Primality Test
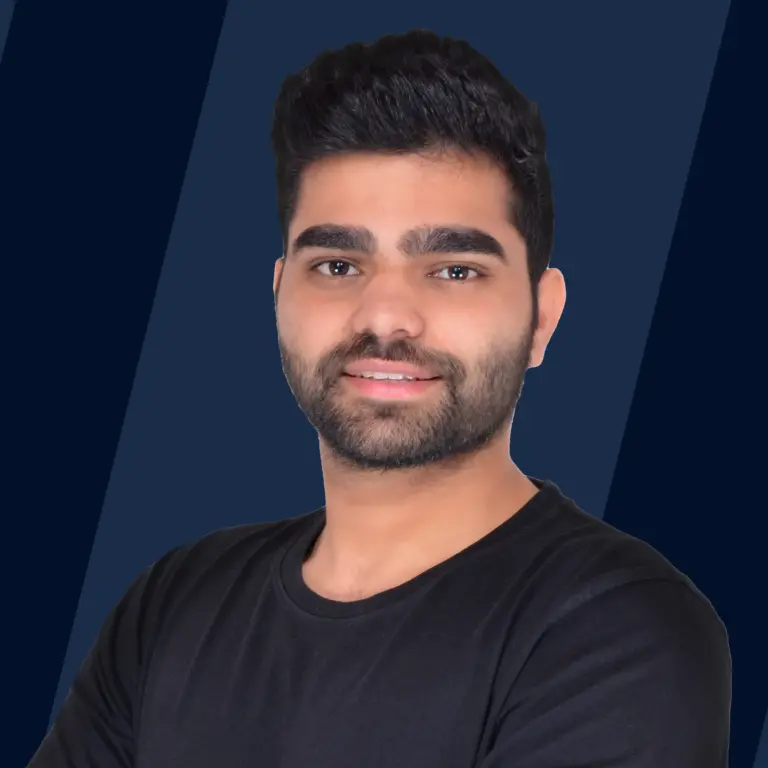
Overview
The Primality test is an algorithm for determining whether a given positive integer is a prime number or not.There are many methods to check whether a number is prime or not, like, the School Method, Trial Division Method, Fermat Primality test, and Miller-Rabin Primality test.
Takeaways
Algorithm for determining whether a given positive integer is a prime number or not :
- School method
- Trial Division Method
- Fermat Primality Test
- Miller-Rabin Primality Test
What is Primality Test?
The Primality test is an algorithm for determining whether a given positive integer is a prime number or not.
The primality of a number can also be checked by determining whether it is a composite number or not, because if the number is not a composite number nor 1, then it must be a prime number.
Example
Source Code - C++17
Source Code - Java (1.8)
Source Code - Python 3
The output is the same for all of the implementations as they peform the same thing, the only difference being the programming language.
Output
In the above examples, we are checking whether the given number is a prime number or not, to do so, we are iterating from to to check if these numbers divide the given number. If they do, then the given number is not a prime number, else it is a prime number.
School method
The school method is a simple solution that comes first to everyone's mind for primality check as it is the simplest of all.
In the School Method algorithm, we iterate through all the numbers from to (the number to be checked is ) and check if they divide the number .
Complexity Analysis
Time Complexity:
The time complexity of the School Method primality check is as we iterate times.
Space Complexity:
The space complexity here is constant.
The algorithm in the example above is School Method only.
Optimized School Method
In the School Method, we can do some optimizations like:
- Instead of checking till , we will check till because a factor of larger than must be a multiple of a number smaller than that has been already checked and should not be checked again.
- We will first check the divisibility of by and , then check its divisibility by numbers of the form and starting at . We do so because any integer greater than can be expressed in the form of , , , , , and (for ), but integers of the form , , , and will be divisible by or .
Let's have a look at its implementation in the section below.
Implementation of the Optimized School Method
Source Code - C++17
Source Code - Java (1.8)
Source Code - Python 3
The output is the same for all of the implementations as they peform the same thing, the only difference being the programming language.
Output
In the above examples, we are checking whether the given number is a prime number or not, to do so, we are first checking its divisibility by and , then checking its divisibility by numbers of the form and . If the given number is divisible by any number then it is not a prime number, else it is.
Complexity Analysis
Time complexity:
The asymptotic time complexity of the Optimized School Method primality check is because we iterate ( in the for loop) times in this algorithm.
Space Complexity:
The space complexity here is constant.
Trial Division Method
The Trial Division Method is a primality check algorithm in which we accept some integer , check if any number from 2 to divides the given integer, if a divisor is found then is a composite number, otherwise, it is a prime number. It is very similar to the school method.
Complexity Analysis
Time complexity:
The time complexity of The Trial Division primality check is as we iterate times.
Space Complexity:
The space complexity here is constant.
Fermat Primality Test
The Fermat Primality test is a probabilistic method to determine whether the given integer is a probable prime number or not.
It is based on Fermat's Little Theorem that states if is a prime number and is not divisible by , then , i.e (where is an integer constant).
If we want to test for a prime number, then we will pick some random integers that are not divisible by and see whether the congruency holds or not. If the congruency doesn't hold for a value of , then it will be a proof that is a composite number, but if the congruency holds for more than one values of , then is a probable prime number.
Algorithm Steps
- Repeat the following times (higher value of indicates higher probability of correct results):
- Handle corner cases for ( is the number to be tested).
- Pick a random number in the range .
- If , then return .
- Return , is probably a prime number.
Example
Let us assume, we have to check whether is a prime number or not. We will randomly pick a number in the range . Then we will check whether the congruency holds or not.
For ,
, as ,
Now there can be two cases, is a prime number, or is a Fermat liar. To proceed, let's take .
but, , as .
This proves that is a composite number and is Fermat liar, being the Fermat witness for the compositeness of .
Complexity Analysis
Time Complexity:
Here, is the number of iterations, and is the number to be tested for primality. is the time complexity for computing and the algorithm is repeated times. So the time complexity is .
Space Complexity:
There is no need for extra space, so the space complexity is constant.
The Fermat's little theorem is used for one more Primality test, called the Miller-Rabin Primality Test.
Miller-Rabin Primality Test
The Miller-Rabin Primality Test is also a probabilistic method to determine whether the given integer is a prime number or not, similar to the Fermat Primality Test. It is also based on the Fermat's Little Theorem (discussed in the above section).
This test was discovered by Gary Lee Miller in 1976, later modified by Michael Oser Rabin in 1980.
The result of this test will be whether the given number is a composite number or a probable prime number. The probable prime numbers in the Miller-Rabin Primality Test are called strong probable numbers, as they have a higher chance of being a prime number than in the Fermat's Primality Test.
A number is said to be strong probable prime number to base if one of these congruence relations (equivalence relations in modulo arithmetics, means is congruent to modulo ) holds:
Algorithm Steps
is the number to be tested.
indicates the accuracy of the test.
is an odd number such that can be written in the form of (Since is odd, must be even and ).
- Handle base cases for .
- if is even, return .
- Find an odd number .
- Loop the following times:
- Check if , if yes, return .
- Return .
- Pick a random number in the range .
- Compute KaTeX parse error: Expected 'EOF', got '%' at position 15: x = pow(a, d) %̲ n.
- Return if .
- Loop the following till .
- .
- Return if .
- Return if .
Implementation of the Miller-Rabin Primality Test
Source Code - C++17
Source Code - Java (1.8)
Source Code - Python 3
The output is the same for all of the implementations as they peform the same thing, the only difference being the programming language.
Output
In the above examples, we are checking whether the given number is a probable prime number or a composite number, to do so, we are using the Miller-Rabin Primality Test. If the function returns , then the given number is a probable prime number, otherwise, it is a composite number.
The Miller-Rabin Test, being an extension of the Fermat's Primality Test, is more accurate than Fermat's Primality Test, thus, it is preferred over that.
Complexity Analysis
Time Complexity:
The time complexity of the Miller-Rabin Primality Test is , where is the number to be checked for primality, and is the number of iterations performed for accuracy as per the requirement.
Space Complexity:
There is no need for extra space, so the space complexity is constant.
Conclusion
- Primality tests are used to determine whether a given number is a prime or not.
- The School method is a naive algorithm of time complexity.
- The Optimized School method and the Trial Division algorithm have a time complexity of .
- The Fermat's Primality test and Miller-Rabin Primality test return whether a number is composite or a probable prime, and are based on Fermat's Little Theorem.
- The time complexity of the Fermat's Primality Theorem is .
- The time complexity of the Miller-Rabin Primality Test is .