Create Directory in Python
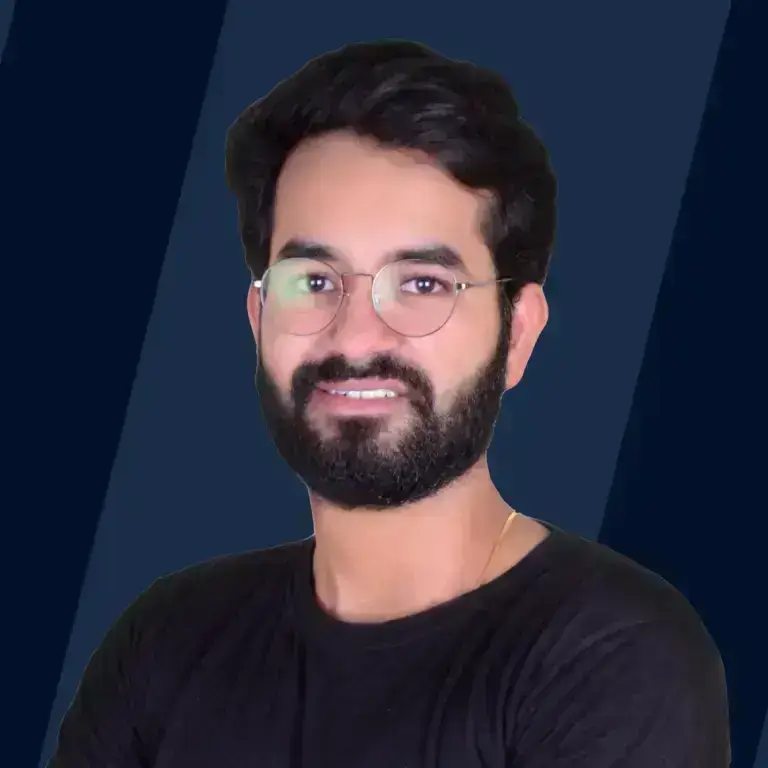
Overview
Python has an OS module in its standard utility modules that provides several functionalities to interact with the underlying operating systems and the files. If an inaccessible or invalid path, file name, or other arguments are passed to methods in OS module, OSError is raised by the program. OS module provides two methods os.mkdir() and os.makedirs() that are used to create directories using Python in the file system.
Introduction
Python has an OS module that provides several functionalities to interact with the underlying operating systems and the files. OS module comes under Python's standard utility modules, and you do not need to install or download this module separately from your Python installation. But it is not a built-in module so we have to import it before use. OS module provides the programmer with a portable way of accessing and using operating system dependent functionality. Several functions to interact with the computer's file system is provided in the os and os.path modules. If an inaccessible or invalid path, file name, or other arguments are passed to methods in OS module, OSError is raised by the program even if they have correct types but are not accepted by the underlying operating system as a valid argument.
OS module provides two different methods for creating directories in Python, that are -
- os.mkdir()
- os.makedirs()
Let us understand both of these two methods in detail.
Method 1: Using os.mkdir() Method to Create a Directory in Python
OS module has an in-built method os mkdir() to create directories using Python in the system.
Syntax
Two arguments are passed to the method they are
- path: A path is a string or byte value that includes the complete path and the name of the directory to be created. path argument is basically the location where the user wants to create a directory.
- mode (optional): This is an optional argument that the user may pass. It contains the information about the permissions that need to be given to deal with the file operations within the directory. The default value is 0o777 (octal).
Example 1: Create a Directory using Python in a specified location
Output
In the above example, we are passing the path string that has the location followed by name of the new directory that we need to create. The function doesn't have any return type and the code will continue without any error if the new directory is successfully created, otherwise program will throws an OSError.
Example 2: Providing Permissions for Reading and Writing Operations Within the Directory
Output
Here, setting mode = 0o666 allows the user to perform read and write file operations within the created directory.
Exceptions with os.mkdir() Method
The os.mkdir() method in OS module raises a FileExistsError if the directory in the location specified as path already exists. For example, after executing example 1, the directory will be created, and if we try to execute the same program again following error will be raised.
Output
Method 2: Using os.makedirs()
Another way to create a directory in Python is using the os.makedirs() method built-in in the OS module to create nested directories within the system. The os.makedirs() function creates a parent directory, intermediate directories, and leaf directories (leaf directories are those directories that occur at the end of a path) if they are not already present in the file system.
Syntax
The syntax of it is similar to os.mkdir() and two arguments of os.makedirs() is same as os.mkdir() function i.e., path and mode. Apart from these two arguments os.makedirs() also has a third optional argument that is
- exist_ok (optional): A default value of optional is False. If the target directory already exists in the file system, an OSError is raised if the value of this parameter is False; otherwise, no error will be raised by the function.
Example
Output
Here, the makedirs() function creates the OS_Module directory (leaf directory) as well as creates an intermediate directory, i.e. Lib.
Exceptions with os.makedirs() Method
Just like os.mkdir() method, os.mkdir() method also raises the same FileExistsError if the directory in the location specified as path already exists. This can be understood when we try to create the same directories that we created in the previous example.
Output
Handling Errors While Using os.mkdir() or os.makedirs() Methods
We know that if an inaccessible or invalid path, file name, or other arguments are passed to functions in OS module, OSError is raised by the program even if they have correct types but are not accepted by the underlying operating system. We can put the os functions inside the try block to prevent programs from abruptly terminating when OSErrors are raised by functions in OS module. Let us see this with examples.
Example 1: Handling Error While Using os.mkdir() Method
Output
Example 2: Handling Error While Using os.makedirs() Method
Output
Conclusion
- OS module comes under Python's standard utility modules that provide several functionalities to interact with the underlying operating systems and the files.
- If an inaccessible or invalid path, file name, or other arguments are passed to methods in OS module, OSError is raised by the program even if they have correct types but are not accepted by the underlying operating system.
- OS module provides two different methods for creating directories using Python, that are os.mkdir(), os.makedirs().
- Both the methods have common two arguments that are path and an optional argument mode, but os.makedirs() also has an optional third argument which is exist_ok.
- Path is a string or byte value which includes the complete path and the name of the directory to be created, and mode contains the information about the permissions that need to be given to deal with the file operations within the directory. The default value is 0o777.