What is Data Hiding in Python?
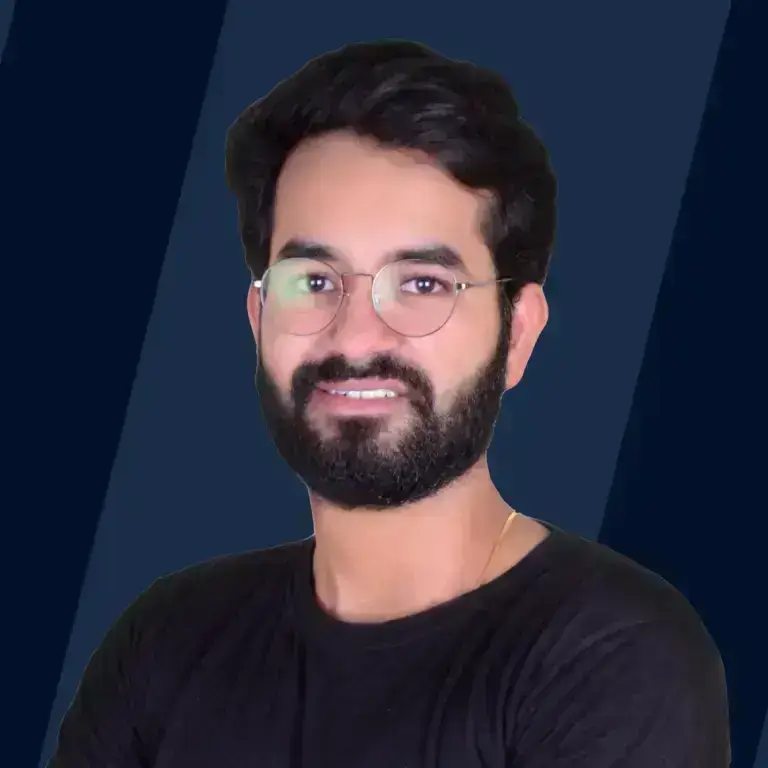
Data hiding is a method used in object-oriented programming (OOP) to hide the details of an object and function. Or in other words, data hiding means isolating the client from the implementation part of the application.
In Python, modules are enough to understand how to use a particular application correctly, but users can’t know how the application is working and what processes are running inside it.
Thus, data hiding is the most essential feature because it avoids dependency and provides security. Data hiding is also known as information hiding or encapsulation.
Syntax
In python, if we want to hide any variable, then we have to use the double underscore() before the variable name. This makes the class members inaccessible and private to other classes.
Public
When a member of a class is "public," it means it can be accessed by other objects. Or in other words, it can be accessed from any part of the program but only within a program. All the members and member functions are public by default.
Private
When members of the class are private, they can only be accessed by objects of the same class. It is not accessible outside of the class, not even any child class can access it. If you try to do it, the Python compiler will give an error message.
We use double underscores (__) before the data member to create a private data member inside the class.
Protected
Protected means the member of a class is only accessible by its inherited or child class. To create a protected data member inside the class, we use a single underscore (_) before the data member.
Examples of Data Hiding in Python
Let's take some examples to understand the topic data hiding in Python more clearly.
Example 1
In the below example, you can see that we are accessing the username and password from inside the class. In the method creden, as you can see that we have passed only Self. If you want to access the instance variables then we have to use the Self. Like here, username and password are the instance variables and we want to access their values.
You can read more about the self keyword from the given link: Self in Python
Output
Note: If we try to access a private member of a class from outside of a class, then it will throw an error.
Let's have a look at it.
Output
Example 2
To access private members of the class, we can use the following syntax: objectName._className.__attrName.
Output
Example 3
As you can see in the below code, the username is a private attribute while the password is a protected attribute.
Output
How Does Data Hiding Work in Python Classes?
As we have already discussed in the previous sections, we use a double underscore to make any attribute name private or inaccessible.
Let's see how we can hide any attribute so that it becomes inaccessible to outer classes.
Code
Output
In the above code, as you can see, we are trying to access the private attribute i.e. marks from the outside of the class using objects, that is why it is throwing an exception.
But, we can access the values of private attribute marks by using the following syntax given below:
Code
Output
So we can see that we are able to access the private members using the special syntax, but it's not easy as compared to the normal case.
Advantages and Disadvantages of Data Hiding
Advantages of Data Hiding
- By data hiding, the objects in the class are disconnected from the irrelevant data.
- It increases the security of the data so that hackers can not access it.
- Data hiding helps to prevent damage to volatile data by hiding it from the public.
Disadvantages of Data Hiding
- For the data hiding, the programmers have to write extra lines of code that become lengthy and unreadable.
- Data hiding prevents the linkage between visible and invisible data that makes the objects work faster.
- The objects work comparatively slower. That's why object-oriented programs are typically slower than procedure-based programs.
Learn More
Conclusion
Let’s conclude our topic, "What is data hiding in Python?" by looking into some of the important points.
- Data hiding is a method used in object-oriented programming (OOP) to hide the details of an object and function.
- Data hiding is the most essential feature because it avoids dependency and provides security.
- In python, if we want to hide any variable, then we have to use the double underscore() before the variable name.
- If we try to access a private member of a class from outside of a class, then it will throw an error.
- Data hiding helps to prevent damage to volatile data by hiding it from the public.
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.