable is not initialized with a value, the code will give a compile-time error as it will not be able to analyze the variable's data type because there is no value assigned to it.
Example: Program to use auto with variables in C++
Output
In this code, the compiler itself decides by looking at the initialization that the variable weight is a float type and age is an integer because of the auto keyword.
Auto Used with Literals
Literals denote a fixed value, usually used for initialization. When using the auto keyword with literals in C++, we can further aid the compiler in analyzing the data type using a suffix.
Let us look at an example to understand this better.
Example: Program to use auto with literals in C++
Here we have used suffix and the auto keyword to help the compiler determine the variable's data type. The variable d will be double by default. Still, the variable f will be given the type float, the variable u will be given the type unsigned long, and the variable st will be given the data type string because of the respective suffixes used.
Auto Used with Const Variables
The only problem with using the auto keyword is that constant variables will not be analyzed. If we want a variable to be constant and define it using the auto keyword, we will also have to use the keyword const along with the auto keyword. The syntax will be:
Here, var is the variable name and val is the value assigned to it, which will remain constant throughout the program because we have declared it a constant.
Auto Used with Functions
The auto keyword is also used as the return type for any function. The only condition is that each return statement inside this function should return the same variable type. The compiler decides the function's return type by analyzing the return statement expression. Let us look at the syntax for the same:
Here func is the function name, par1 and par2 are parameters of the function, and val is the returned value.
Note: The auto keyword is used with only those functions declared and defined in the same block. The reason is the same as that for variables. The compiler would not be able to decide the data type of the return variable if there is no function definition.*
Example: Program to Use Auto with Functions in C++
Output
In this code, the compiler decides the return type of the function to be an integer because of the auto keyword.
Function with the auto keyword in header file
The functions defined using return type as auto are also used in header files. But for that, its return type needs to be specified in the function declaration using the '->' operator.
Let us look at an example.
Output
In this example, the function product() has been declared using the auto keyword, and we have also specified the function's return type using the '->' operator. Now, this function can be included in a header file also. If we don't declare the expected return type using the '->' operator, then the function declared using the auto keyword cannot be included in a header file.
Difference Between auto and decltype in C++
Auto | Decltype |
---|---|
The auto keyword deduces the variable type by analyzing its initialization | The decltype keyword returns the type of the expression passed to it. This return value is used to declare the data type of some other variable. |
The auto keyword lets you declare a variable with a particular type | The decltype extracts and assigns the type of data from the variable, so decltype is like an operator that finds/evaluates the type of expression passed to it |
Difference Between Syntax of auto and decltype
So essentially, while the auto keyword deduces the variable type by its initialization, the decltype keyword deduces and assigns the data type of a variable based on the parameter passed to it.
Let us look at an example to see the same.
Output
Important Uses of the Auto Keyword in C++
We have already looked at the usage of the auto keyword with variables and as a return type of function. But there are many other instances where the auto keyword can be useful.
Let us look at some of these.
Iterators
We can use the auto keyword to declare iterators instead of writing out the complete syntax, which may be lengthy and time-consuming for some complex structures.
Example: Declare an iterator for a map
As the code above shows, using the auto keyword saves time and effort.
Function Parameter
The auto keyword can not only be used as the return type for functions but also as the data type of the parameters declared in the function.
When the function is called, the compiler analyses the correct data types of the parameters.
Example: Declare a function using auto for parameters
Output
Class Objects
The auto keyword can also declare custom class objects in C++.
Example: Declare a Custom Class Object
Output
Pointers
We can also use auto to declare pointers to other variables. Although we can use * along with the auto keyword to make the code readable, it is not necessary to analyze that it has been declared as a variable.
Example: Declare a Pointer
Output
References
Like pointers, we can also use auto to declare references to other variables. But for this, we will have to use the & symbol with the auto keyword.
The syntax will be:
Here, ref_var is the variable that references the variable var1.
Example: Declare Reference to a Variable
Output
Lambda Functions
The auto keyword can not only be used for the return type of normal functions but is also the best for lambda functions.
For a recap, lambda functions are functions that can be defined as a code snippet inside another function call so that they can use the variables that have been declared in the function before them without having to pass them as parameters.
Example: Declare Lambda Function
Output
Templates
Templates in C++ are like a generalized function that can be used for many different data types because we do not define them for any particular data type but instead for a generalized data type using a template.
Now, we can use the auto keyword for the return type of templates just like we can with functions. Let us look at an example.
Example: Declare template using auto keyword
Output
Structured Binding
Structured Binding in C++ helps us decompose the different components of a pair/tuple or an object consisting of different variables. So there will be a single object enclosing various other variables, which can be taken apart using structured binding.
The auto keyword is used in structured bindings to decompose the individual components.
Let us take the example of a pair and understand how we can use the auto keyword to specify the different variables enclosed.
Example: Structured Binding using auto keyword
Output
It is evident in this code how easy it became to assign num1 and d1 the values in the pair by using the auto keyword. We didn't even need to declare the variables. They were declared using the auto keyword.
What is auto&& in C++?
Like the auto keyword in C++, the auto&& keyword can be used as a universal reference value, which means it will accept any value, whether an lvalue or an rvalue reference expression. Let us look in detail at what this exactly means.
To recap, an lvalue expression is one whose resources cant be reused, like normal variables in C++ or any other objects that are declared using a name directly. For example, in the code int y = 7; is an lvalue. Similarly, is also an lvalue where is a vector. These objects are such that they are declared with a name and are permanent.
But an rvalue expression denotes an object whose resources cannot be reused, which means a temporary disposable object. These objects are not declared or defined explicitly. For example, in the code func(class_obj()) where func() is a function that takes a class object as a parameter. It is clear in the code that we have not explicitly defined any class object. Instead, we have just passed the function class_obj() as a parameter which will create a temporary class object and pass it to the function func(). Hence, this class object is temporary, disposable, and an rvalue.
Now, to reference an lvalue object or variable, we have to use one & symbol, but to reference an rvalue object or variable, we have to use two ampersands &&. But, if we use the auto keyword to declare the reference, we can use auto&&, which will work for both lvalue and rvalue reference expressions.
Common Mistakes with the Auto Keyword in C++
Although the auto keyword in C++ is beneficial, it may cause errors because of some very common mistakes while using it in your program. Some of these are:
Variable Initialization
As mentioned in the first section, it is necessary to initialize a variable in the same statement while declaring it with the auto keyword in C++. This is because the compiler will need the initialized value to assign the variable a data type, and not doing so will lead to a compilation error.
Multiple Variable Declarations using Auto
We usually declare multiple variables in the same line when they all are of the same data type.
For example, this syntax is very common in C++:
In this case, x and y are assigned the data type integer. But, if we try to do the same with the auto keyword, it will lead to an error. Only one variable declaration and initialization is permitted with the auto keyword in C++ in a line.
Integer and Boolean Values
You might've come across programs that use integers as boolean. Booleans are also sometimes initialized using integers. But, if you use the data type auto to initialize your variable, it isn't easy to understand whether the variable was intended to be a boolean value or an integer value. In this case, the auto keyword can be avoided to avoid confusion.
Auto Used to Store Function Return Values
Consider this line of code:
Here f() is some custom defined function. Looking at this code, we cannot know what data type var will be assigned. Instead, we will have to go and look at the definition of the function f() for the same, which might be cumbersome.
Transform your passion for coding into C++ expertise. Join Scaler Topics C++ Free course and become a proficient C++ developer.
Conclusion
- The auto keyword can be used in the place of the variable's data type, and the compiler will automatically detect and assign a data type to the variable.
- The auto keyword is used in two ways:
- With Variables: to detect data type.
- With Functions: to detect return type
- The difference between the auto keyword and decltype keyword is that the auto keyword deduces the variable type by its initialization. In contrast, the decltype keyword deduces and assigns the data type of a variable based on the parameter passed to it.
- The auto&& keyword can be used as a universal reference value to bind to both an lvalue or an rvalue expression.
- Other uses of the auto keyword are iterators, class objects, function parameters, lambda functions, structured binding, references, and many more.