C Program to Convert Binary Number to Decimal
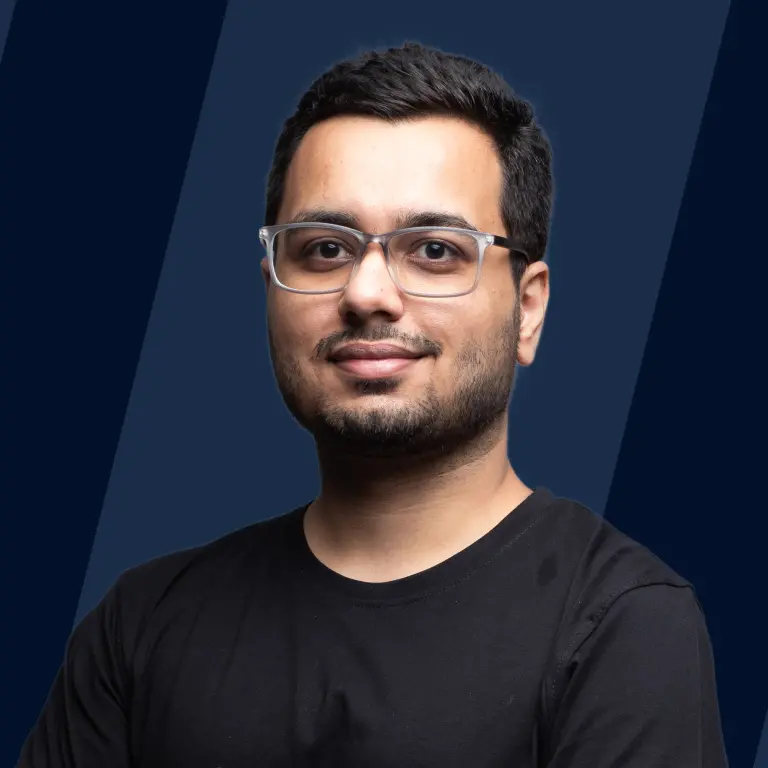
Introduction
We convert binary numbers to decimal numbers to make it easier to interpret huge binary values in a human-readable format. Binary numbers are the base - number system numerals, which are generally represented by just two digits/symbols: (Zero) and (One), while decimal numbers are the base - number system numbers, which are represented by numbers formed by digits in the range of .
The computer, as we all know, can not interpret the characters and numbers that humans type or perform. It can only understand binary numbers, i.e., and instead. When we input a decimal number into the computer, various software or compilers assist us in converting these decimal numbers into their binary form ( and ) for a computer machine to interpret the human language. Similarly, when a computer shows us some results, it internally converts the binary values into decimal values using various available software on the computer. We will first see what binary numbers and decimal numbers are; then we will move on to see how to convert binary to decimal in C.
Binary Numbers
and are used for binary number representation, and these are used to encode the computer data.
For example:
in the binary system = in the decimal system,
in the binary system = in the decimal system, etc.
Let's look at the below image to understand the binary number representation :
Decimal Numbers
Decimal numbers are used for human computations that are formed using integers in the range of . For example, , , , , etc.
Algorithm to Convert Binary Numbers to Decimal
- Take an input binary number from the user.
- Store the binary number in a temporary variable to preserve the value of the binary number in the program.
- Make a loop (repeat the below steps) until the temporary variable is greater than .
- Divide the temporary variable (binary value) by and take a single binary digit (remainder) from the right side of the binary value and store it in a remainder variable.
- Simultaneously multiply the remainder values from their place values according to the base number system, i.e. sequential powers of (beginning from ).
- Take the sum of these resultant values at each step and store the value in a decimal number equivalent variable.
- Reduce temp at each step by a factor of (temp /= 10) and increase the base by a factor of (base *= 2).
- When the loop ends, the decimal number variable contains a binary number equivalent value.
Example:
To create the decimal equivalent of the binary number , we took the binary number and multiplied each digit from right to left by sequential powers of .
Convert Binary Number to Decimal Using while Loop
We are applying the above conversion algorithm using the while loop to convert binary to decimal in C :
C Program:
Input:
Output:
Explanation:
- First, we have taken an input binary number from the user using the scanf() function.
- We have stored the input binary number (111) in a temporary variable to preserve the value of the binary number for further usage.
- We have applied the conversion algorithm using the while loop in the above C Program. Further, we have stored the converted binary number in a decimalNumber variable.
- Lastly, we have printed the decimal equivalent number (7) in the output.
Convert Binary Number to Decimal Using For Loop
We are applying the conversion algorithm using the for loop to convert binary to decimal in C :
C Program:
Input:
Output:
Explanation:
- We have taken an input binary number (1110) from the user using the scanf() function.
- We have applied the conversion algorithm using the for a loop this time in the above C Program. Further, we have stored the converted binary number in a decimalNumber variable.
- Lastly, we have printed the decimal equivalent number (14) in the output.
Convert Binary Number to Decimal Using Function
We are applying the conversion algorithm using a user-defined function and a while loop to convert binary to decimal in C :
C Program:
Input:
Output:
Explanation:
- We have taken an input binary number (110) from the user in the main() method using the scanf() function.
- We have applied the conversion algorithm in a user-defined function this time and used the while loop to implement the algorithm inside the function.
- Further, we have stored the converted binary number in a decimalRep variable and returned the value to the main() function.
- Lastly, we have printed the decimal equivalent number (6) in the output.
Conclusion
- To convert Binary to Decimal in C, extract the last digit from the binary number at each step by dividing it by until the binary number becomes , and simultaneously multiply the extracted digit to the sequential powers of , and add all the product values to get an equivalent decimal number.
- We have to convert binary to decimal in C to make it easier for us to interpret large binary values.
- The computer can only deal with binary numbers, so to understand the computer data we also need to convert the binary value to decimal values (human-understandable).
- and are used for binary number representation, while decimal numbers can be represented by integer numbers formed by the digits in the range of .