C Program to Convert Decimal to Binary
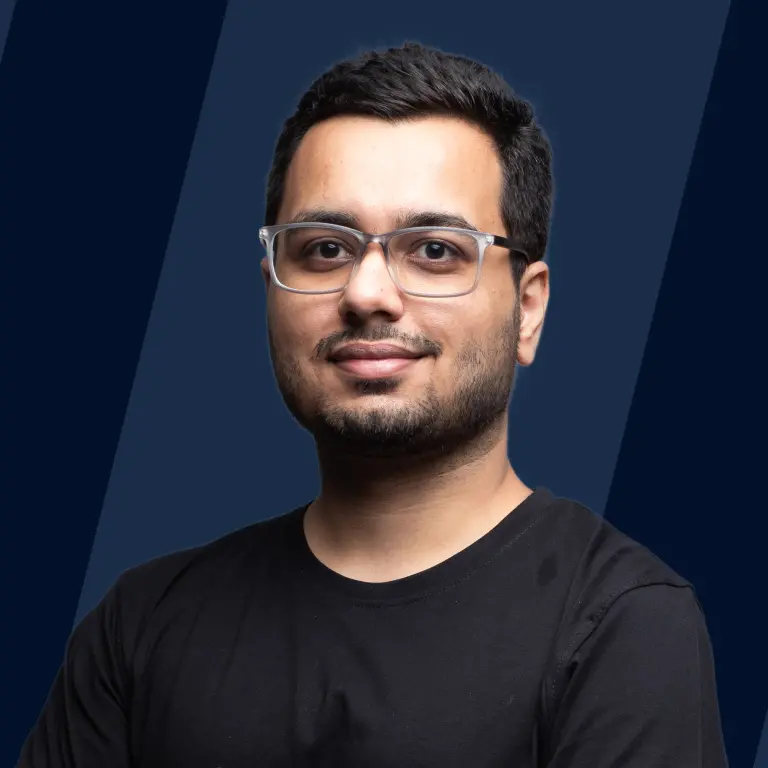
Given a decimal number (a number that consists of numbers between 0 - 9), we can convert it into a binary number (consisting of 0's and 1's) using loops, stacks, or bitwise operators.
The article describes the following approaches to convert decimal numbers to binary numbers:
- Using for-loop
- Using while loop
- Using Stack
- Using Right Shift (>>) and And(&) Operator
- Using Bitwise Operators
- Without Using Arrays
What is a Decimal Number?
A decimal number is a number represented in the decimal number system.
This system uses base 10 to represent numbers i.e. every digit in a decimal number is one of the following numbers: 0,1,2,3,4,5,6,7,8,9.
Ex: 1123, 32, 451, etc.
What is a Binary Number?
Binary number is a number represented in the binary number system.
This system uses base 2 to represent numbers i.e. every digit in a binary number is one of the following numbers: 0,1.
Ex: 101, 111, 100, etc.
Algorithm for Decimal to Binary Conversion in C
Step 1: Divide the number by 2 and find the remainder, then store the remainder in an array.
Step 2: Divide the number by 2.
Step 3: Repeat the above two steps until the number is greater than zero.
Step 4: Print the array in reverse order to get the binary representation of the number.
C Program to Convert Decimal to Binary Using For Loop
Output:
Time Complexity: O(log n) because we keep on dividing the number by 2 in every step which overall takes log n steps.
Space Complexity: O(log n) because there are log n + 1 digits in the binary representation of a number.
C Program to Convert Decimal to Binary Using While Loop
Output:
Time Complexity: O(log n) because we keep on dividing the number by 2 in every step which overall takes log n steps.
Space Complexity: O(log n) because there are log n + 1 digits in the binary representation of a number.
C Program to Convert Decimal to Binary Using Stack
Output:
Time Complexity : O(log n) because we keep on dividing the number by 2 in every step which overall takes log n steps.
Space Complexity : O(log n) because there are log n + 1 digits in the binary representation of a number.
C Program to Convert Decimal to Binary Using Right Shift (>>) and And(&) Operator
Output:
C Program to Convert Decimal to Binary Using Bitwise Operators
Idea here in this algorithm is to find binary representation of the number by checking every bit of the number. Since we know that any number is stored as binary in the computer system, so by checking every bit we will be able to find the required binary representation.
Output:
Time Complexity: O(log n) because there are log n + 1 digits in the binary representation of the number.
Space Complexity: O(1) because we are not using any extra space.
C Program to Convert Decimal to Binary without Using Arrays
The idea here in this algorithm is not to use any extra space to store the binary representation of the number. We do so by storing the binary representation as a decimal number. But here, we have to take care of long long integer overflow so we can perform this till the range of long long int.
Output :
Time Complexity: O(log n) because we keep on dividing the number by 2 in every step which overall takes log n steps.
Space Complexity: O(1) because we are not using any extra space.
Conclusion
So in this article, we learned
- Some things about number systems particularly their types.
- Different approaches to convert a number from decimal number system to binary number system.
- Time complexity of converting a decimal number to binary is O(log n) where n is the number.
- Space Complexity is O(log n) if we use array/stack. O(1) if we do not use array/stack.
To learn about how to convert from a binary number to decimal, visit this article.